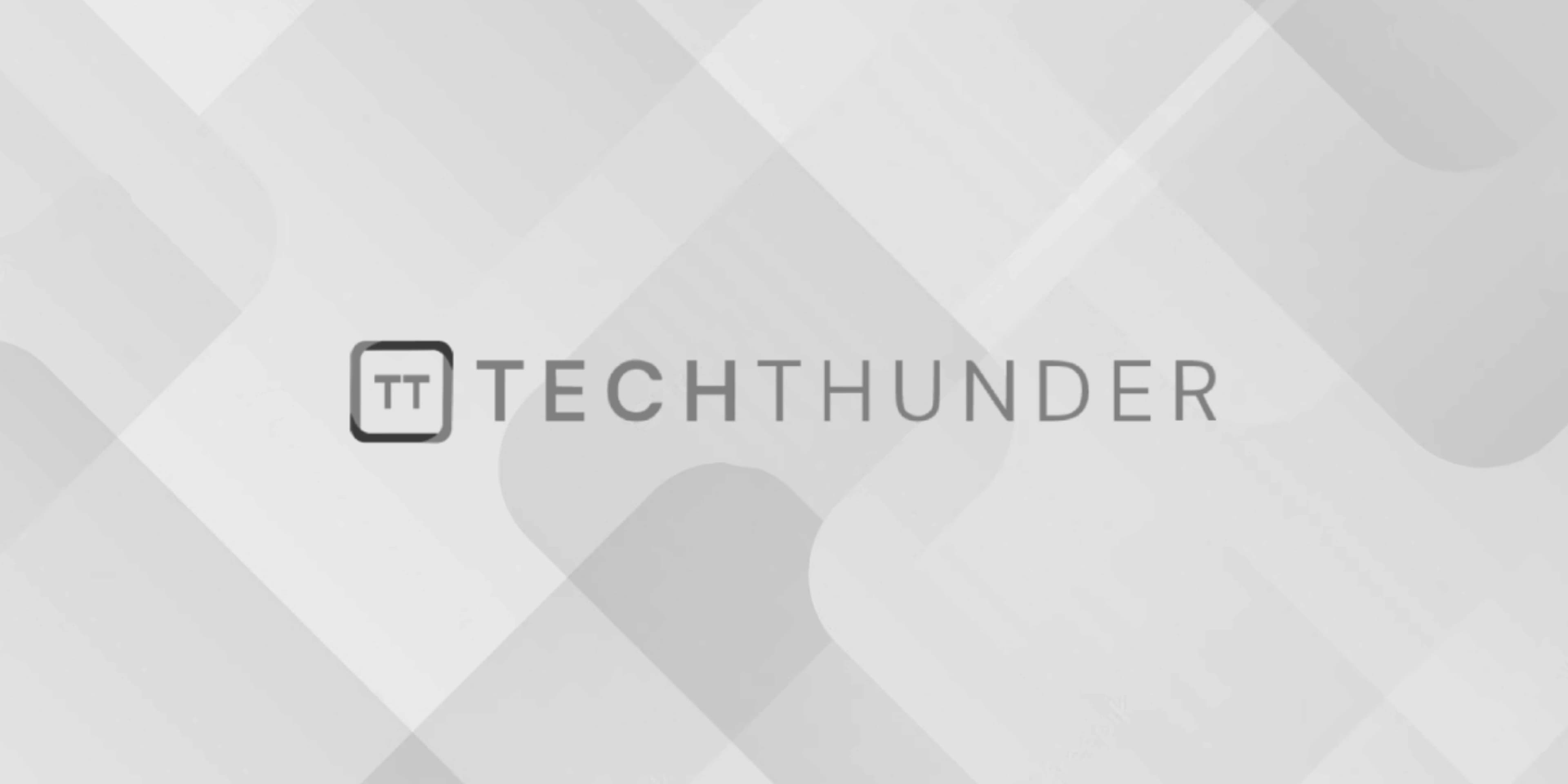
How to display digital clock using HTML, CSS, and JavaScript
To display a digital clock using HTML, CSS, and JavaScript, follow these steps:
Step 1: Create the HTML structure:
<!DOCTYPE html>
<html>
<head>
<title>Digital Clock</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="clock">
<span id="hours">00</span>:
<span id="minutes">00</span>:
<span id="seconds">00</span>
</div>
<script src="script.js"></script>
</body>
</html>
Step 2: Create the CSS (styles.css):
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f2f2f2;
}
.clock {
font-size: 2rem;
font-weight: bold;
}
Step 3: Implement the JavaScript (script.js):
function updateClock() {
const now = new Date();
const hours = formatTime(now.getHours());
const minutes = formatTime(now.getMinutes());
const seconds = formatTime(now.getSeconds());
document.getElementById('hours').textContent = hours;
document.getElementById('minutes').textContent = minutes;
document.getElementById('seconds').textContent = seconds;
}
function formatTime(time) {
return time < 10 ? `0${time}` : time;
}
// Update the clock every second
setInterval(updateClock, 1000);
// Initial update
updateClock();
In this example, we create a simple HTML structure with three spans (hours, minutes, and seconds) to display the time. We apply some basic styling using CSS to center the clock on the page.
In the JavaScript code, we define two functions:
updateClock()
: This function gets the current time (hours, minutes, and seconds) and updates the corresponding spans in the HTML.formatTime()
: This function formats the time to ensure that it always displays two digits (e.g., 01, 02, …, 09).
We use setInterval(updateClock, 1000)
to call the updateClock()
function every second, which continuously updates the time on the clock.
When you open the HTML file in your browser, you should see a digital clock displaying the current time in real-time.
Feel free to customize the styles and layout to match your preferences. This is a basic example, and you can enhance it with additional features or animations to make the clock more visually appealing.