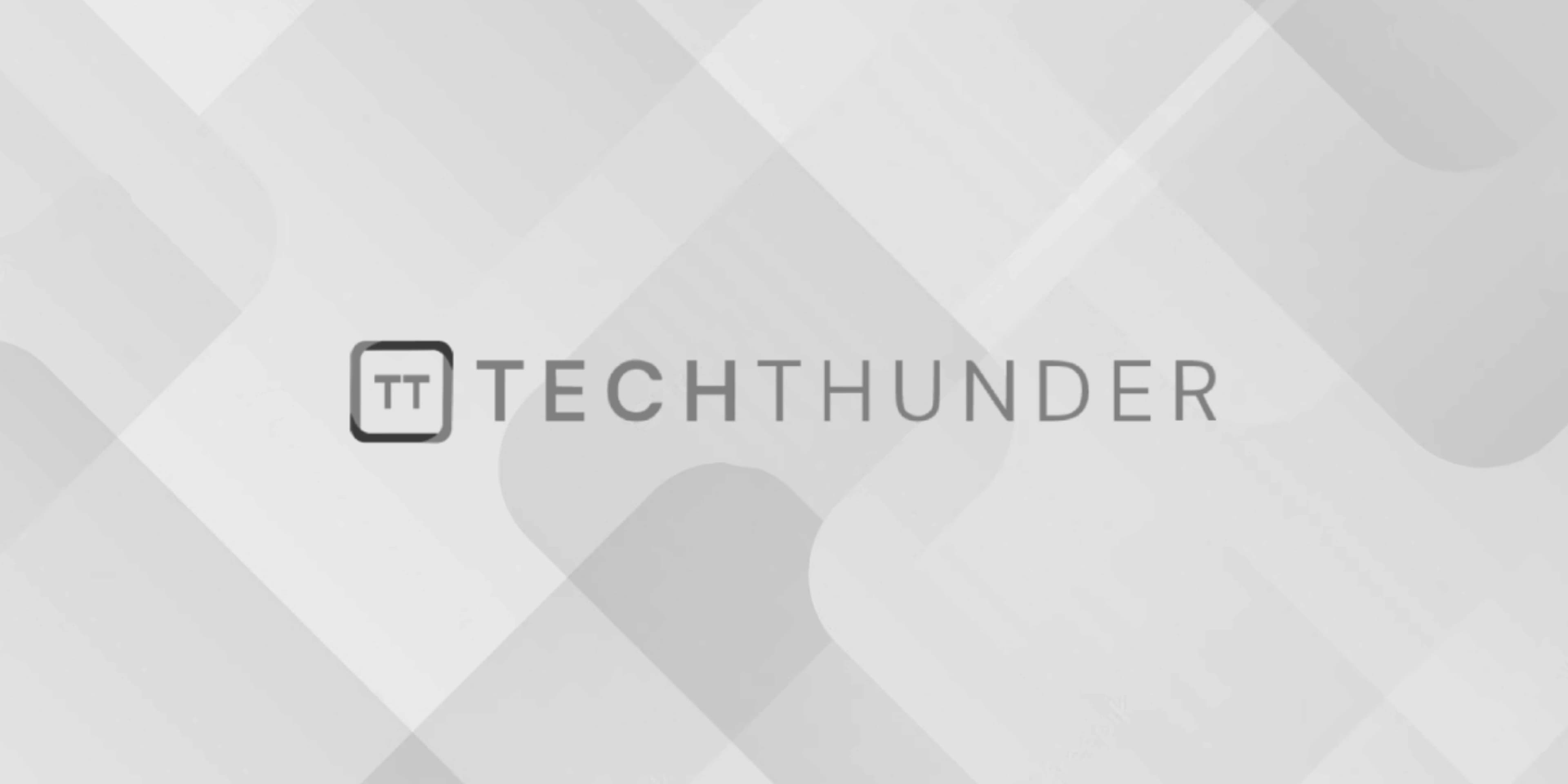
jQuery toArray() method
The jQuery.toArray()
method is used to convert a jQuery object into a regular JavaScript array. It allows you to extract the elements of a jQuery object and store them in a standard array, making it easier to perform array-specific operations on the elements.
Here’s the basic syntax of the toArray()
method:
$(selector).toArray()
Parameters:
selector
: The selector expression used to select the elements.
Return Value:
The toArray()
method returns a regular JavaScript array containing the elements of the jQuery object.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery toArray() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div class="item">Item 1</div>
<div class="item">Item 2</div>
<div class="item">Item 3</div>
<script>
$(document).ready(function() {
// Select all elements with class "item" and convert them to an array
var itemArray = $(".item").toArray();
// Log the number of elements in the array and their content
console.log("Number of elements in the array:", itemArray.length);
itemArray.forEach(function(element, index) {
console.log("Element " + index + ":", element.innerHTML);
});
});
</script>
</body>
</html>
In this example, we use the toArray()
method to convert the jQuery object containing elements with the class “item” into a regular JavaScript array. We then log the number of elements in the array and their content using console.log()
.
The output of the above example will be:
Number of elements in the array: 3
Element 0: Item 1
Element 1: Item 2
Element 2: Item 3
In this output, we see that the elements of the jQuery object are successfully converted into an array, and we can access the array elements using standard array methods like forEach()
.
By converting a jQuery object into an array, you can take advantage of the vast array manipulation methods available in JavaScript and easily perform operations on the selected elements. It is especially helpful when you need to work with the elements as a collection and perform actions that are more convenient with arrays.