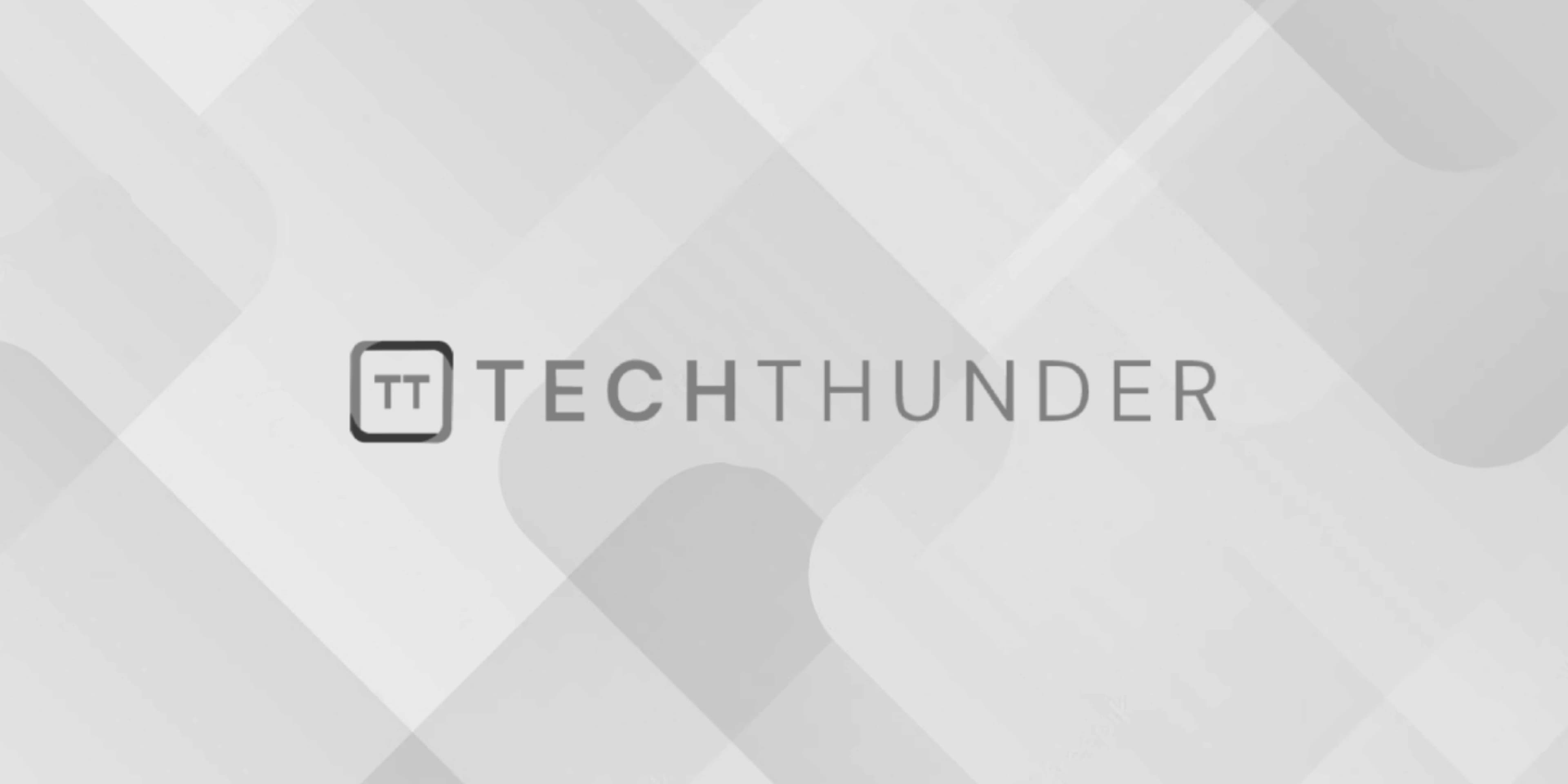
jQuery prev() method
The jQuery.prev()
method is used to select the immediately preceding sibling of each element in the jQuery object. It allows you to target the sibling element that appears just before the selected element in the DOM hierarchy.
Here’s the basic syntax of the prev()
method:
$(selector).prev([filter])
Parameters:
selector
: Optional. A selector expression used to filter the preceding sibling elements. If provided, only the preceding siblings that match the selector will be selected.filter
: Optional. A selector expression used to further filter the selected preceding siblings.
Return Value:
The prev()
method returns a jQuery object containing the selected preceding sibling elements.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery prev() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div>Div 1</div>
<p>Paragraph 1</p>
<span>Span 1</span>
<div>Div 2</div>
<script>
$(document).ready(function() {
// Select the immediately preceding sibling of the <span> element
var precedingSibling = $("span").prev();
// Log the selected preceding sibling element
console.log(precedingSibling);
});
</script>
</body>
</html>
In this example, we use the prev()
method to select the immediately preceding sibling of the <span>
element. The method returns a jQuery object containing the <p>
element, which is the sibling that appears just before the <span>
element in the DOM hierarchy.
The output of the above example will be:
[<p>Paragraph 1</p>]
In this output, we see that the prev()
method successfully selected the preceding sibling element of the <span>
element.
If you provide a selector
or filter
argument to the prev()
method, it will only select the preceding siblings that match the specified selector or filter. For example:
var filteredPrecedingSiblings = $("span").prev("div");
This will select only the preceding sibling with the <div>
element type and exclude any other preceding siblings that don’t match the selector.
The prev()
method is useful when you want to target the sibling element that comes immediately before a particular element in the DOM hierarchy. It provides a convenient way to traverse the DOM tree and select elements based on their position relative to other elements.