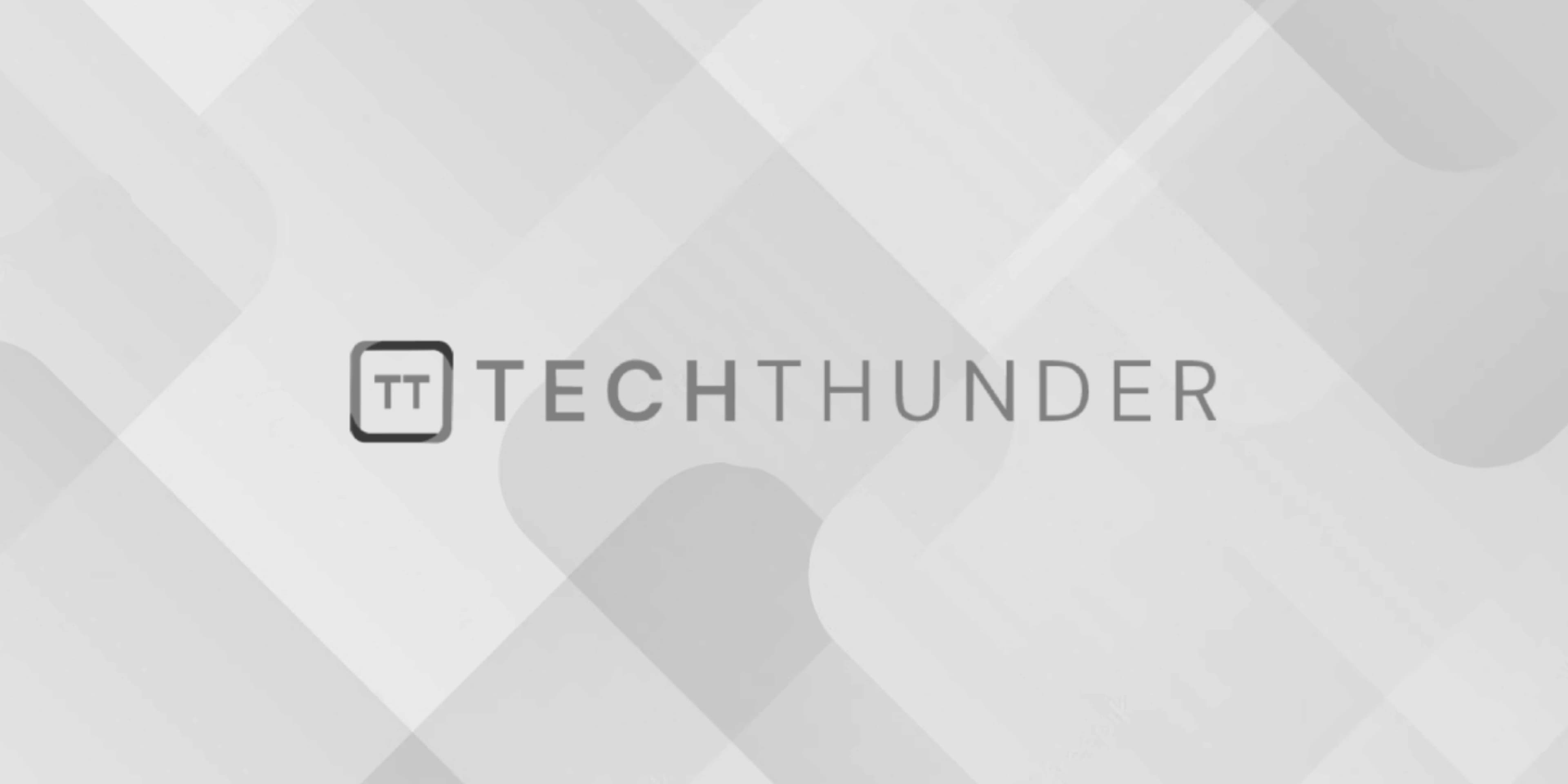
jQuery post() method
The jQuery post()
method is used to send an HTTP POST request to a specified URL and receive a response from the server. It is a shorthand method for making AJAX (Asynchronous JavaScript and XML) POST requests.
Here’s the basic syntax of the post()
method:
$.post(url, data, successCallback, dataType)
Parameters:
url
: The URL to which the POST request will be sent.data
: (Optional) The data to be sent to the server with the POST request. It can be in the form of an object, a string, or a serialized form data.successCallback
: (Optional) A function to be executed if the request is successful. The response from the server is passed to this function as a parameter.dataType
: (Optional) The type of data expected from the server. It can be “xml”, “json”, “html”, “text”, etc. By default, it is intelligent guessing based on the MIME type of the response.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery post() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div id="result"></div>
<script>
$(document).ready(function() {
// Data to be sent to the server
var postData = {
name: "John",
age: 30
};
// Send a POST request to the server
$.post("https://example.com/api", postData, function(response) {
// Process the server response
$("#result").text("Response from server: " + response);
}, "text");
});
</script>
</body>
</html>
In this example, we use the post()
method to send a POST request to the server with the postData
object as the data. The server is expected to respond with plain text data, and the response is displayed inside the result
div on the page.
The post()
method is a convenient way to perform AJAX POST requests and is commonly used to submit data to a server without refreshing the entire web page. It is often used in conjunction with server-side scripts or APIs to handle the incoming data and return a response to the client-side JavaScript.