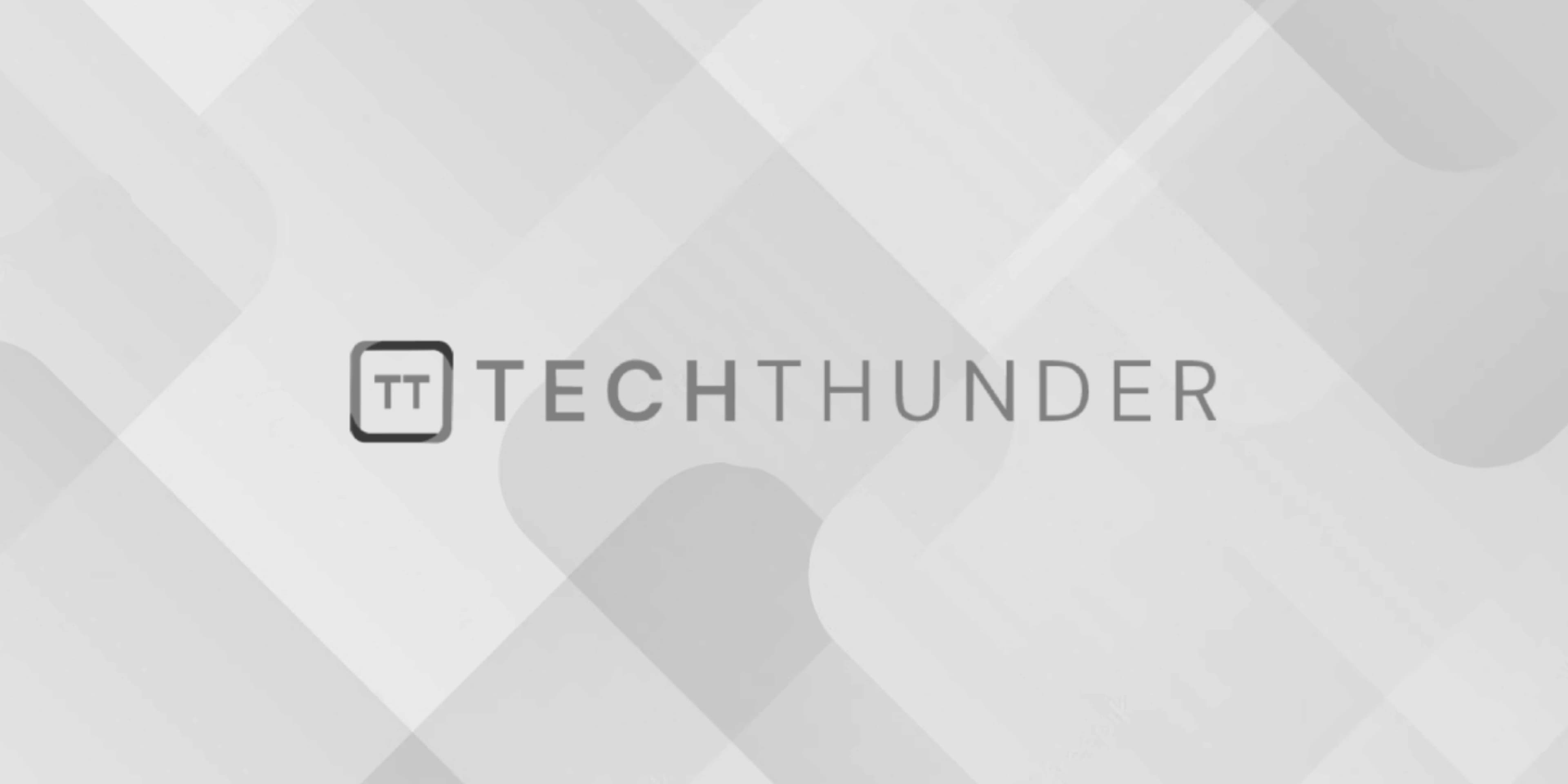
How to create an online radio using jQuery and jPlayer
Creating an online radio using jQuery and jPlayer involves setting up the jPlayer library and utilizing its audio player features to stream and play audio content. Below is a step-by-step guide on how to create a basic online radio using jQuery and jPlayer:
Step 1: Set Up HTML Structure
<!DOCTYPE html>
<html>
<head>
<title>Online Radio</title>
<!-- Include jQuery and jPlayer libraries -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jplayer/2.9.2/jplayer/jquery.jplayer.min.js"></script>
</head>
<body>
<h1>Online Radio</h1>
<div id="jquery_jplayer"></div>
<div id="jp_container_1" class="jp-audio" role="application" aria-label="media player">
<div class="jp-type-single">
<div class="jp-gui jp-interface">
<ul class="jp-controls">
<li><a href="javascript:void(0);" class="jp-play" tabindex="1">play</a></li>
<li><a href="javascript:void(0);" class="jp-pause" tabindex="1">pause</a></li>
</ul>
<div class="jp-progress">
<div class="jp-seek-bar">
<div class="jp-play-bar"></div>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
Step 2: Set Up CSS Styling (Optional)
You can add some basic CSS to style the player controls and progress bar. Customize the styling according to your design requirements.
Step 3: Initialize jPlayer and Set Up Audio Stream
$(document).ready(function() {
$("#jquery_jplayer").jPlayer({
ready: function () {
$(this).jPlayer("setMedia", {
mp3: "your-audio-stream-url.mp3"
});
},
swfPath: "https://cdnjs.cloudflare.com/ajax/libs/jplayer/2.9.2/jplayer",
supplied: "mp3",
preload: "none",
wmode: "window",
useStateClassSkin: true,
autoBlur: false,
smoothPlayBar: true,
keyEnabled: true,
remainingDuration: true,
toggleDuration: true
});
});
Replace "your-audio-stream-url.mp3"
with the URL of the audio stream you want to play. Ensure that the audio stream URL points to a valid audio file or an online audio stream.
Step 4: Customize Controls (Optional)
You can customize the player controls further by adding more options and features provided by jPlayer. For example, you can add volume controls, a playlist, or custom event handlers for play and pause actions.
Remember that this is a basic example to get you started with jPlayer for creating an online radio. Depending on your specific requirements, you may need to implement additional features, handle multiple audio streams, and perform server-side operations to manage the online radio station effectively. Additionally, ensure that you comply with any legal requirements for streaming audio content and consider using a reliable streaming service to host your online radio.