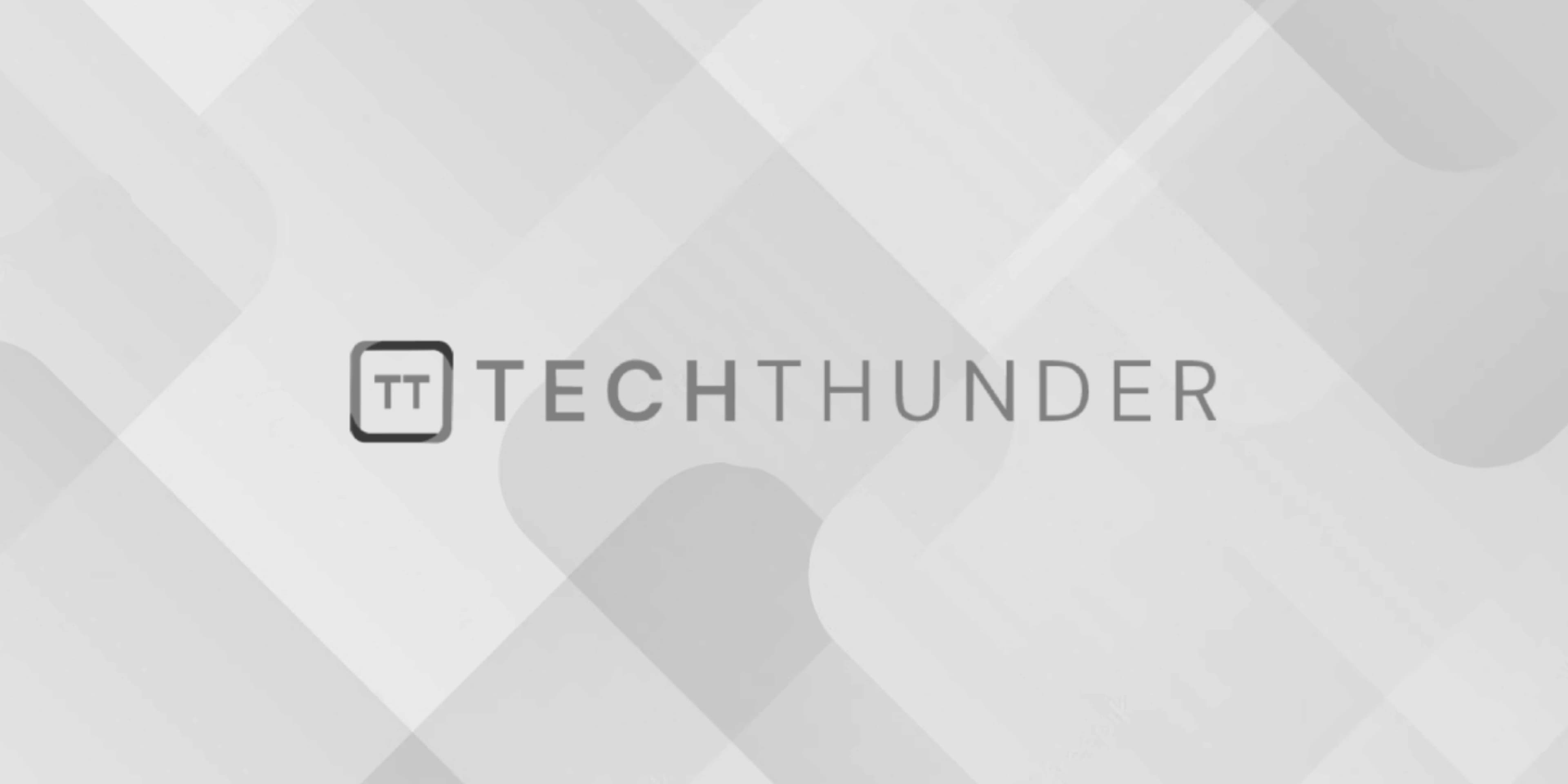
Vertical Dot Navigation Plugin
The concept of a vertical dot navigation typically involves a vertical list of dots that represent different sections or steps of a page. When the user scrolls down the page, the corresponding dot representing the current section gets highlighted or activated. However, you can create a vertical dot navigation menu using jQuery and CSS, or you may find custom plugins or libraries that offer similar functionality under different names.
Below is an example of how you can create a simple vertical dot navigation using HTML, CSS, and jQuery:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Vertical Dot Navigation</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="vertical-dot-navigation">
<ul>
<li class="dot" data-section="section1"></li>
<li class="dot" data-section="section2"></li>
<li class="dot" data-section="section3"></li>
<!-- Add more dots as needed -->
</ul>
</div>
<div class="section" id="section1">
<!-- Content for Section 1 -->
</div>
<div class="section" id="section2">
<!-- Content for Section 2 -->
</div>
<div class="section" id="section3">
<!-- Content for Section 3 -->
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
CSS (styles.css):
body {
margin: 0;
padding: 0;
}
.vertical-dot-navigation {
position: fixed;
top: 50%;
right: 20px;
transform: translateY(-50%);
z-index: 100;
}
.vertical-dot-navigation ul {
list-style: none;
padding: 0;
}
.vertical-dot-navigation .dot {
width: 10px;
height: 10px;
border-radius: 50%;
background-color: #ccc;
margin: 10px 0;
cursor: pointer;
}
.vertical-dot-navigation .dot.active {
background-color: #f00;
}
.section {
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
font-size: 24px;
}
JavaScript (script.js):
$(document).ready(function() {
const dots = $('.dot');
const sections = $('.section');
// Highlight the dot corresponding to the current section on scroll
$(window).on('scroll', function() {
const scrollTop = $(window).scrollTop();
const windowHeight = $(window).height();
sections.each(function() {
const offsetTop = $(this).offset().top;
const sectionHeight = $(this).height();
const sectionId = $(this).attr('id');
if (scrollTop >= offsetTop && scrollTop < offsetTop + sectionHeight - windowHeight) {
dots.removeClass('active');
dots.filter(`[data-section="${sectionId}"]`).addClass('active');
}
});
});
// Scroll to the corresponding section when a dot is clicked
dots.on('click', function() {
const sectionId = $(this).attr('data-section');
const targetSection = $(`#${sectionId}`);
const targetOffset = targetSection.offset().top;
$('html, body').animate({ scrollTop: targetOffset }, 500);
});
});
In this example, we create a vertical list of dots using HTML and CSS, representing different sections of the page. We use jQuery to handle scrolling and highlighting of the active dot based on the current section visible on the page. When a dot is clicked, it smoothly scrolls to the corresponding section.
You can customize the styles and add more sections and dots as needed to fit your specific use case. Additionally, you can extend this concept to create more advanced animations or transitions for the vertical dot navigation.