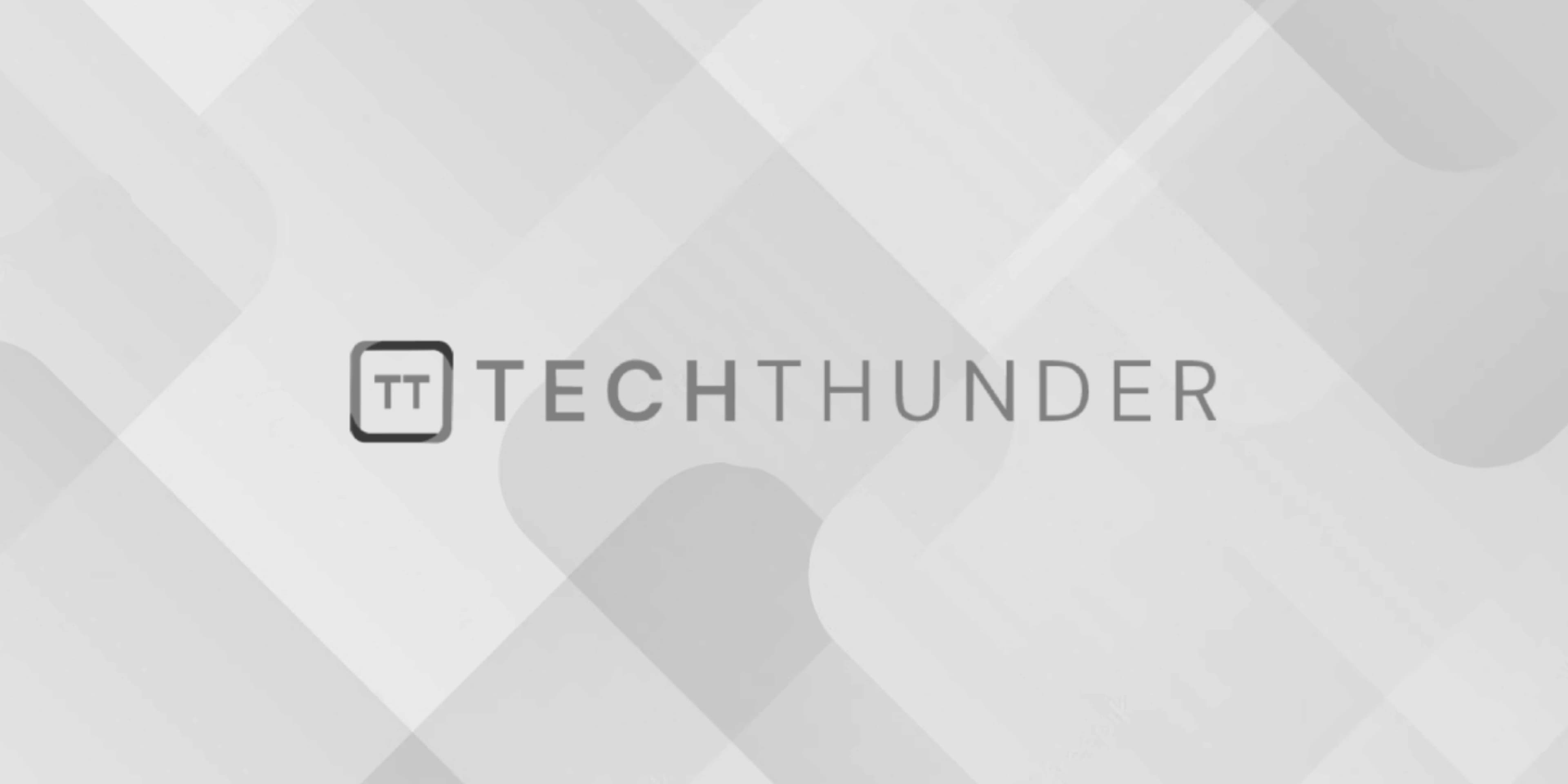
jQuery undelegate() method
The jQuery.undelegate()
method is a deprecated method in jQuery, and its use is discouraged. Instead, it is recommended to use the .off()
method for event delegation and event unbinding.
The .undelegate()
method was used to remove event handlers that were previously attached using the .delegate()
method. The .delegate()
method allowed you to attach event handlers to elements that match a selector, even if those elements were added to the DOM dynamically after the initial binding.
Here’s the basic syntax of the deprecated undelegate()
method:
$(selector).undelegate(selector, eventType, handler)
Parameters:
selector
: A selector expression to filter the elements to which the event handler was originally bound.eventType
: The type of event (e.g., “click”, “mouseover”, etc.).handler
: The event handler function to be removed.
Return Value:
The undelegate()
method has no explicit return value.
Example:
<!DOCTYPE html>
<html>
<head>
<title>Deprecated jQuery undelegate() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="dynamicButton">Click Me</button>
<script>
// Deprecated usage of undelegate (Strongly discouraged)
$(document).delegate("#dynamicButton", "click", function() {
console.log("Button clicked.");
});
// Later in the code, to remove the event handler
$(document).undelegate("#dynamicButton", "click");
</script>
</body>
</html>
In this example, we use the deprecated undelegate()
method to remove the event handler for the click event that was previously attached to the dynamically added button with the ID “dynamicButton.”
Instead of using undelegate()
, you should use the .off()
method to remove event handlers. The .off()
method provides a more consistent and flexible way to remove event handlers, including both direct and delegated event handlers.
Here’s an example of using .off()
to remove a delegated event handler:
// Recommended usage with .off()
$(document).on("click", "#dynamicButton", function() {
console.log("Button clicked.");
});
// Later in the code, to remove the event handler
$(document).off("click", "#dynamicButton");
In summary, use the .off()
method instead of .undelegate()
for event unbinding, and prefer event delegation with .on()
over .delegate()
for attaching event handlers to dynamically added elements.