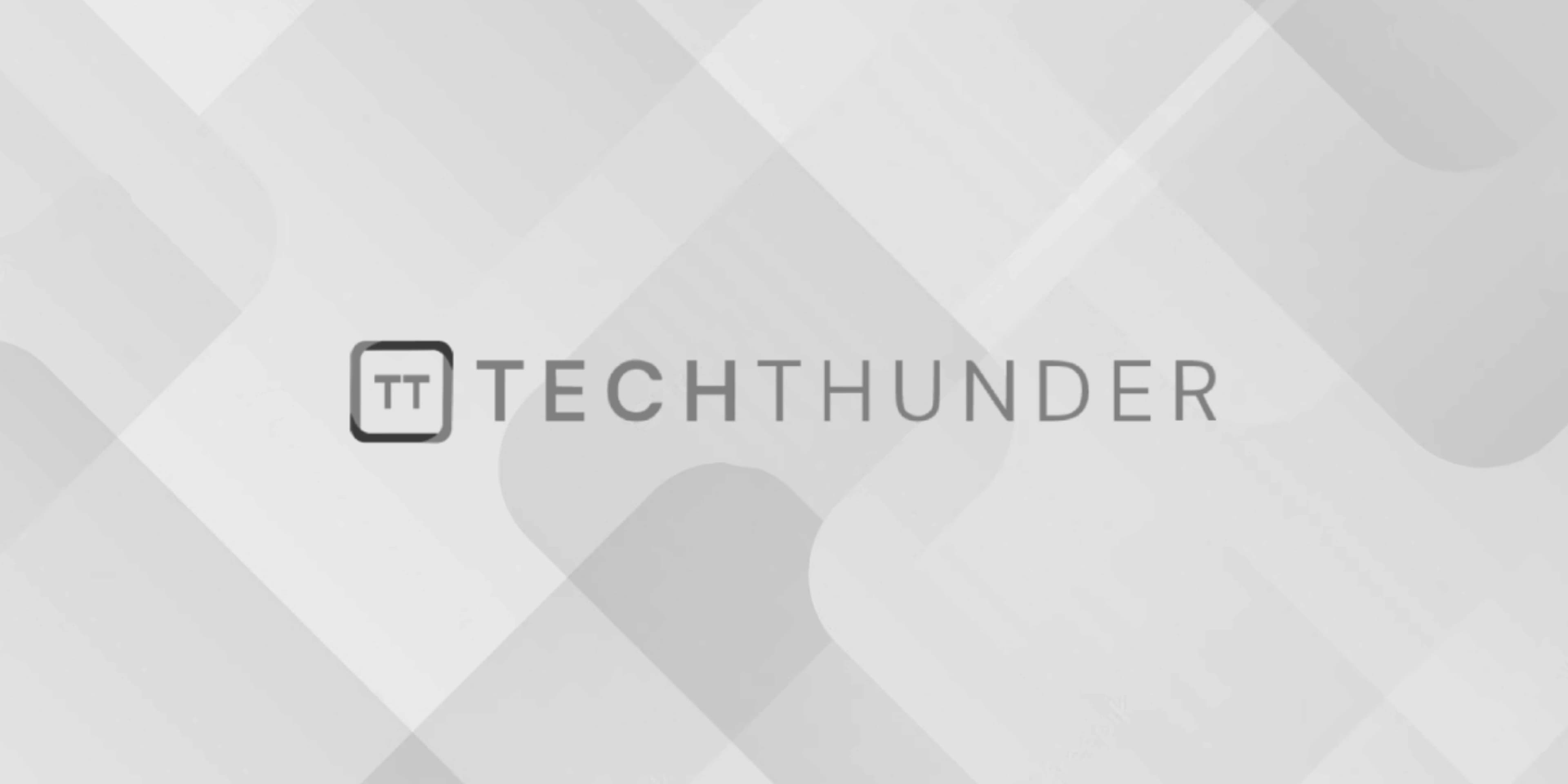
Checkbox validation in jQuery
Checkbox validation in jQuery involves ensuring that a checkbox is checked before proceeding with a form submission or any other action. To achieve this, you can use the prop()
method to check if the checkbox is checked or not. Here’s a simple example of how to perform checkbox validation using jQuery:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Checkbox Validation using jQuery</title>
</head>
<body>
<form id="myForm">
<label>
<input type="checkbox" name="agree" id="agreeCheckbox"> I agree to the terms and conditions
</label>
<br>
<button type="submit" id="submitButton">Submit</button>
</form>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
JavaScript (script.js):
$(document).ready(function() {
$('#myForm').submit(function(e) {
if (!$('#agreeCheckbox').prop('checked')) {
e.preventDefault();
alert('Please agree to the terms and conditions.');
}
});
});
In this example, we have a form with a single checkbox input for agreeing to the terms and conditions. When the form is submitted, the submit
event is triggered. We use the prop()
method to check if the checkbox with the ID #agreeCheckbox
is checked or not. If it’s not checked (prop('checked')
returns false
), we prevent the default form submission (e.preventDefault()
) and display an alert asking the user to agree to the terms and conditions.
By using this approach, the form will not be submitted unless the checkbox is checked, ensuring that the user agrees to the terms before proceeding.
You can modify the validation logic based on your specific requirements. For example, you might need to validate multiple checkboxes or add additional validation checks for other form fields. The general idea remains the same: use the prop()
method to check the checkbox status and handle the form submission accordingly.