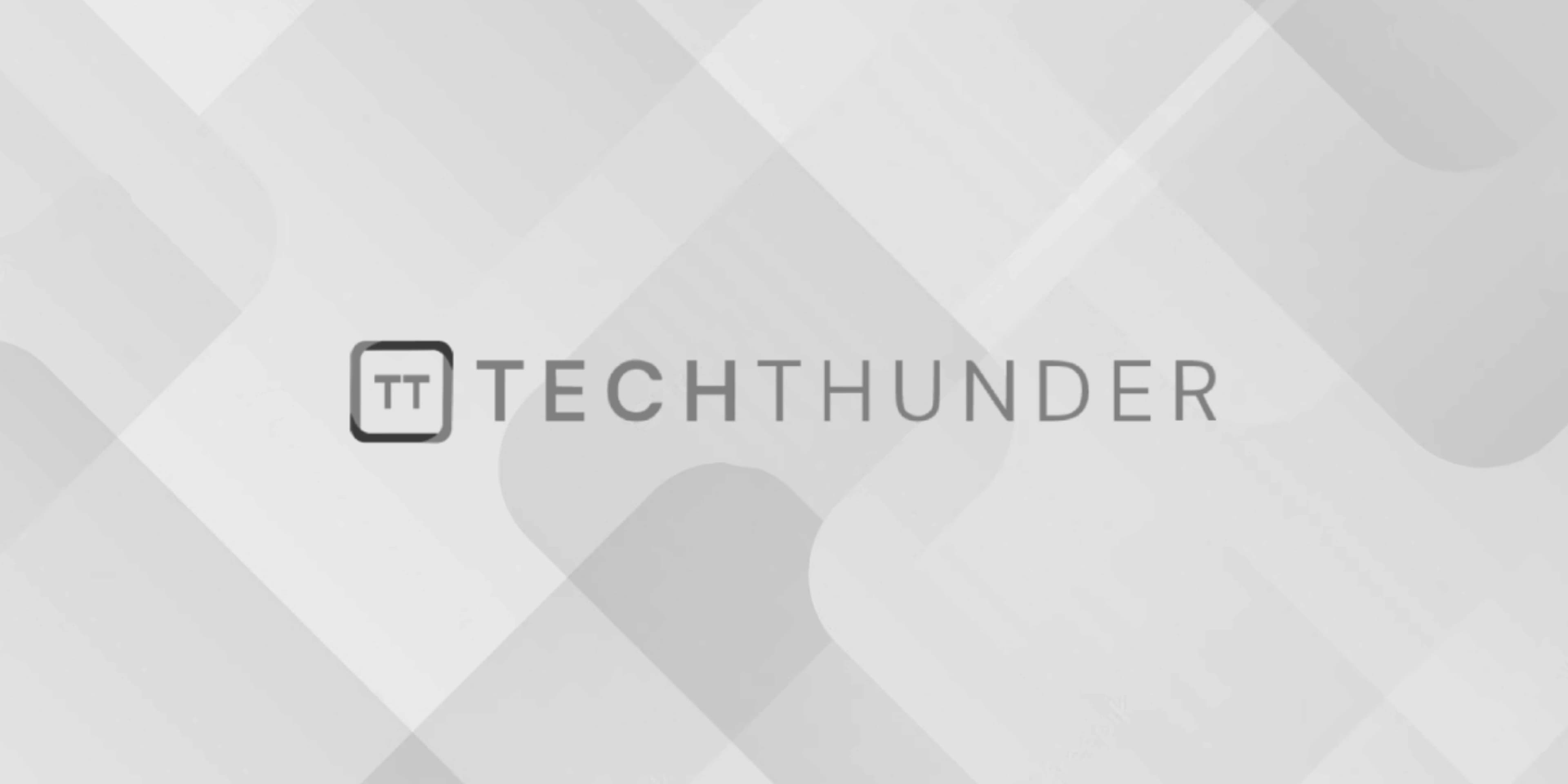
jQuery isNumeric() method
The jQuery isNumeric()
method is used to check whether a given value is a numeric value or can be converted to a valid numeric representation. It returns true
if the value is numeric and false
otherwise.
Here’s the basic syntax of the isNumeric()
method:
$.isNumeric(value)
Parameters:
value
: The value to be checked for numeric validity.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery isNumeric() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div id="output"></div>
<script>
$(document).ready(function() {
var value1 = "123";
var value2 = "3.14";
var value3 = "Hello";
var value4 = 42;
var value5 = "42px";
// Check if each value is numeric
$("#output").append("Value 1 is numeric: " + $.isNumeric(value1) + "<br>");
$("#output").append("Value 2 is numeric: " + $.isNumeric(value2) + "<br>");
$("#output").append("Value 3 is numeric: " + $.isNumeric(value3) + "<br>");
$("#output").append("Value 4 is numeric: " + $.isNumeric(value4) + "<br>");
$("#output").append("Value 5 is numeric: " + $.isNumeric(value5) + "<br>");
});
</script>
</body>
</html>
In this example, we have five different values: "123"
, "3.14"
, "Hello"
, 42
, and "42px"
. We use the isNumeric()
method to check if each value is numeric. The results will be displayed in the output
div. The method returns true
for values that are numeric or can be parsed as numbers ("123"
, "3.14"
, 42
) and false
for non-numeric values ("Hello"
, "42px"
).
The isNumeric()
method is helpful when you need to validate user input or when you want to ensure that a value can be used in numeric calculations without causing unexpected errors. It’s a quick and easy way to check the numeric validity of a value in jQuery.