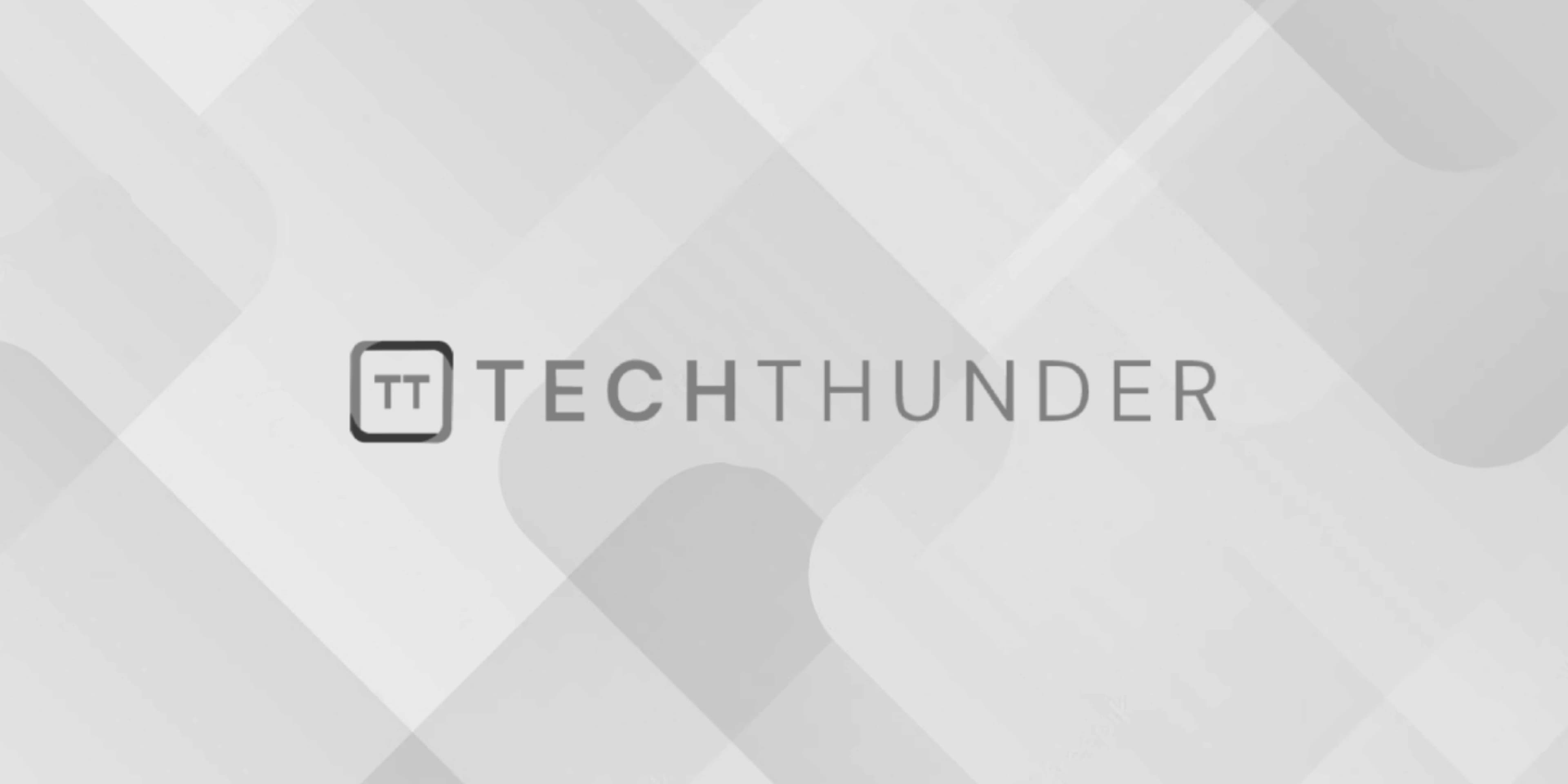
jQuery on() method
The jQuery on()
method is used to attach event handlers to elements, both existing and future elements that match the selector. It provides a way to handle events in a more efficient and flexible manner than using traditional event handlers like click()
, change()
, etc. The on()
method can be used to handle multiple events for one or more elements.
Here’s the basic syntax of the on()
method:
$(selector).on(event, handler)
Parameters:
selector
: A selector string to specify the elements to which the event handler will be attached.event
: A string representing the event type (e.g., “click”, “change”, “mouseenter”, etc.).handler
: The function to be executed when the event is triggered.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery on() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="myButton">Click Me</button>
<script>
$(document).ready(function() {
// Attach a click event handler to the button
$("#myButton").on("click", function() {
alert("Button clicked!");
});
});
</script>
</body>
</html>
In this example, we have a button with the ID “myButton”. We use the on()
method to attach a click event handler to the button. When the button is clicked, the function provided as the second argument to on()
will be executed, and an alert with the message “Button clicked!” will be shown.
The on()
method is especially useful when dealing with dynamically added elements or elements that may not exist at the time of binding the event handler. Since it works on current and future elements that match the selector, it provides a more efficient way to handle events in such scenarios.
Additionally, the on()
method allows you to handle multiple events for the same element with a single call, making your code more concise and easier to maintain. It also supports event delegation, which can improve performance for large document structures by attaching event handlers to a parent element and handling events for its descendants.