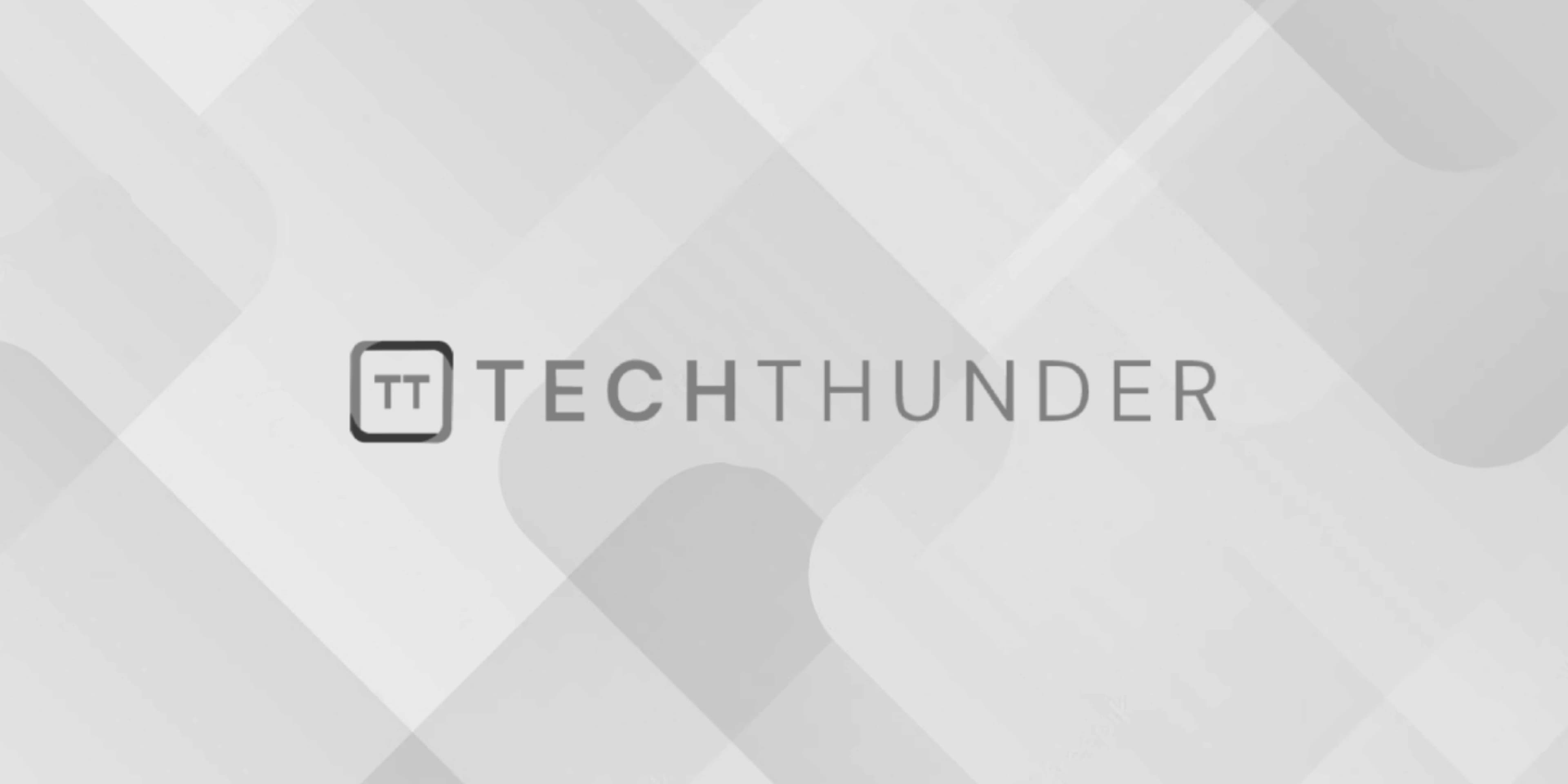
jQuery File manager for web
As of my last knowledge update in September 2021, there isn’t an official jQuery file manager specifically provided by jQuery itself. However, there are several third-party jQuery-based file manager plugins and libraries available that you can use to implement a file manager for the web. These plugins allow users to browse, upload, download, and manage files and directories within a web application.
One popular jQuery file manager plugin is “elFinder.” It provides a feature-rich file manager with a user-friendly interface and supports various file operations. Below is a step-by-step guide on how to set up a basic file manager using “elFinder”:
Step 1: Include Required Dependencies
First, include the required CSS and JavaScript files for “elFinder” in your HTML file:
<!DOCTYPE html>
<html>
<head>
<title>jQuery File Manager</title>
<!-- Include jQuery library -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<!-- Include elFinder CSS -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/elfinder/2.1.58/css/elfinder.min.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/elfinder/2.1.58/css/theme.min.css">
<!-- Include elFinder JS -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/elfinder/2.1.58/js/elfinder.min.js"></script>
</head>
<body>
<!-- File manager container -->
<div id="elfinder"></div>
<!-- Initialize elFinder -->
<script>
$(document).ready(function() {
$('#elfinder').elfinder();
});
</script>
</body>
</html>
Step 2: Set Up a Server-Side Connector (Required)
“elFinder” requires a server-side connector to handle file operations and provide the necessary backend functionalities. The connector acts as a bridge between the client-side JavaScript and the server-side file system. You can find various connectors for different server-side technologies on the “elFinder” website or GitHub repository.
For example, if you are using PHP, you can use the PHP connector:
- Download the PHP connector from the “elFinder” website: https://studio-42.github.io/elFinder/#download
- Extract the downloaded archive and place the “connector” folder on your server.
Step 3: Configure elFinder (Optional)
You can customize “elFinder” by passing configuration options when initializing it. For example, you can specify the connector URL and other options:
<script>
$(document).ready(function() {
$('#elfinder').elfinder({
url: 'path/to/connector/php/connector.php', // Replace with your server-side connector URL
height: 500, // Set the height of the file manager container
// Other configuration options go here
});
});
</script>
Ensure that you replace 'path/to/connector/php/connector.php'
with the correct URL to your server-side connector file.
Please note that the “elFinder” plugin is just one example of a jQuery file manager. There are other jQuery-based file manager plugins available with different features and capabilities. Depending on your specific requirements, you may choose a different plugin that best suits your needs. Always ensure to use plugins from reputable sources, and consider checking the documentation and support of the plugin you choose to ensure compatibility and reliability.