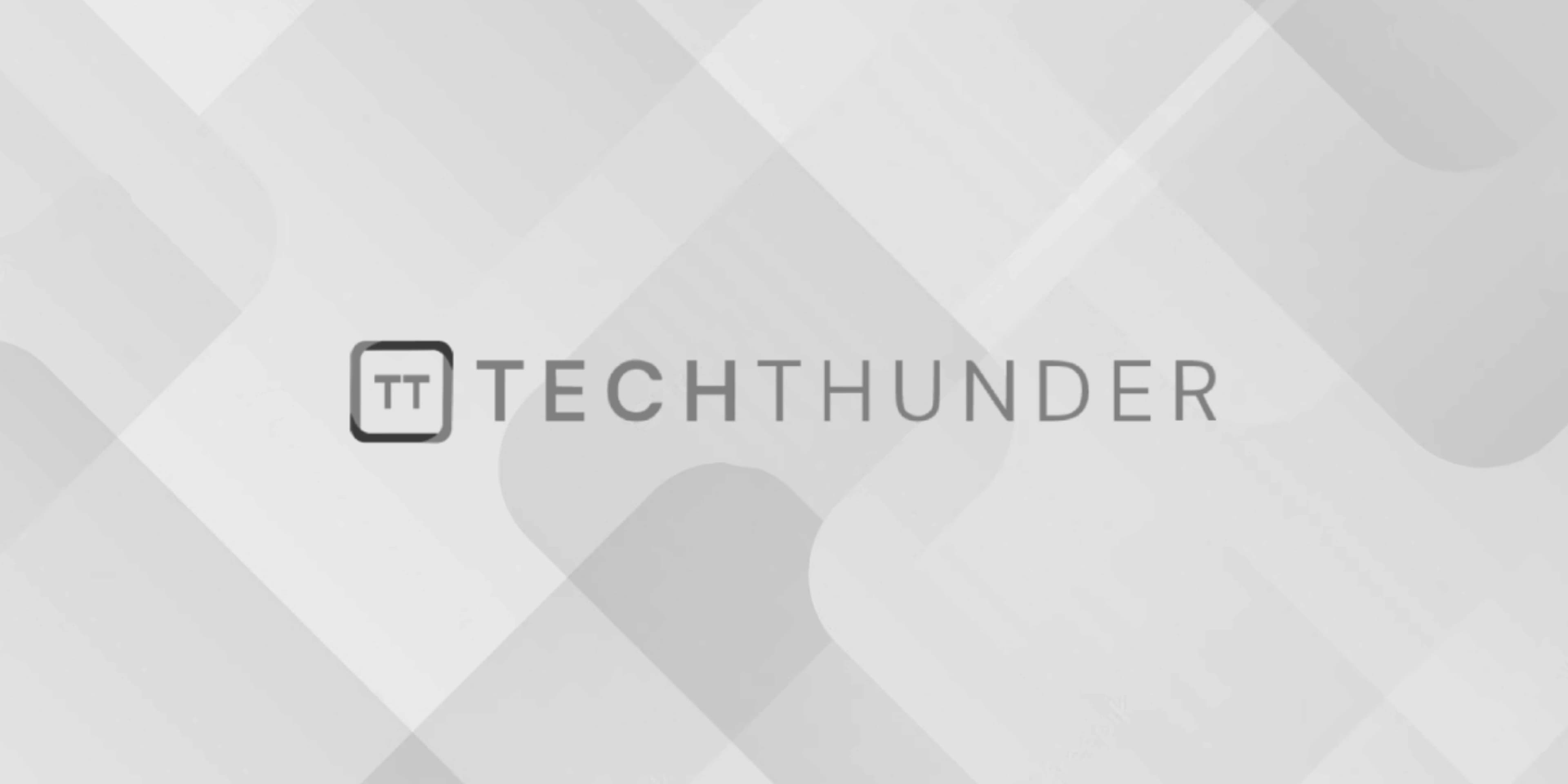
How to add options to select element using jQuery
To add options to a select element using jQuery, you can use the append()
or prepend()
method to insert new <option>
elements into the select element. The append()
method adds options to the end of the select element, while the prepend()
method adds options to the beginning. Here’s how you can do it:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Add Options to Select Element</title>
</head>
<body>
<select id="mySelect">
<!-- Existing options -->
<option value="option1">Option 1</option>
<option value="option2">Option 2</option>
</select>
<button id="addOptionBtn">Add Option</button>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
JavaScript (script.js):
$(document).ready(function() {
// Add option to select element on button click
$('#addOptionBtn').click(function() {
var optionValue = 'option3';
var optionText = 'Option 3';
// Use append() to add option to the end of the select element
$('#mySelect').append($('<option>', {
value: optionValue,
text: optionText
}));
// Alternatively, use prepend() to add option to the beginning of the select element
// $('#mySelect').prepend($('<option>', {
// value: optionValue,
// text: optionText
// }));
});
});
In this example, we have an existing select element with two options: “Option 1” and “Option 2”. When the button with the ID addOptionBtn
is clicked, a new option with the value “option3” and the text “Option 3” will be added to the select element using the append()
method. If you want to add the option to the beginning of the select element, you can use the prepend()
method instead, as shown in the commented code.
You can dynamically generate the optionValue
and optionText
variables based on user input or data from the server. The append()
and prepend()
methods allow you to create options dynamically and add them to the select element as needed.