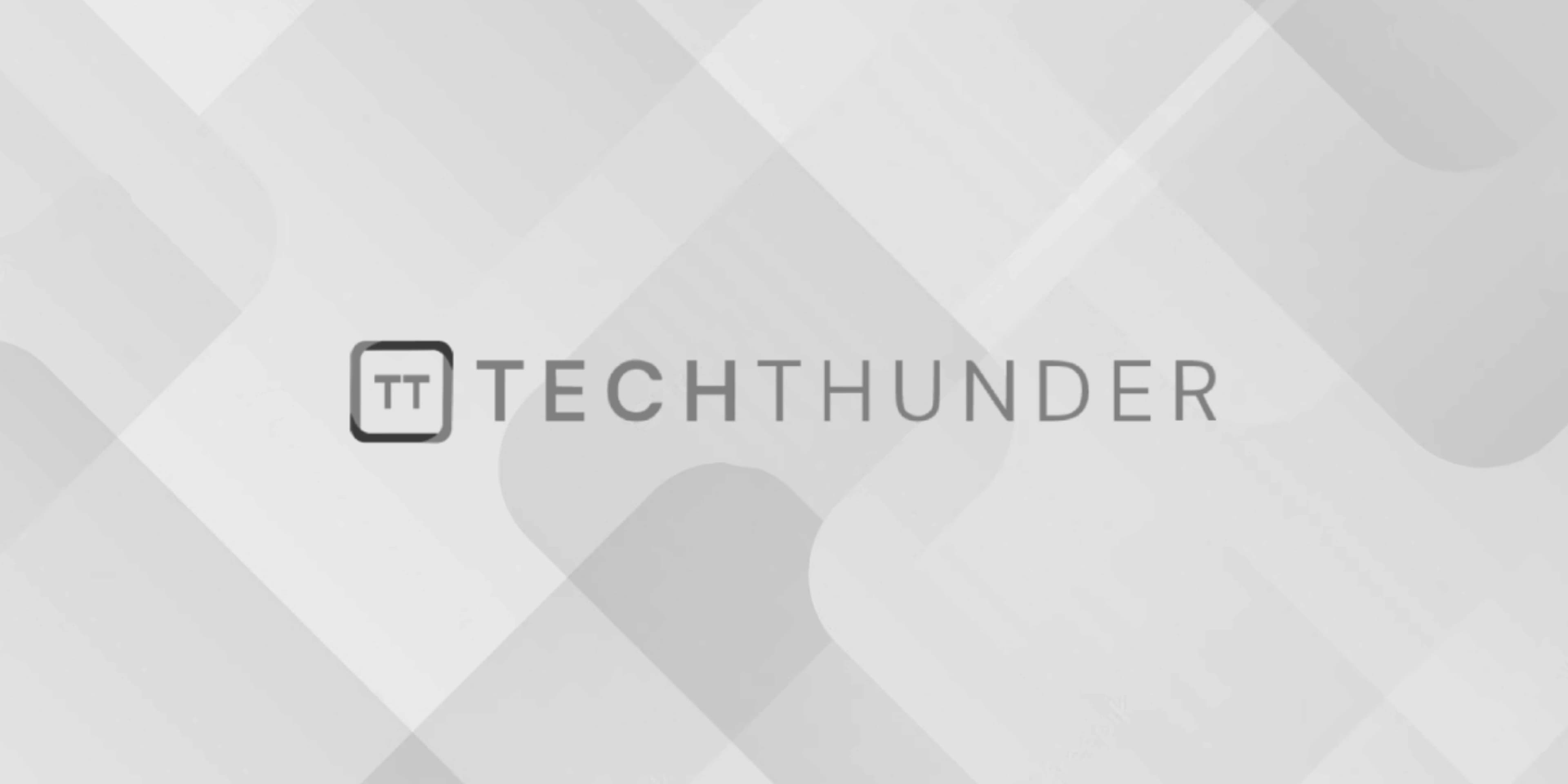
192 views
jQuery size() method
The size()
method in jQuery has been deprecated as of jQuery version 1.8, and it was removed in jQuery version 3.0. The size()
method was used to get the number of elements in a jQuery object, which is equivalent to the length
property of the jQuery object.
Deprecated size()
method example:
<!DOCTYPE html>
<html>
<head>
<title>Deprecated jQuery size() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<script>
$(document).ready(function() {
var listItems = $("ul li");
var numberOfItems = listItems.size();
console.log("Number of list items: " + numberOfItems);
});
</script>
</body>
</html>
Instead of using the size()
method, you can directly use the length
property of the jQuery object to achieve the same result:
Current approach using length
property:
<!DOCTYPE html>
<html>
<head>
<title>jQuery length Property Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<script>
$(document).ready(function() {
var listItems = $("ul li");
var numberOfItems = listItems.length;
console.log("Number of list items: " + numberOfItems);
});
</script>
</body>
</html>
Both examples will produce the same output in the console, showing the number of list items, which is 3 in this case.
Using the length
property is the recommended approach since the size()
method has been deprecated and removed from jQuery.