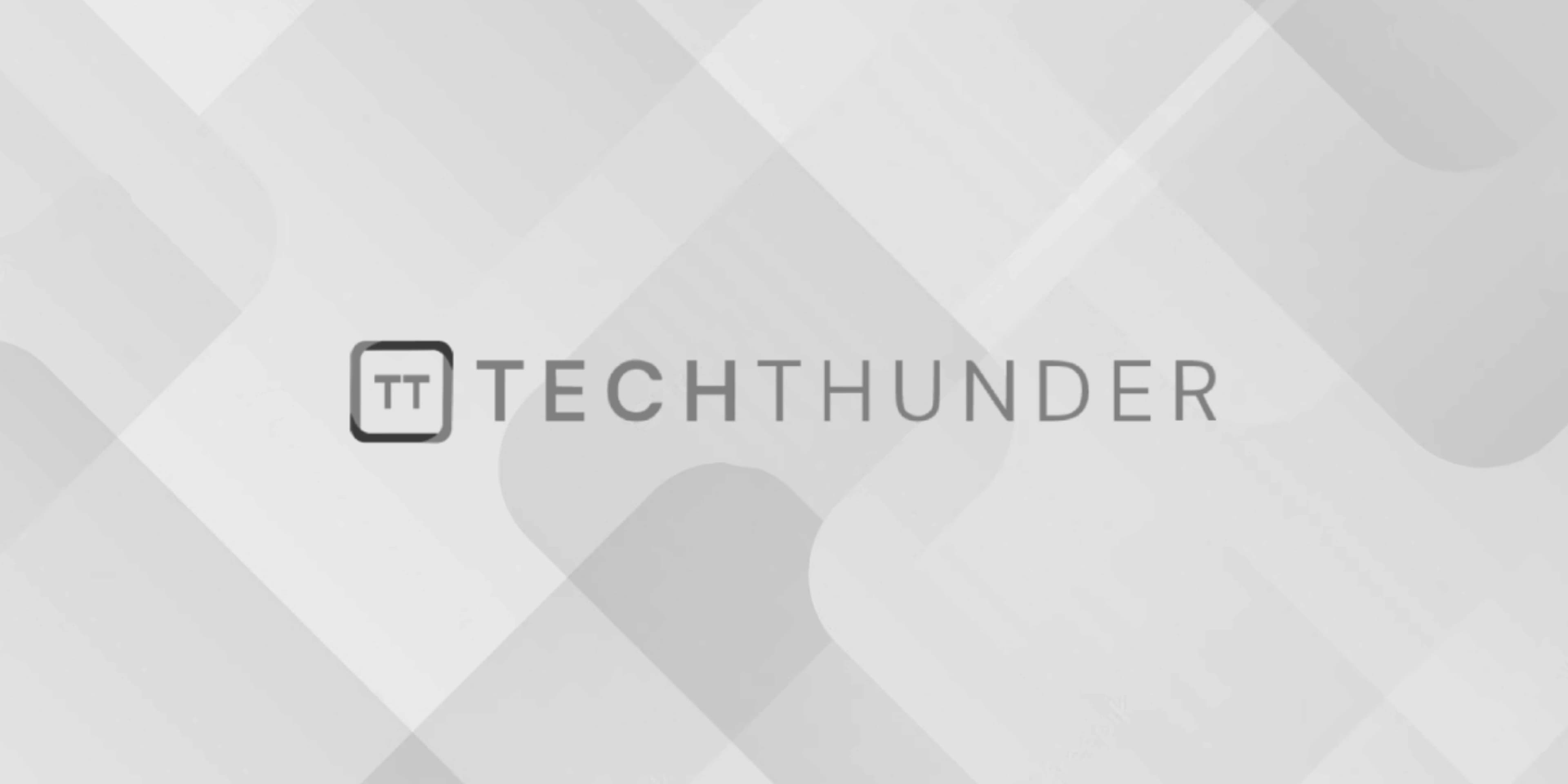
jQuery error() method
The jQuery.error()
method in jQuery. However, jQuery provides various error-handling mechanisms that you can use to handle errors in your code.
error
event:
jQuery allows you to attach an event handler to theerror
event of elements like images, scripts, or iframes. This event is triggered when an error occurs while loading the resource.
Example of handling the error
event for an image:
<!DOCTYPE html>
<html>
<head>
<title>jQuery error() Event Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<img src="nonexistent-image.jpg" alt="Error Example" id="myImage">
<script>
$(document).ready(function() {
// Attach error event handler to the image
$("#myImage").on("error", function() {
console.log("Error loading image.");
});
});
</script>
</body>
</html>
In this example, the error
event is triggered because the specified image file (“nonexistent-image.jpg”) does not exist. The event handler logs an error message to the console.
try...catch
:
For handling JavaScript errors in general, you can use thetry...catch
statement. This allows you to catch and handle errors gracefully without the script stopping execution.
Example using try...catch
:
try {
// Code that may cause an error
undefinedFunction(); // This function does not exist, so an error will occur
} catch (error) {
// Handle the error
console.log("An error occurred:", error.message);
}
In this example, the try...catch
block will catch the error that occurs when calling the undefinedFunction()
, and the error message will be logged to the console.
Remember that while jQuery provides ways to handle specific errors related to certain elements (e.g., images, scripts), standard JavaScript error-handling techniques like try...catch
can be used to handle other types of errors within your code.
If you are referring to a specific jQuery.error()
method or feature that was introduced or updated after my last update, please consult the official jQuery documentation for the most up-to-date information on jQuery methods and features.