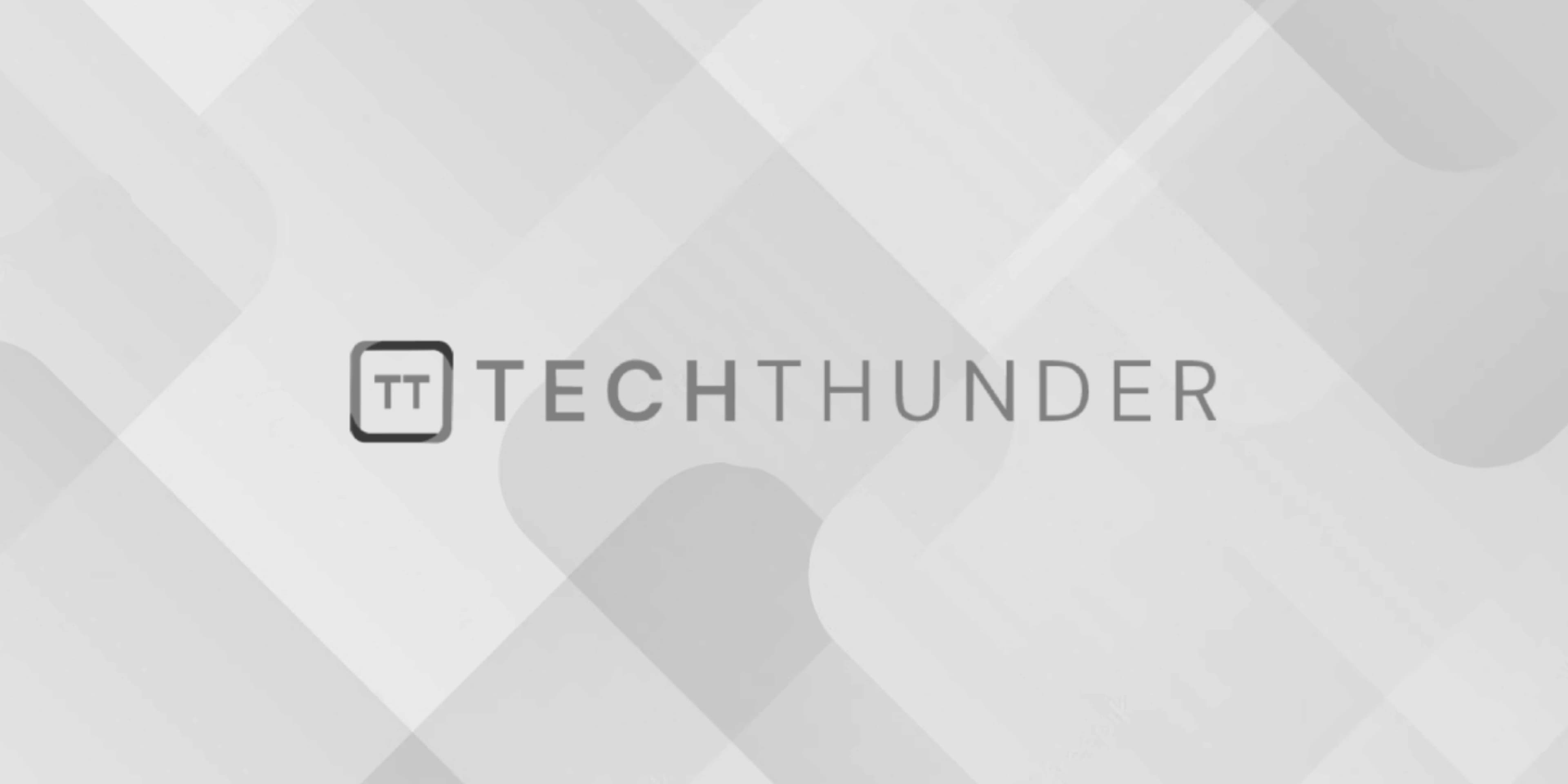
jQuery noop() method
The noop()
method in jQuery is a utility function that does nothing when called. It is a shorthand for “no operation.” The purpose of this method is to provide a function that can be used as a placeholder or default callback when an actual function is expected but no action needs to be taken.
Here’s the basic syntax of the noop()
method:
$.noop()
Return Value:
The noop()
method doesn’t return any value.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery noop() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="submitButton">Submit</button>
<script>
$(document).ready(function() {
// Attach a click event handler to the submit button
$("#submitButton").click(function() {
// Perform some validation or data processing here
if ($("#nameInput").val() === "") {
// If the name input is empty, prevent the form submission
// and show an error message (using noop() as a placeholder)
alert("Please enter your name.");
return $.noop();
}
// Otherwise, continue with form submission (noop() acts as a placeholder here too)
return $.noop();
});
});
</script>
</body>
</html>
In this example, we have a form with a submit button. When the submit button is clicked, the click
event handler function is executed. Inside the function, we perform some validation to check if the name input is empty. If the name input is empty, we prevent the form submission and show an error message using alert()
. In this case, we use $.noop()
as a placeholder to return from the event handler without performing any further action.
Using noop()
in this context indicates that we intentionally do nothing, effectively bypassing any additional logic that might have followed. It serves as a cleaner and more explicit alternative to using an empty function or returning false
, especially when there is no real action to be taken in a particular scenario.