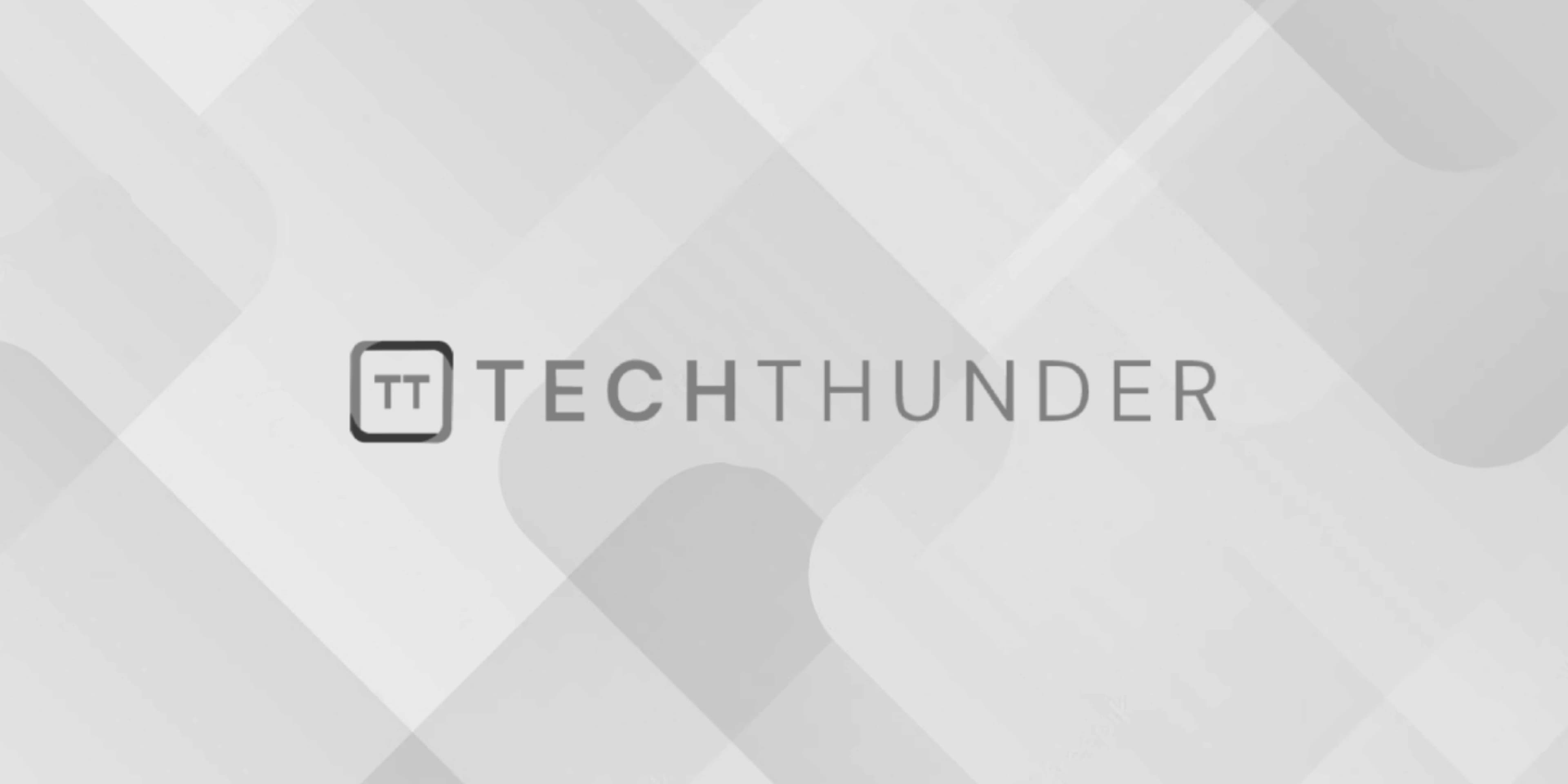
How to Convert an HTML Table into Excel Spreadsheet using jQuery
To convert an HTML table into an Excel spreadsheet using jQuery, you can use the “TableExport” library, which allows exporting HTML tables to various formats, including Excel (XLSX). The library simplifies the process of generating downloadable Excel files from HTML tables.
Here’s a step-by-step guide to using the “TableExport” library to convert an HTML table into an Excel spreadsheet:
- Create the HTML table:
<!DOCTYPE html>
<html>
<head>
<title>HTML Table to Excel Spreadsheet</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="table-container">
<h1>Employee Data</h1>
<table id="employee-table">
<thead>
<tr>
<th>Name</th>
<th>Age</th>
<th>Department</th>
<th>Salary</th>
</tr>
</thead>
<tbody>
<tr>
<td>John Doe</td>
<td>30</td>
<td>IT</td>
<td>$50000</td>
</tr>
<tr>
<td>Jane Smith</td>
<td>28</td>
<td>HR</td>
<td>$45000</td>
</tr>
<!-- Add more rows if needed -->
</tbody>
</table>
<button id="export-btn">Export to Excel</button>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="tableexport.min.js"></script>
<script src="script.js"></script>
</body>
</html>
- Create the CSS (styles.css):
body {
font-family: Arial, sans-serif;
text-align: center;
}
.table-container {
max-width: 600px;
margin: 50px auto;
padding: 20px;
border: 2px solid #ccc;
border-radius: 8px;
}
h1 {
margin-top: 0;
}
table {
width: 100%;
border-collapse: collapse;
}
th, td {
border: 1px solid #ccc;
padding: 8px;
}
button {
margin-top: 10px;
padding: 8px 16px;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 4px;
cursor: pointer;
}
- Implement the JavaScript (script.js):
$(document).ready(function() {
$('#export-btn').on('click', function() {
exportToExcel();
});
function exportToExcel() {
const table = document.getElementById('employee-table');
TableExport(table, {
formats: ['xlsx'],
filename: 'employee_data',
exportButtons: false,
}).exportFile();
}
});
- Download the tableexport.min.js file from the TableExport GitHub repository: https://github.com/clarketm/TableExport
Place the downloaded tableexport.min.js file in the same directory as your HTML file.
When you click the “Export to Excel” button, the HTML table will be converted into an Excel spreadsheet (XLSX format) and downloaded to your device.
Please note that using a third-party library like “TableExport” provides an easy way to convert an HTML table into an Excel file. However, there are other methods to achieve this functionality as well, depending on your specific requirements and the tools available in your environment.