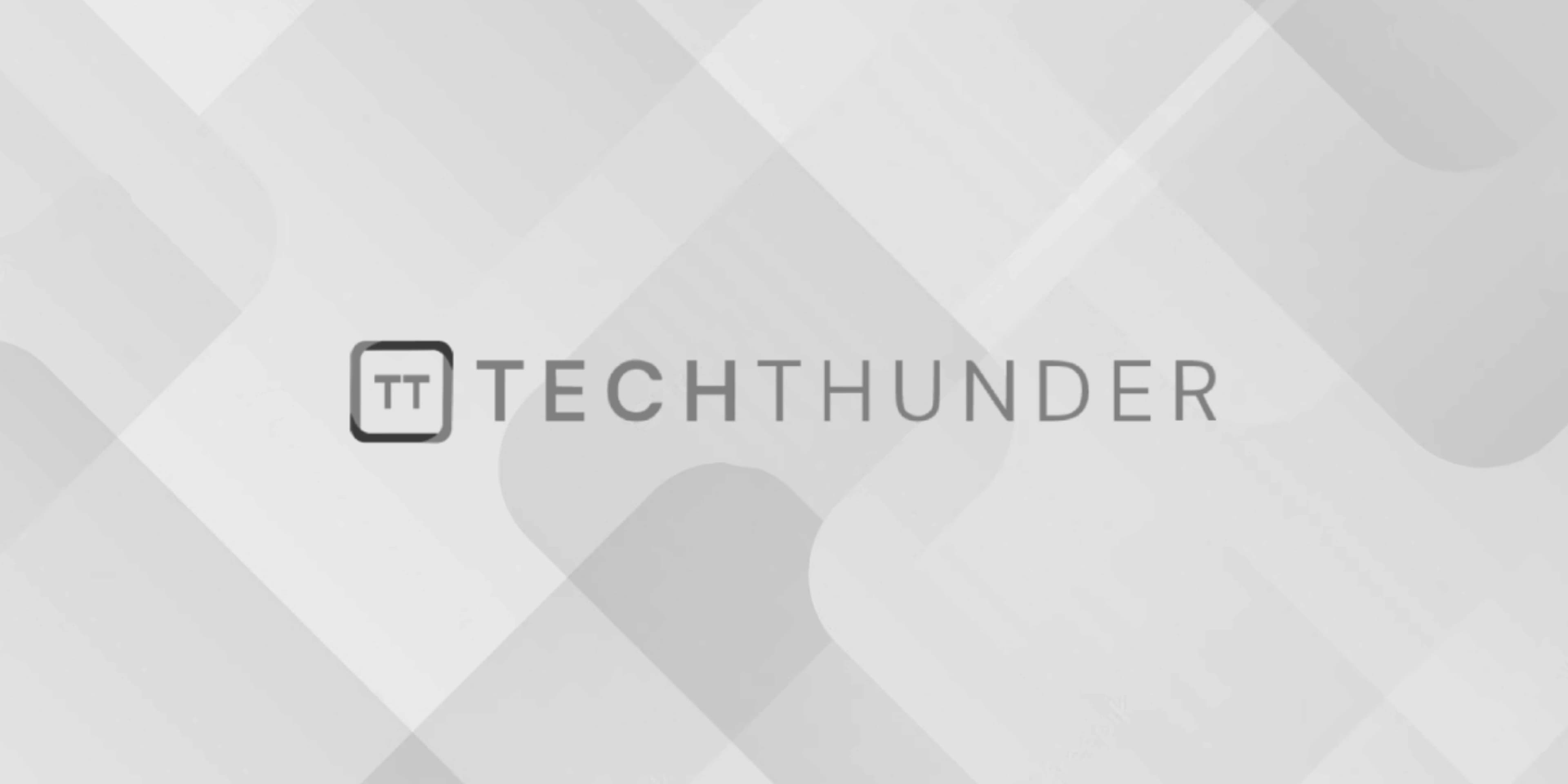
jQuery :nth-child selector
The jQuery :nth-child
selector is used to select elements based on their position within their parent element. It allows you to target elements that match a specific index or formula within their parent container.
Here’s the basic syntax of the :nth-child
selector:
$(parentSelector).find(':nth-child(n)')
Parameters:
parentSelector
: A selector string or jQuery object representing the parent element that contains the elements you want to target.n
: An integer, keyword, or formula that specifies which elements to select based on their position within the parent element. The formula follows the formatan+b
, wherea
andb
are integers, andn
represents the index of the child elements (1-indexed).
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery :nth-child Selector Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
<li>Item 5</li>
</ul>
<script>
$(document).ready(function() {
// Select the first and third <li> elements within the <ul>
$('ul li:nth-child(1), ul li:nth-child(3)').addClass('highlight');
});
</script>
<style>
.highlight {
background-color: yellow;
}
</style>
</body>
</html>
In this example, we have an unordered list (<ul>
) with five list items (<li>
). We use the :nth-child()
selector to select the first and third list items within the <ul>
and apply the highlight
class to them. As a result, the first and third list items will have a yellow background.
You can use the :nth-child
selector to target specific elements within a parent container based on their position. It’s particularly useful for styling or manipulating elements in patterns based on their order in the DOM.