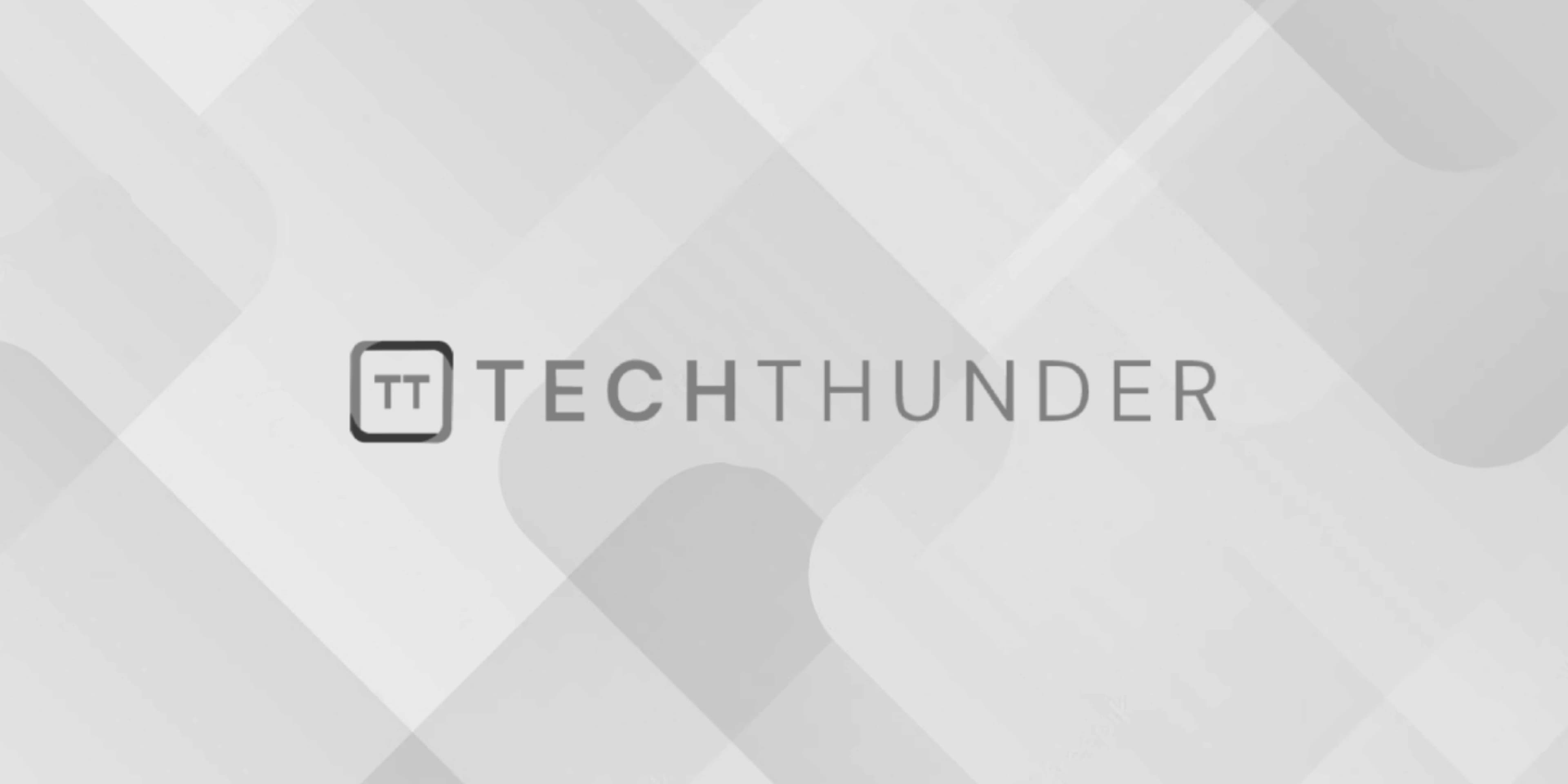
Full Screen video Background using jquery
To create a full-screen video background using jQuery, you can use the HTML5 <video>
element for the video and CSS to handle the full-screen display. jQuery can be used to manipulate the video element and handle any additional interactions or events if required.
Here’s a simple example of how to create a full-screen video background using jQuery:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Full-Screen Video Background</title>
<style>
body, html {
margin: 0;
padding: 0;
height: 100%;
overflow: hidden;
}
#video-container {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
z-index: -1;
overflow: hidden;
}
#video-background {
width: 100%;
height: 100%;
object-fit: cover;
}
.content {
position: relative;
z-index: 1;
padding: 20px;
color: #fff;
}
</style>
</head>
<body>
<div id="video-container">
<video id="video-background" autoplay muted loop>
<source src="your-video-file.mp4" type="video/mp4">
<!-- Include additional video formats for cross-browser support -->
</video>
</div>
<div class="content">
<!-- Your website content goes here -->
<h1>Welcome to our Website</h1>
<p>This is some content on the page.</p>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
JavaScript (script.js):
$(document).ready(function() {
// Adjust the video container height to the window height
function adjustVideoHeight() {
var windowHeight = $(window).height();
$('#video-container').height(windowHeight);
}
// Call the function on page load and window resize
adjustVideoHeight();
$(window).on('resize', adjustVideoHeight);
});
In this example, the video is played in a full-screen video container (#video-container
) that covers the entire viewport. The video itself is set to be object-fit: cover
, which ensures that it scales proportionally to cover the entire container without distorting.
Replace "your-video-file.mp4"
in the <source>
tag with the URL or path to your video file. Additionally, include additional <source>
tags with different video formats (e.g., WebM, Ogg) for cross-browser support.
The JavaScript code in the script.js
file ensures that the video container height is adjusted to the window height on page load and whenever the window is resized, maintaining the full-screen video effect even when the window size changes.
Please note that full-screen video backgrounds can consume more bandwidth and resources, so use them judiciously and consider providing alternative content for users on devices or browsers that do not support video or autoplaying videos. Additionally, ensure that you have the appropriate rights to use the video content and comply with any copyright or licensing restrictions.