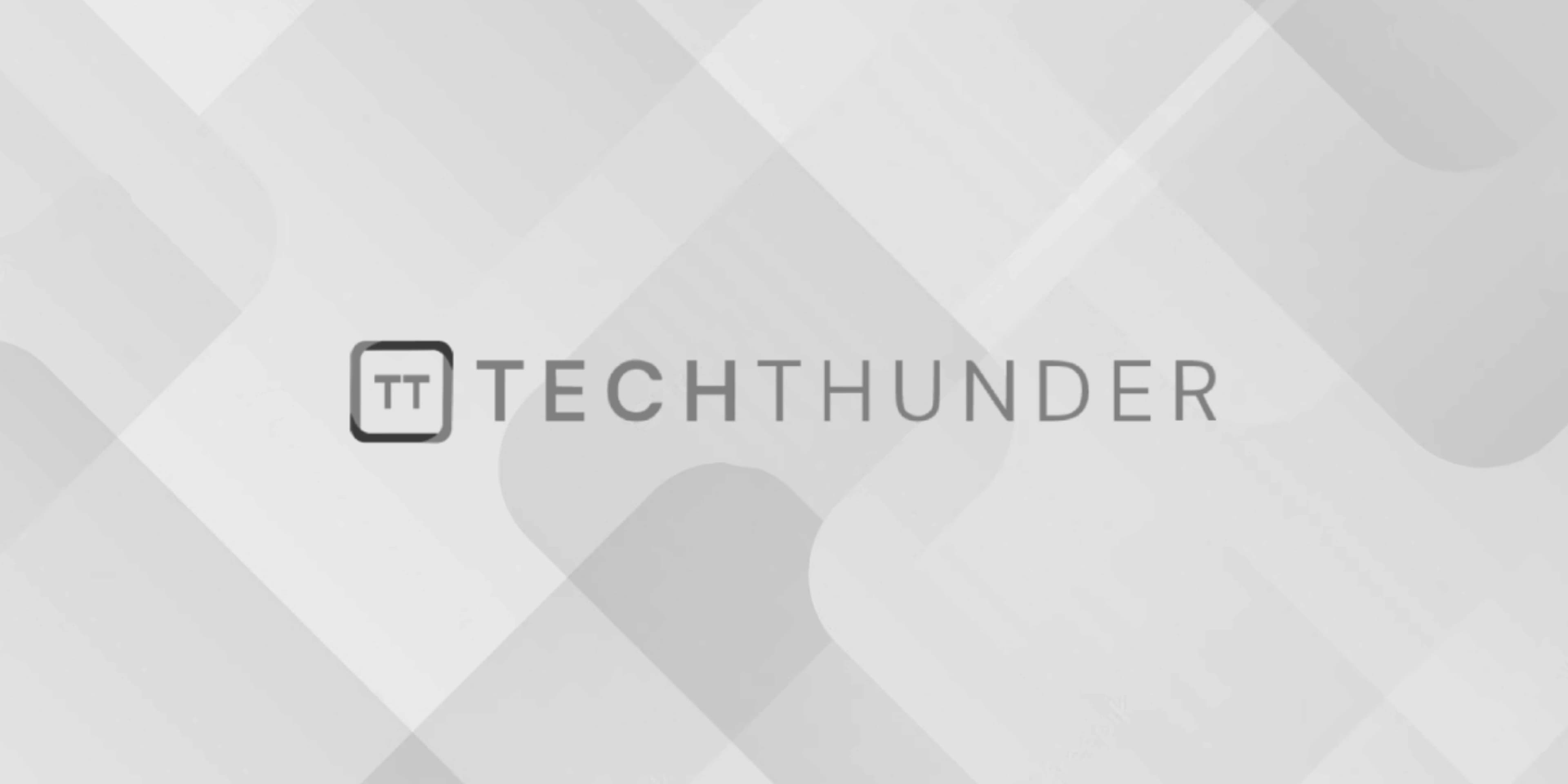
jQuery merge() method
The jQuery provides a built-in jQuery.merge()
function that can be used to merge two arrays or jQuery objects.
Here’s the syntax for jQuery.merge()
:
jQuery.merge(target, source);
Parameters:
target
: The array or jQuery object that will receive the merged elements.source
: The array or jQuery object whose elements will be merged into thetarget
.
Return Value:
The jQuery.merge()
function returns the target
array or jQuery object after the elements from the source
have been merged into it.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery merge() Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<ul id="list1">
<li>Item 1</li>
<li>Item 2</li>
</ul>
<ul id="list2">
<li>Item 3</li>
<li>Item 4</li>
</ul>
<script>
$(document).ready(function() {
// Merge two jQuery objects
var $list1 = $('#list1 li');
var $list2 = $('#list2 li');
var $mergedList = $.merge($list1, $list2);
// Log the merged jQuery object
console.log($mergedList);
// Output the content of the merged list
$mergedList.each(function(index) {
console.log("Merged Item " + index + ":", $(this).text());
});
});
</script>
</body>
</html>
In this example, we use the jQuery.merge()
function to merge two jQuery objects representing two lists ($list1
and $list2
) into a new jQuery object $mergedList
. The merged list contains all the list items from both lists.
The output of the above example will be:
jQuery.fn.init(4)
[li, li, li, li]
Merged Item 0: Item 1 Merged Item 1: Item 2 Merged Item 2: Item 3 Merged Item 3: Item 4
In this output, we see that the elements from both lists have been successfully merged into the $mergedList
jQuery object, and we can access and manipulate them together.
Remember that jQuery.merge()
is a general-purpose utility function that can be used to merge arrays or jQuery objects. If you want to perform element manipulation within the context of a specific jQuery object, you should use the appropriate jQuery methods and functions that directly apply to jQuery objects, like .add()
, .append()
, .extend()
, etc.