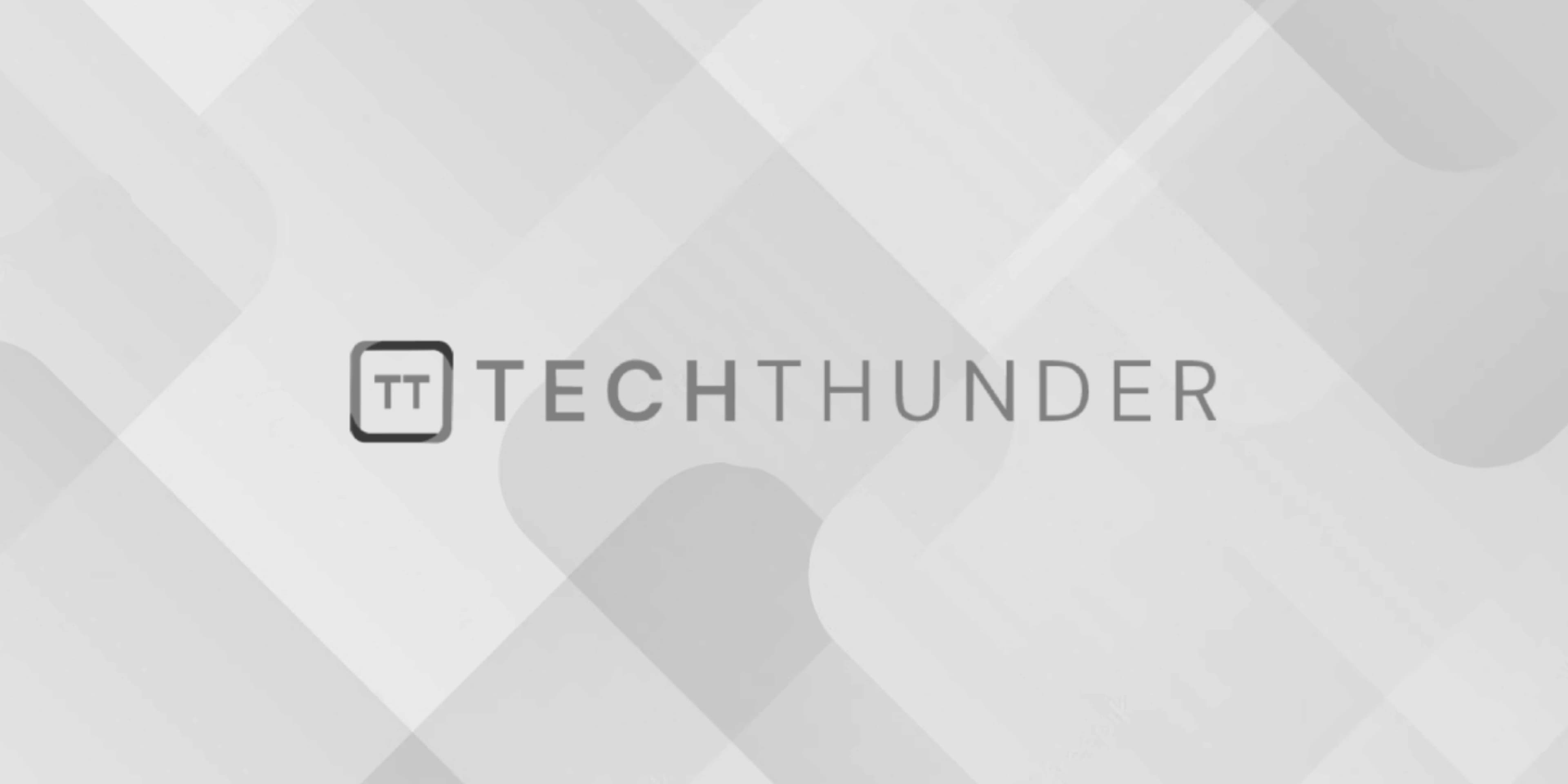
jQuery siblings() method
The jQuery siblings()
method is used to select all the sibling elements of the matched elements. Sibling elements are elements that share the same parent element in the HTML structure and appear next to each other in the document.
Here’s the basic syntax of the siblings()
method:
$(selector).siblings()
Parameters:
selector
: A selector expression used to filter the sibling elements further. It is optional.
Return Value:
The siblings()
method returns a jQuery object containing all the sibling elements that match the optional selector.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery siblings() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<ul>
<li>Item 1</li>
<li class="selected">Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
</ul>
<script>
$(document).ready(function() {
// Select all sibling elements of the element with class "selected"
var siblings = $(".selected").siblings();
// Add a class to the selected sibling elements for styling
siblings.addClass("highlight");
});
</script>
<style>
.highlight {
background-color: yellow;
}
</style>
</body>
</html>
In this example, we have an unordered list (<ul>
) with four list items (<li>
). One of the list items has the class “selected.” We use the siblings()
method to select all the sibling elements of the list item with the class “selected.” We then add a class called “highlight” to these sibling elements to apply a yellow background to them.
When you run the code, you will see that all the sibling elements of the list item with the class “selected” (excluding the “selected” item itself) are highlighted with a yellow background.
The siblings()
method is useful when you want to manipulate or style elements that share the same parent and appear next to each other in the HTML structure. It allows you to target elements without having to know their specific positions or indices in the parent element’s children.