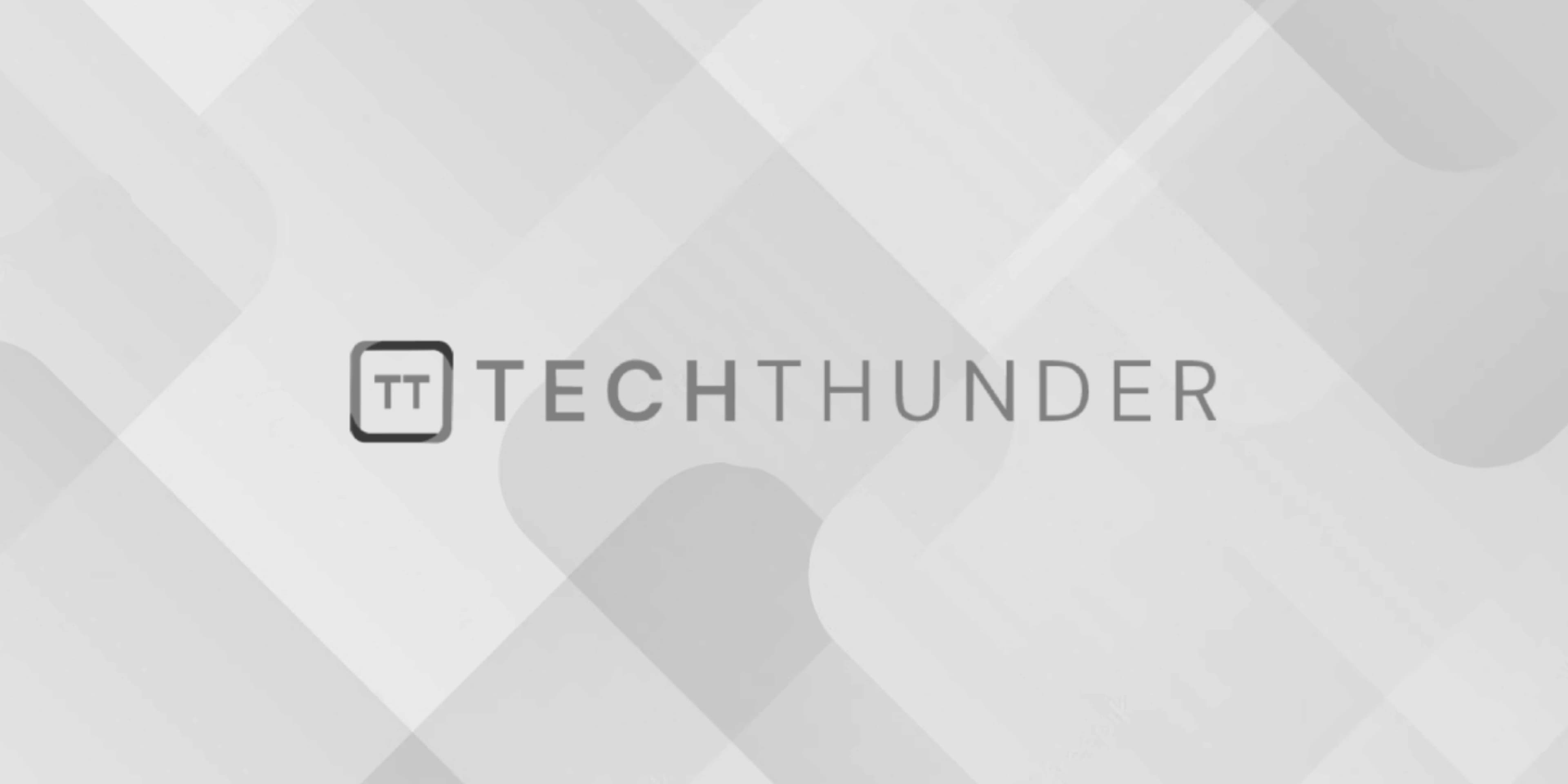
jQuery $.proxy() method
The $.proxy()
method in jQuery is used to create a new function with a specific context (i.e., the value of the this
keyword) for the original function. It is often used to ensure that a function is called with a particular object as its context, regardless of how it is called or where it is invoked.
Here’s the basic syntax of the $.proxy()
method:
$.proxy(function, context)
Parameters:
function
: The original function whose context you want to modify.context
: The object to be used as the context (the value ofthis
) when the function is called.
Return Value:
The $.proxy()
method returns a new function that wraps the original function and sets the specified context as its this
value when the new function is invoked.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery $.proxy() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="myButton">Click Me</button>
<script>
$(document).ready(function() {
var myObject = {
name: "John",
sayHello: function() {
console.log("Hello, " + this.name);
}
};
// Create a new function with myObject as the context using $.proxy()
var proxyFunction = $.proxy(myObject.sayHello, myObject);
// Bind the click event to the new proxyFunction
$("#myButton").on("click", proxyFunction);
});
</script>
</body>
</html>
In this example, we have an object myObject
with a sayHello()
method. When the button with the id “myButton” is clicked, we want to call the sayHello()
method of myObject
, but we want to ensure that myObject
is used as the context (this
) inside the method.
We use $.proxy(myObject.sayHello, myObject)
to create a new function proxyFunction
that wraps myObject.sayHello
and sets myObject
as the context. We then bind the proxyFunction
to the click event of the button.
When you click the button, the sayHello()
method will be called with myObject
as the context, and it will log “Hello, John” to the console.
The $.proxy()
method is particularly useful when you need to pass a function as a callback, but you want to ensure that the function is executed with a specific context. It helps to avoid issues with the value of this
being lost or set to the wrong object when the function is invoked.