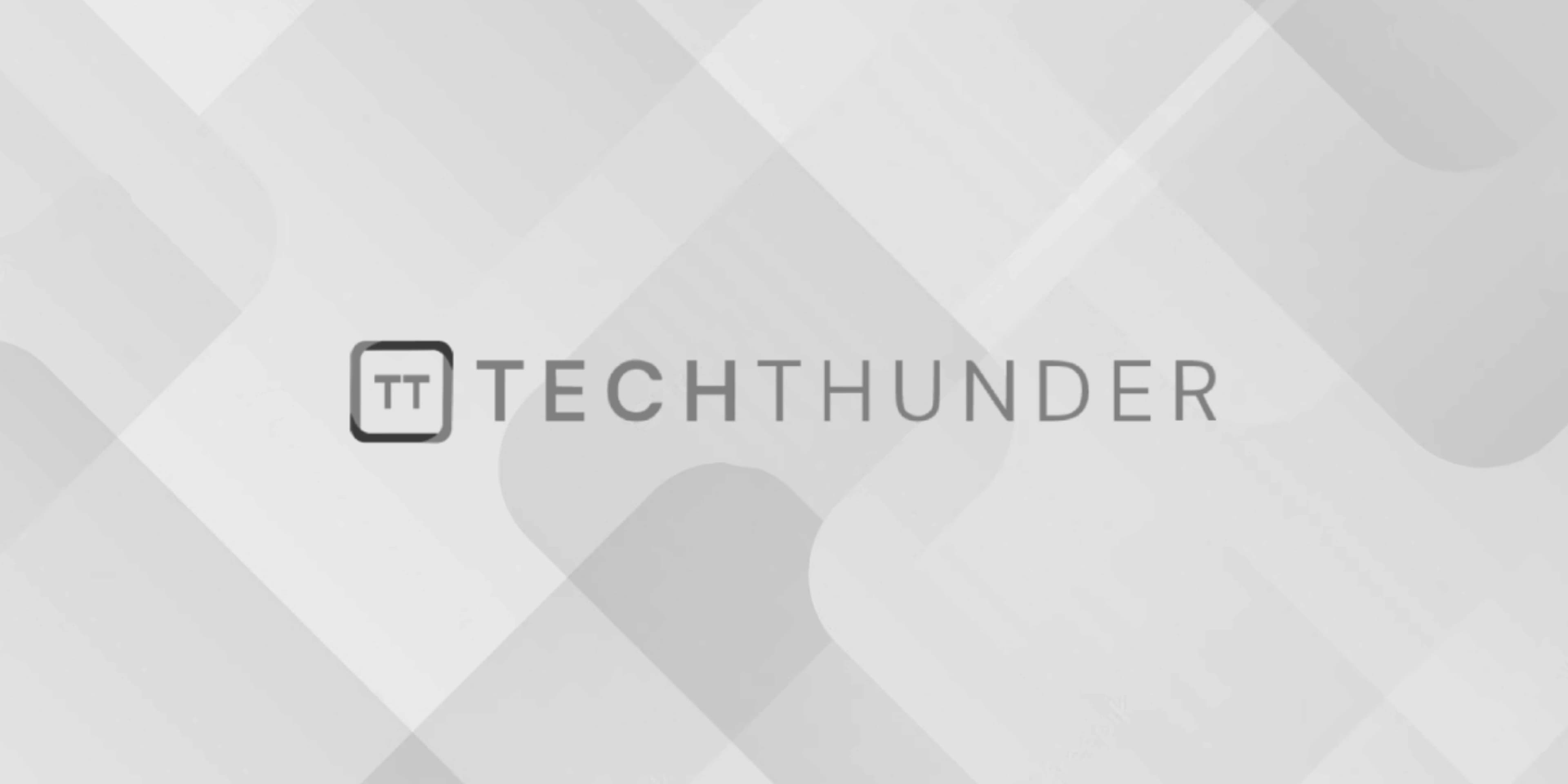
jQuery val() method
The jQuery val()
method is used to get or set the current value of form elements, such as input fields, select boxes, and textareas. It allows you to read and update the values of these form elements dynamically.
The syntax for using the val()
method is as follows:
To Get the Current Value:
$(selector).val();
To Set a New Value:
$(selector).val(newValue);
selector
: It is a string that specifies the form element(s) to be selected.newValue
(optional): It is the new value that you want to set for the form element. If you omit this parameter, theval()
method will act as a getter and return the current value.
Here’s an example of how you can use the val()
method:
HTML:
<input type="text" id="myInput" value="Default Value">
<select id="mySelect">
<option value="option1">Option 1</option>
<option value="option2">Option 2</option>
<option value="option3">Option 3</option>
</select>
JavaScript:
// Get the current value of the input field
var inputValue = $('#myInput').val();
console.log('Input Value: ' + inputValue);
// Set a new value for the input field
$('#myInput').val('New Value');
// Get the current value of the select box
var selectValue = $('#mySelect').val();
console.log('Select Value: ' + selectValue);
// Set a new value for the select box
$('#mySelect').val('option3');
In the example, the val()
method is used to get the current values of the input field with the ID “myInput” and the select box with the ID “mySelect.” The current values are logged to the console.
Then, the val()
method is used again to set new values for the input field and the select box. For the input field, the value is changed to “New Value,” and for the select box, the value is changed to “option3.”
The val()
method is commonly used when you want to interact with form elements and retrieve their values for validation, submission, or to update them based on user input or other events.
Please note that the val()
method can be used with a variety of form elements, such as text inputs, checkboxes, radio buttons, and select boxes. Additionally, when used with select boxes, it can be used to both get and set the selected option(s).