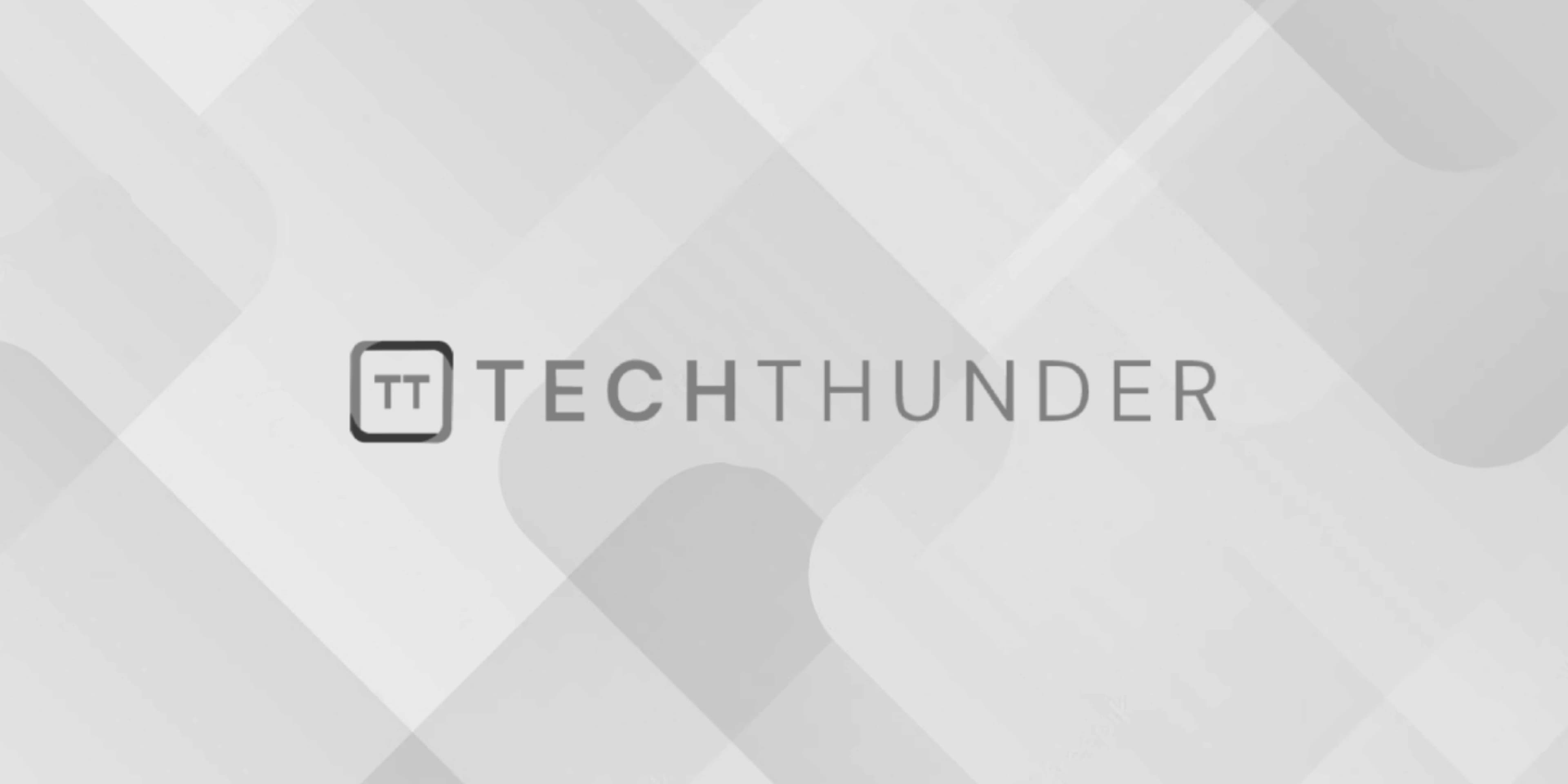
jQuery remove() method
The jQuery remove()
method is used to remove selected elements from the DOM. It completely removes the elements, including all their child elements and text nodes, from the document, making them no longer part of the HTML structure.
The syntax for using the remove()
method is as follows:
$(selector).remove();
selector
: It is a string that specifies the elements to be selected.
Here’s an example of how you can use the remove()
method:
HTML:
<div id="myDiv">
<p>This is a paragraph.</p>
<span>This is a span element.</span>
</div>
JavaScript:
// Remove the entire div element and its content from the DOM
$('#myDiv').remove();
After executing the above JavaScript code, the entire div
element with the ID “myDiv” and all its child elements will be removed from the document:
Resulting HTML:
<!-- The div and its content are completely removed from the DOM -->
It’s important to note that the remove()
method not only removes the element from the DOM but also detaches any event handlers and data associated with the element. If you need to retain any data or event handlers, you can use the .detach()
method instead.
Here’s an example using .detach()
:
// Detach the entire div element and its content (retains data and event handlers)
var detachedDiv = $('#myDiv').detach();
// Do something with the detachedDiv
// Reattach the detachedDiv back to the DOM (with data and event handlers intact)
$('body').append(detachedDiv);
The remove()
method is commonly used when you want to delete elements from the page permanently, such as when removing dynamically generated content or implementing a feature to delete certain elements based on user actions.
Remember that once an element is removed using the remove()
method, it cannot be brought back without recreating it from scratch. So, use this method with caution and ensure that you no longer need the content before removing it from the DOM.