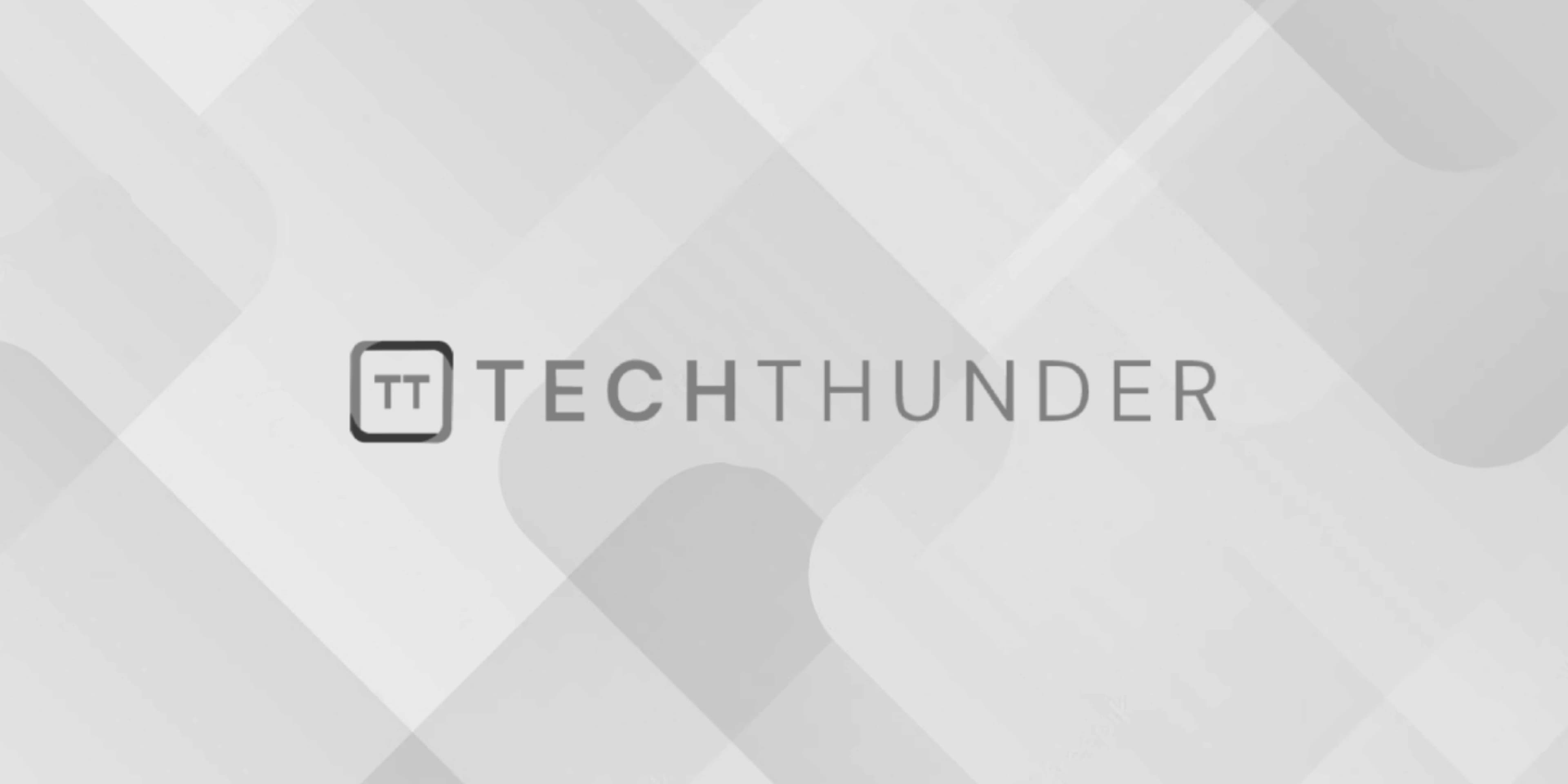
Lazy load images using jQuery
Lazy loading is a technique used to defer the loading of images until they are needed. This can help improve the page’s initial loading time and reduce unnecessary network requests for images that may not be visible to the user immediately.
To implement lazy loading for images using jQuery, you can use the “LazyLoad” library. Here’s a step-by-step guide to set it up:
- Download the “LazyLoad” library from the GitHub repository: https://github.com/verlok/lazyload
- Include the “lazyload.min.js” file and jQuery in your HTML file:
<!DOCTYPE html>
<html>
<head>
<title>Lazy Load Images</title>
</head>
<body>
<div class="image-container">
<!-- Placeholder images with data-src attribute -->
<img data-src="image1.jpg" alt="Image 1">
<img data-src="image2.jpg" alt="Image 2">
<img data-src="image3.jpg" alt="Image 3">
<!-- Add more images with data-src attribute as needed -->
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="lazyload.min.js"></script>
<script src="script.js"></script>
</body>
</html>
- Implement the JavaScript (script.js):
$(document).ready(function() {
// Initialize LazyLoad
const lazyLoadInstance = new LazyLoad({
elements_selector: ".image-container img",
});
});
In this example, we use the “LazyLoad” library to lazy load images with the data-src
attribute. The actual image URLs are set in the data-src
attribute, while the src
attribute is initially left empty. As the user scrolls down the page and the images come into view, the “LazyLoad” library will automatically load the images.
Please ensure that the “lazyload.min.js” file is placed in the same directory as your HTML file for it to work correctly.
By using lazy loading, images will only be fetched and loaded when the user is likely to see them, leading to faster initial page load times and better user experience.