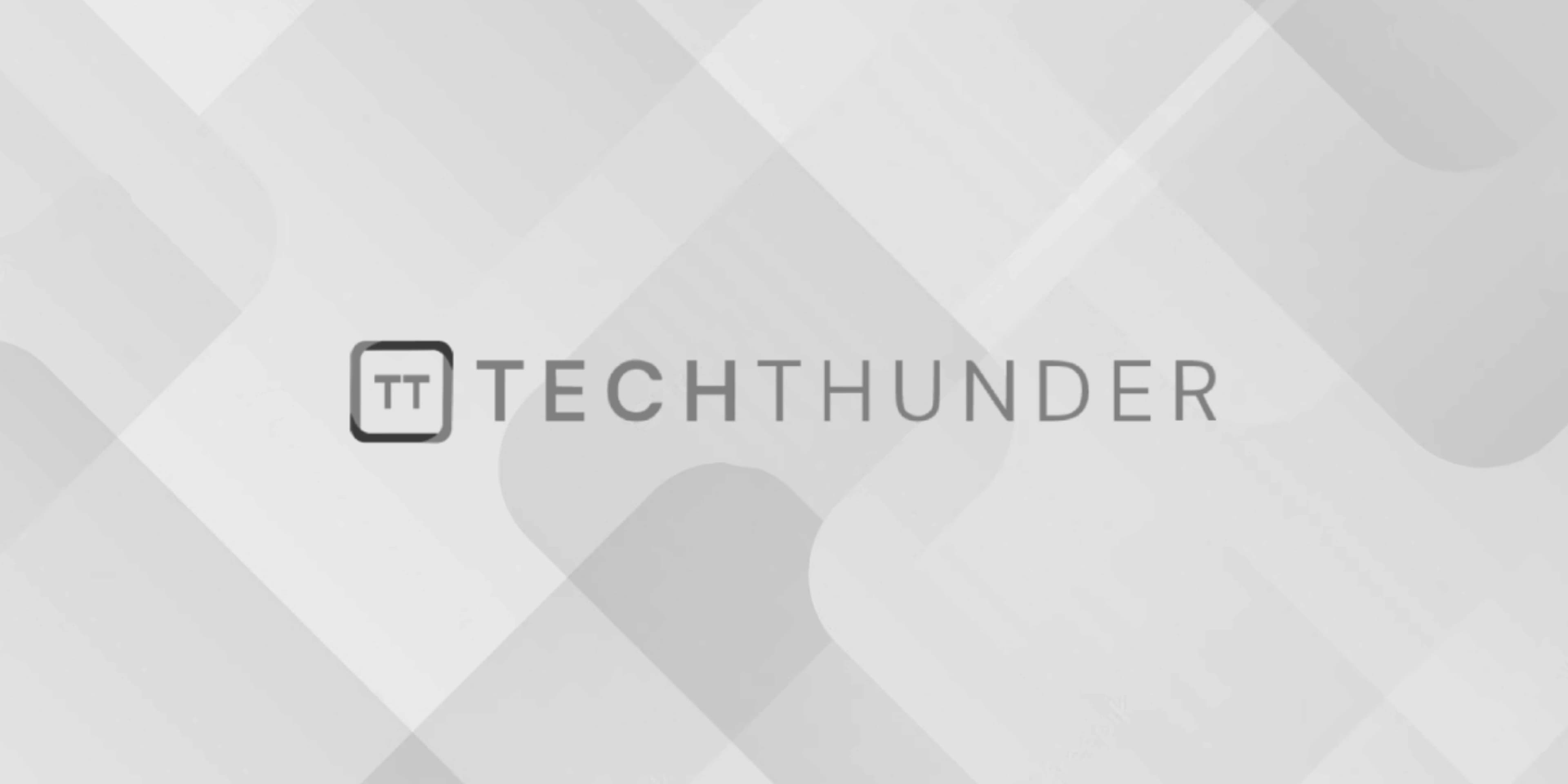
JQuery Blockrain/Tetris game
Creating a full Tetris game from scratch using jQuery and HTML5 canvas is a complex task and cannot be covered in a single response. However, I can provide you with an overview of how to implement a simple version of the Tetris game using jQuery and HTML5 canvas.
In this simplified version, we will create a basic grid, handle block movements, and perform basic collision detection.
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Tetris Game</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<canvas id="gameCanvas" width="200" height="400"></canvas>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
CSS (styles.css):
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f2f2f2;
}
#gameCanvas {
border: 2px solid #333;
}
JavaScript (script.js):
“`js
$(document).ready(function() {
const canvas = document.getElementById(‘gameCanvas’);
const ctx = canvas.getContext(‘2d’);
const blockSize = 20;
const rows = 20;
const cols = 10;
const board = createEmptyBoard();
let currentBlock = getRandomBlock();
let currentX = Math.floor(cols / 2) – Math.floor(currentBlock[0].length / 2);
let currentY = 0;
function createEmptyBoard() {
return Array.from({ length: rows }, () => Array(cols).fill(0));
}
function getRandomBlock() {
const blocks = [
[
[1, 1, 1, 1],
],
[
[2, 2],
[2, 2],
],
[
[3, 0],
[3, 3],
[0, 3],
],
[
[0, 4, 4],
[4, 4, 0],
],
[
[5, 5, 0],
[0, 5, 5],
],
[
[0, 6, 0],
[6, 6, 6],
],
[
[7, 7, 7],
[0, 7, 0],
],
];
return blocks[Math.floor(Math.random() * blocks.length)];
}
function drawBlock(x, y, block) {
for (let r = 0; r < block.length; r++) {
for (let c = 0; c < block[r].length; c++) {
if (block[r][c] !== 0) {
ctx.fillStyle = colors[block[r][c]];
ctx.fillRect((x + c) * blockSize, (y + r) * blockSize, blockSize, blockSize);
ctx.strokeStyle = ‘#333’;
ctx.strokeRect((x + c) * blockSize, (y + r) * blockSize, blockSize, blockSize);
}
}
}
}
function drawBoard() {
for (let r = 0; r < rows; r++) {
for (let c = 0; c < cols; c++) {
if (board[r][c] !== 0) {
ctx.fillStyle = colors[board[r][c]];
ctx.fillRect(c * blockSize, r * blockSize, blockSize, blockSize);
ctx.strokeStyle = ‘#333’;
ctx.strokeRect(c * blockSize, r * blockSize, blockSize, blockSize);
}
}
}
}
function clearBoard() {
board.forEach(row => row.fill(0));
}
function canMove(block, x, y) {
for (let r = 0; r < block.length; r++) { for (let c = 0; c < block[r].length; c++) { if (block[r][c] !== 0) { if (x + c < 0 || x + c >= cols || y + r >= rows || board[y + r][x + c] !== 0) {
return false;
}
}
}
}
return true;
}
function mergeBlock(block, x, y) {
for (let r = 0; r < block.length; r++) {
for (let c = 0; c < block[r].length; c++) {
if (block[r][c] !== 0) {
board[y + r][x + c] = block[r][c];
}
}
}
}
function checkLines() {
for (let r = rows – 1; r >= 0; r–) {
if (board[r].every(cell => cell !== 0)) {
board.splice(r, 1);
board.unshift(Array(cols).fill(0));
}
}
}
function rotateBlock(block) {
const rotatedBlock = [];
for (let c = 0; c < block[0].length; c++) { rotatedBlock[c] = []; for (let r = block.length – 1; r >= 0; r–) {
rotatedBlock[c].push(block[r][c]);
}
}
return rotatedBlock;
}
function update() {