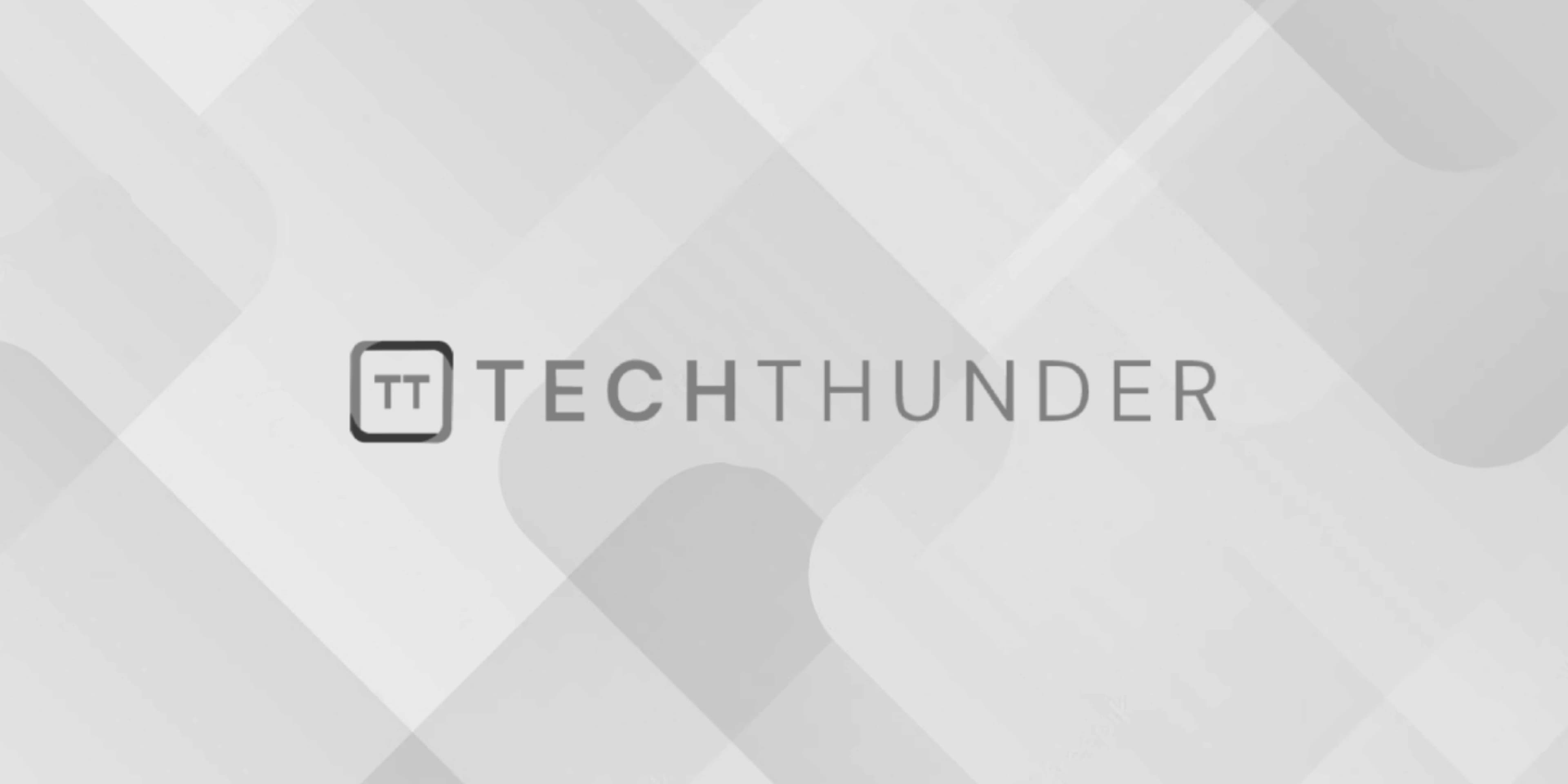
jQuery Filter Table
To implement a jQuery filter table functionality, you can use the jQuery library along with HTML and CSS. The goal is to create an interactive table where users can search and filter the rows based on their input.
Here’s a step-by-step guide on how to create a simple jQuery filter table:
Step 1: Set Up HTML Structure
Create an HTML table with the header row containing column headers and subsequent rows with data.
<!DOCTYPE html>
<html>
<head>
<title>jQuery Filter Table</title>
<style>
table {
width: 100%;
border-collapse: collapse;
}
th, td {
padding: 8px;
border: 1px solid #ccc;
}
</style>
</head>
<body>
<input type="text" id="searchInput" placeholder="Search for names..">
<table id="dataTable">
<tr>
<th>Name</th>
<th>Age</th>
<th>City</th>
</tr>
<tr>
<td>John Doe</td>
<td>30</td>
<td>New York</td>
</tr>
<!-- Add more rows as needed -->
</table>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
Step 2: Implement the jQuery Filter Logic
In your JavaScript file (e.g., script.js
), use jQuery to implement the filter logic:
$(document).ready(function() {
// Function to filter the table rows based on user input
$('#searchInput').on('keyup', function() {
var value = $(this).val().toLowerCase();
$('#dataTable tr:not(:first-child)').filter(function() {
$(this).toggle($(this).text().toLowerCase().indexOf(value) > -1)
});
});
});
In this example, we use the keyup
event to detect changes in the search input field. When the user enters a search term, the toggle
function is used to show or hide rows based on whether the search term matches the content of the rows.
The :not(:first-child)
selector is used to exclude the table header row from filtering, so the header row remains visible while the data rows are filtered.
Step 3: Test the Filter Table
Open the HTML file in your browser, and you should see a table with a search input field. As you type in the input field, the table rows will be filtered dynamically based on the input text.
This is a basic example of implementing a filter table using jQuery. You can further customize the appearance and behavior of the table, add more columns, or integrate with a server-side data source for more advanced filtering and pagination. Additionally, you can explore jQuery plugins or other JavaScript libraries to enhance the filtering capabilities or add more complex features to the table.