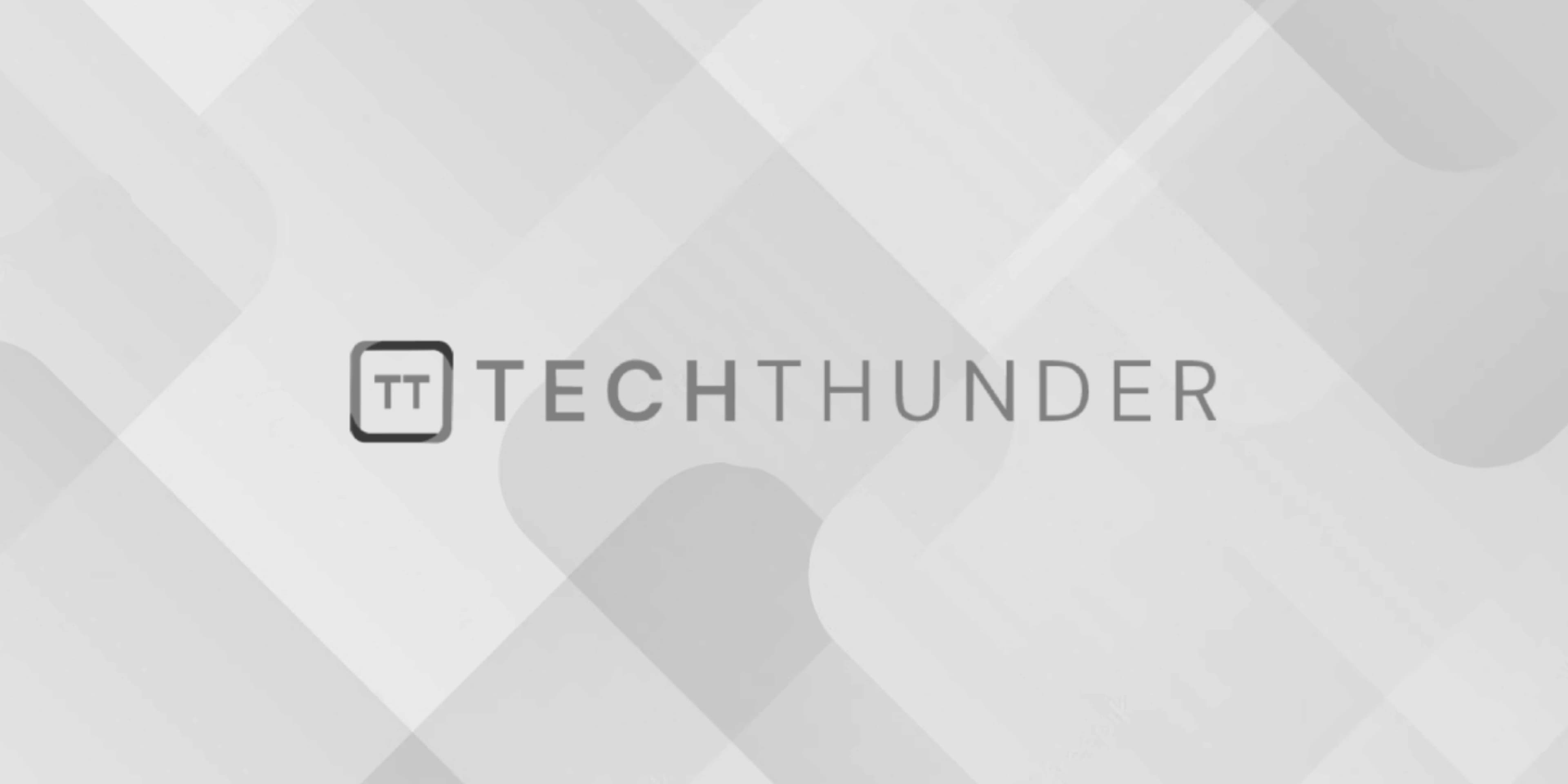
Jquery dragStop Event
As of my last knowledge update in September 2021, there is no native dragStop
event in jQuery. However, jQuery does provide a set of drag-related events that you can use to handle drag interactions with elements.
The primary drag events provided by jQuery are:
- dragstart: This event is triggered when the user starts dragging an element.
- drag: This event is triggered while the user is dragging an element.
- dragend: This event is triggered when the user finishes dragging an element.
If you want to detect the moment when the drag operation stops (equivalent to a dragStop
event), you can use the dragend
event. It will fire when the user completes the drag operation.
Here’s an example of how you can use the dragend
event in jQuery:
<!DOCTYPE html>
<html>
<head>
<title>DragStop Event Example</title>
<style>
#draggable {
width: 100px;
height: 100px;
background-color: #f00;
position: absolute;
}
</style>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
$("#draggable").on("dragend", function(event) {
console.log("Drag operation stopped.");
// Your custom code to handle drag stop goes here
});
});
</script>
</head>
<body>
<div id="draggable" draggable="true"></div>
</body>
</html>
In this example, we have a draggable <div>
element with the ID “draggable.” When the user stops dragging this element, the dragend
event is triggered, and the message “Drag operation stopped.” will be logged to the browser console.
Please note that the dragend
event will be fired even if the drag operation was canceled or if the draggable element was dropped outside of its valid drop zone. If you need to handle specific conditions related to successful drops or other interactions during dragging, you may need to implement additional logic within the dragend
event handler.