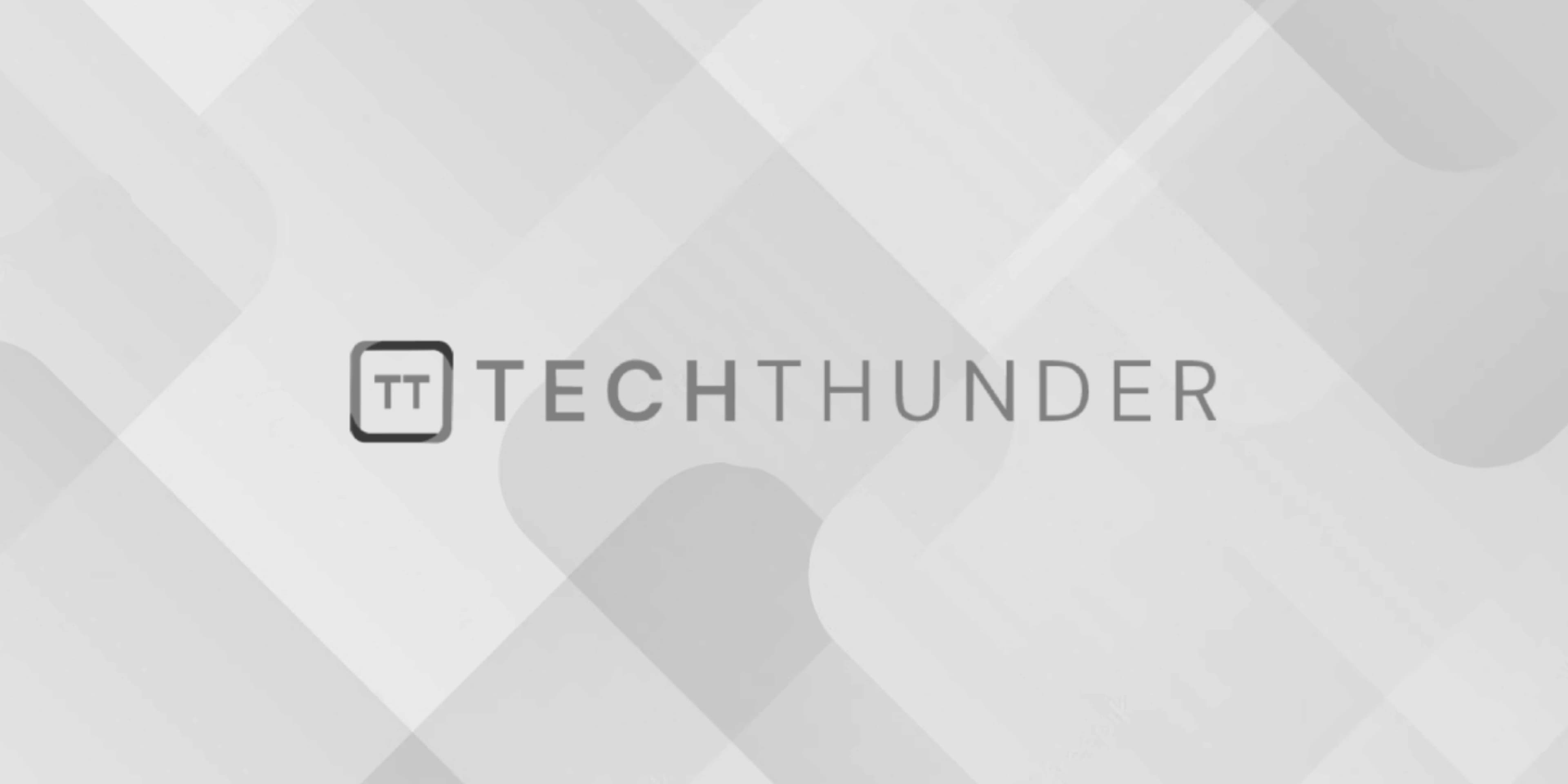
jQuery removeData() method
The jQuery.removeData()
method is used to remove previously stored data from the selected elements. It is commonly used with the jQuery.data()
method, which allows you to associate arbitrary data with DOM elements.
Here’s the basic syntax of the removeData()
method:
$(selector).removeData(key)
Parameters:
selector
: The selector for the elements from which to remove the data.key
: The key of the data to remove.
Return Value:
The removeData()
method returns the selected jQuery object to support method chaining.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery removeData() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div id="myDiv" data-info="This is some information"></div>
<script>
$(document).ready(function() {
var myDiv = $("#myDiv");
// Get the data associated with the element
var info = myDiv.data("info");
console.log("Data before removal:", info); // "This is some information"
// Remove the data associated with the element
myDiv.removeData("info");
// Try to get the data again (it should be undefined)
info = myDiv.data("info");
console.log("Data after removal:", info); // undefined
});
</script>
</body>
</html>
In this example, we have a <div>
element with the ID “myDiv” and a custom data attribute data-info
. We use the data()
method to retrieve the data associated with the element using the key “info.” The data is initially set to “This is some information.”
Next, we use the removeData()
method to remove the data associated with the “info” key from the element. After removal, if we try to get the data associated with “info” again, it will be undefined
because the data has been successfully removed.
The removeData()
method is useful when you want to remove specific data values that were previously associated with elements using the data()
method. It does not affect other attributes or properties of the elements.
Keep in mind that starting from jQuery version 1.7, the recommended way to store data associated with elements is to use the data()
method rather than using attributes like data-info
. The data()
method is more flexible, allows you to store any type of data, and is not limited to data attributes with the data-
prefix.