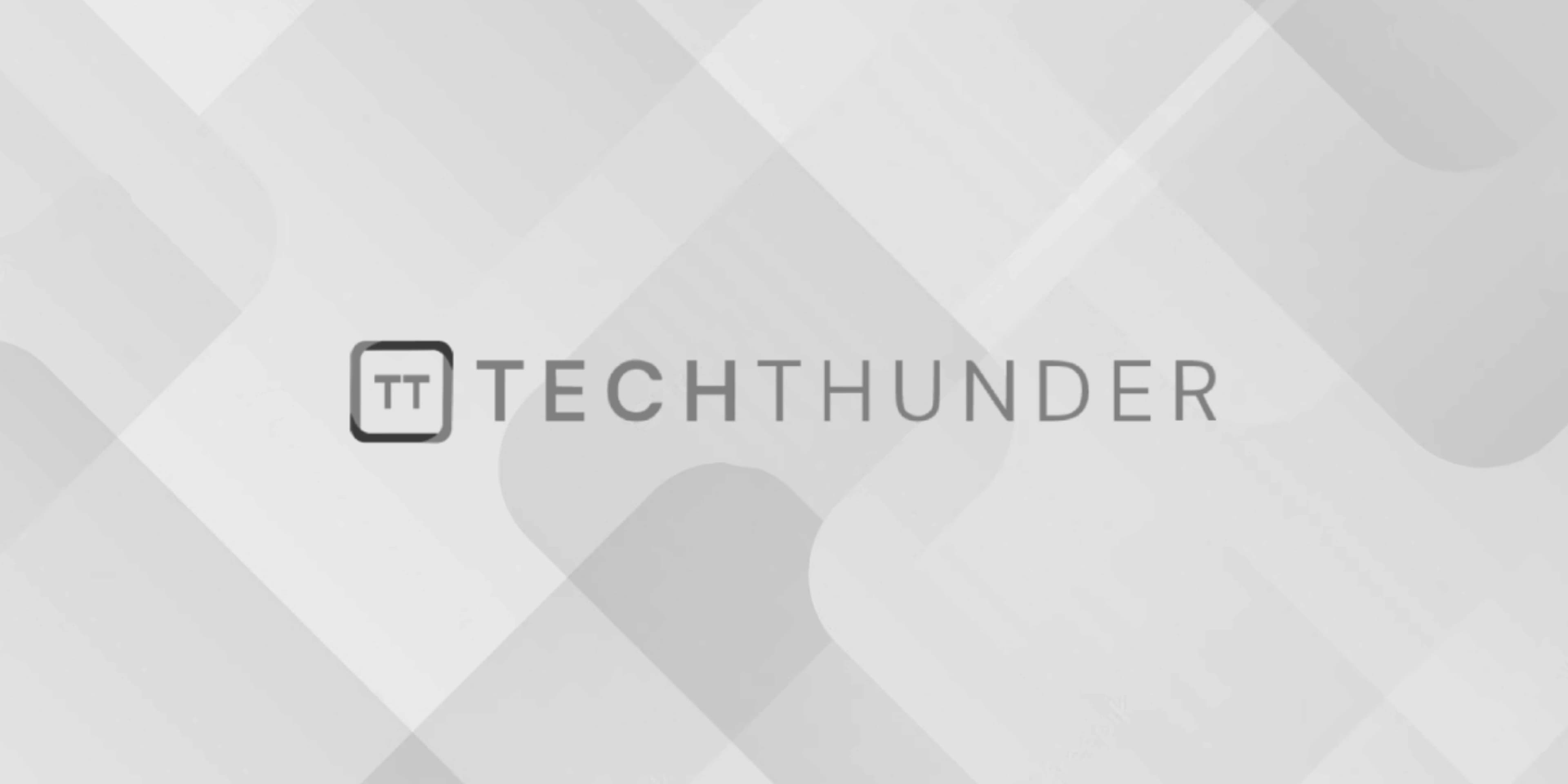
jQuery nextAll() method
The jQuery.nextAll()
method is used to select all the sibling elements that come after the selected elements in the DOM hierarchy. It allows you to target elements that are at the same level in the DOM tree and appear after the selected elements.
Here’s the basic syntax of the nextAll()
method:
$(selector).nextAll([filter])
Parameters:
selector
: Optional. A selector expression used to filter the sibling elements. If provided, only the siblings that match the selector will be selected.filter
: Optional. A selector expression used to further filter the selected siblings.
Return Value:
The nextAll()
method returns a jQuery object containing the selected sibling elements.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery nextAll() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div class="sibling">Sibling 1</div>
<div class="sibling">Sibling 2</div>
<div class="selected">Selected Element</div>
<div class="sibling">Sibling 3</div>
<div class="sibling">Sibling 4</div>
<script>
$(document).ready(function() {
// Select all sibling elements that come after the element with class "selected"
var siblingsAfter = $(".selected").nextAll();
// Log the number of selected sibling elements and their HTML content
console.log("Number of siblings after:", siblingsAfter.length);
siblingsAfter.each(function(index) {
console.log("Sibling " + index + ":", $(this).html());
});
});
</script>
</body>
</html>
In this example, we have a series of <div>
elements with the class “sibling” and one element with the class “selected.” We use the nextAll()
method to select all the sibling elements that come after the element with class “selected.” The method returns a jQuery object containing these sibling elements.
The output of the above example will be:
Number of siblings after: 2
Sibling 0: Sibling 3
Sibling 1: Sibling 4
In this output, we see that there are two sibling elements selected, which are the “Sibling 3” and “Sibling 4” elements. These elements appear after the element with class “selected” in the DOM tree.
If you provide a selector
or filter
argument to the nextAll()
method, it will only select the siblings that match the specified selector or filter. For example:
var filteredSiblingsAfter = $(".selected").nextAll(".sibling");
This will select only the siblings with class “sibling” that come after the element with class “selected” and exclude any other siblings that don’t match the selector.
The nextAll()
method is useful when you want to select sibling elements that appear after a particular element in the DOM hierarchy. It provides a way to traverse the DOM tree and select elements based on their position relative to other elements.