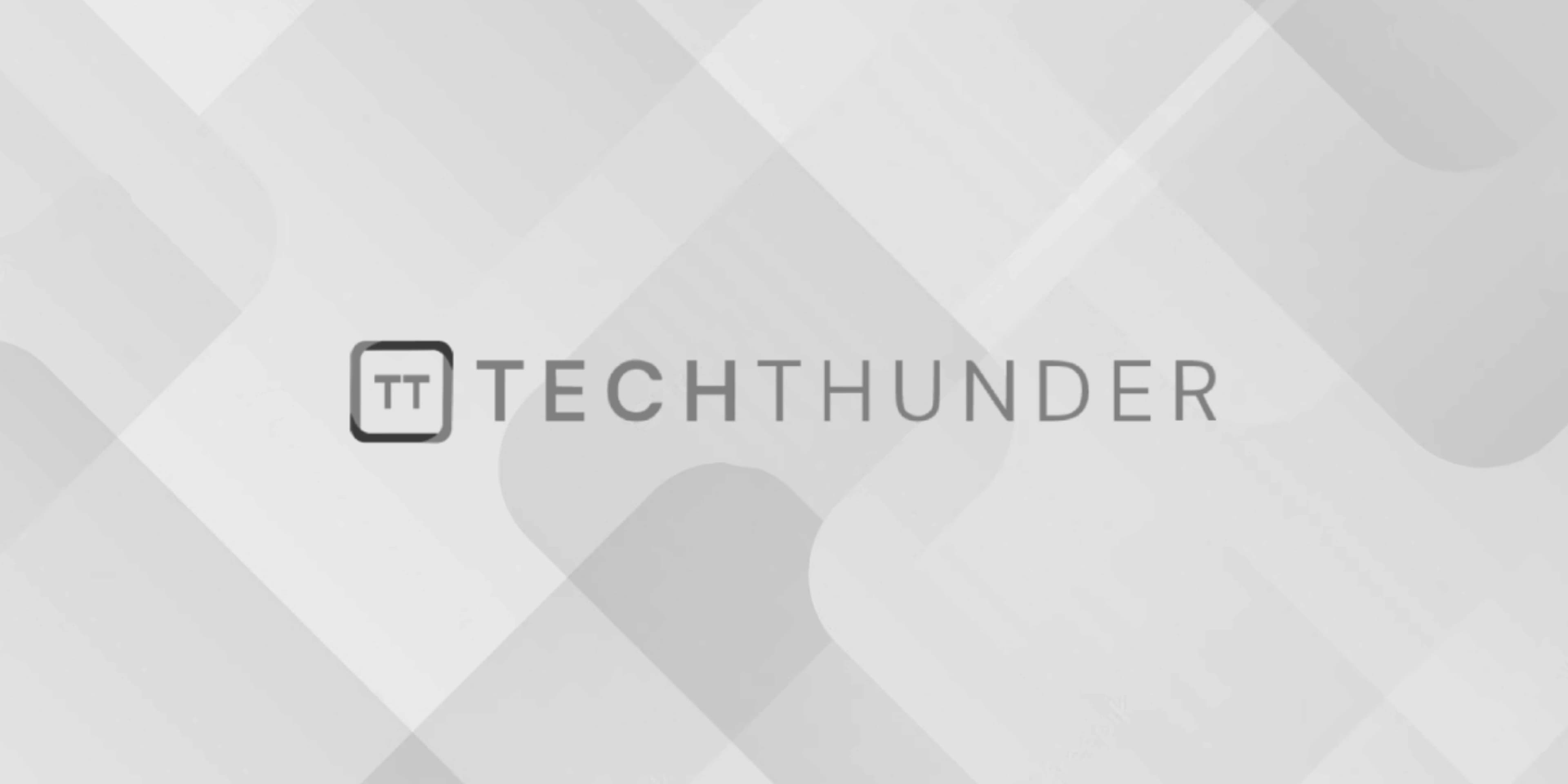
jQuery offsetParent() method
The jQuery.offsetParent()
method is used to get the closest ancestor element that is positioned (has a CSS position value other than “static”) in the DOM hierarchy.
Here’s the basic syntax of the offsetParent()
method:
$(selector).offsetParent()
Parameters:
selector
: Optional. A selector expression used to filter the elements. If provided, the method will return the closest positioned ancestor that matches the selector.
Return Value:
The offsetParent()
method returns a jQuery object containing the closest positioned ancestor element.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery offsetParent() Method Example</title>
<style>
.outer {
position: relative;
border: 2px solid red;
padding: 10px;
}
.inner {
position: absolute;
top: 20px;
left: 30px;
border: 2px solid blue;
padding: 5px;
}
</style>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div class="outer">
<div class="inner">
Some content here.
</div>
</div>
<script>
$(document).ready(function() {
var closestPositionedAncestor = $(".inner").offsetParent();
// Log the closest positioned ancestor's HTML content
console.log("Closest positioned ancestor:", closestPositionedAncestor.html());
});
</script>
</body>
</html>
In this example, we have two nested <div>
elements: “outer” and “inner.” The “outer” div is relatively positioned, and the “inner” div is absolutely positioned within it. We use the offsetParent()
method to find the closest positioned ancestor of the element with the class “inner,” which is the “outer” div.
The output of the above example will be:
Closest positioned ancestor: <div class="outer">
<div class="inner">
Some content here.
</div>
</div>
The offsetParent()
method is particularly useful when you need to find the positioned container of an absolutely positioned element. It returns the closest ancestor element that acts as a reference for the positioning of the element, taking into account any CSS positioning applied to ancestor elements.
If no positioned ancestor is found, the method will return the document root element, which is typically the <html>
element.