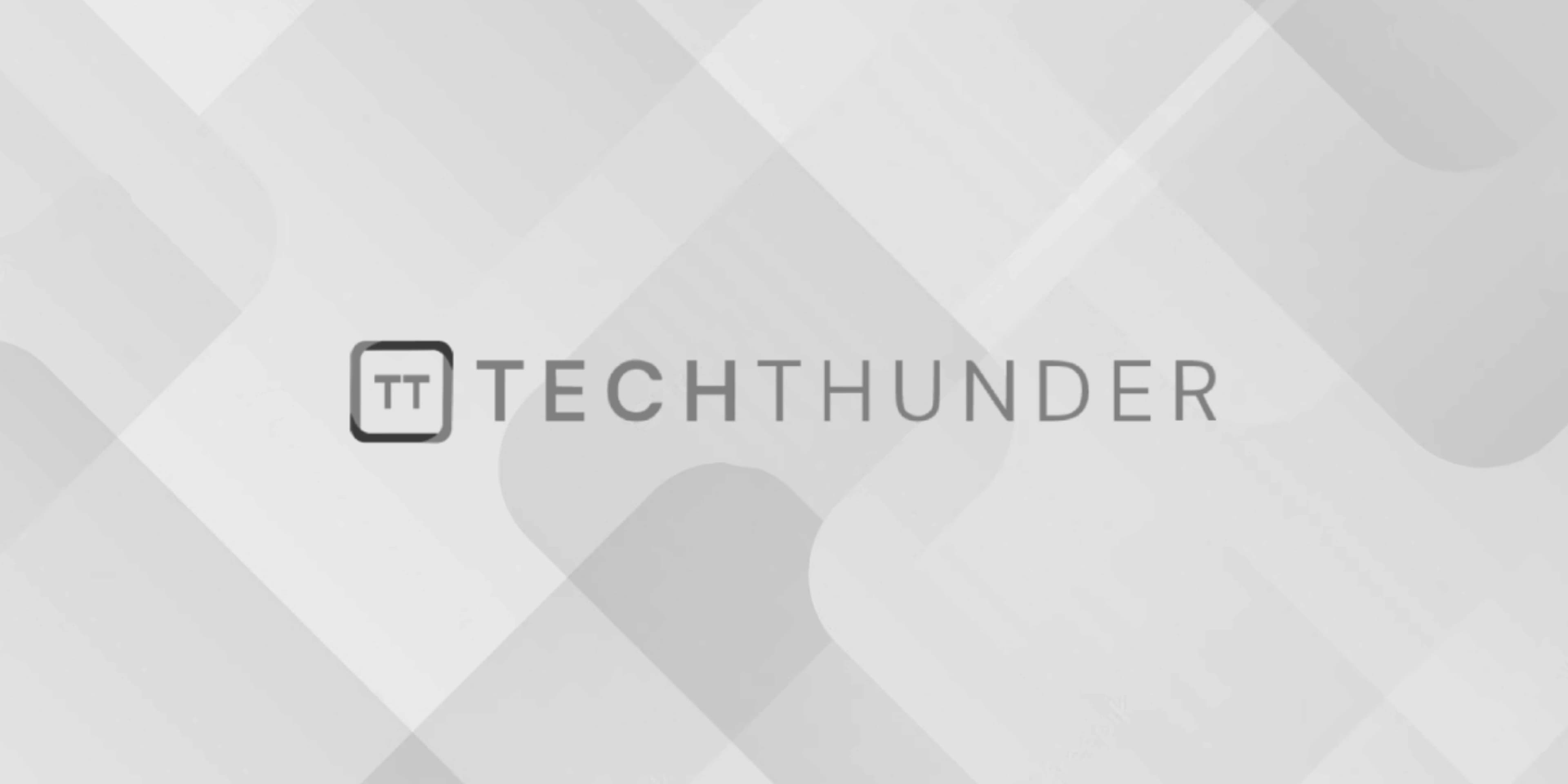
Dynamically Add Remove input fields using JQuery Ajax
To dynamically add and remove input fields using jQuery and Ajax, you can use the following steps:
Step 1: Set up your HTML structure for the form.
Step 2: Implement the “Add” functionality to add new input fields.
Step 3: Implement the “Remove” functionality to remove input fields.
Step 4: Handle data submission using Ajax (optional).
Here’s an example of how to achieve this:
Step 1: HTML structure
<!DOCTYPE html>
<html>
<head>
<title>Dynamically Add/Remove Input Fields</title>
</head>
<body>
<form id="myForm">
<div id="inputContainer">
<!-- Input fields will be dynamically added here -->
</div>
<button type="button" id="addButton">Add Field</button>
<input type="submit" value="Submit">
</form>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
Step 2: JavaScript (script.js)
$(document).ready(function() {
var maxFields = 5; // Set the maximum number of input fields
var addButton = $('#addButton');
var inputContainer = $('#inputContainer');
var fieldIndex = 1;
// Add Functionality
addButton.click(function() {
if (fieldIndex < maxFields) {
fieldIndex++;
var newField = '<div><input type="text" name="field' + fieldIndex + '"> <button type="button" class="removeButton">Remove</button></div>';
inputContainer.append(newField);
}
});
// Remove Functionality
$(document).on('click', '.removeButton', function() {
$(this).parent().remove();
fieldIndex--;
});
// Ajax Form Submission (Optional)
$('#myForm').submit(function(e) {
e.preventDefault();
var formData = $(this).serialize();
console.log(formData);
// Uncomment the following lines to handle form submission using Ajax
// $.ajax({
// type: 'POST',
// url: 'process.php', // Replace with the URL to process the form data
// data: formData,
// success: function(response) {
// console.log('Form data submitted successfully!');
// },
// error: function(error) {
// console.log('Error submitting form data:', error);
// }
// });
});
});
In this example, the JavaScript code uses jQuery to handle the “Add” and “Remove” functionalities. When the “Add Field” button is clicked, a new input field is added to the form dynamically. The “Remove” button allows users to remove any added input field. The maxFields
variable limits the maximum number of input fields that can be added.
The form submission using Ajax is optional and has been commented out in the code. You can uncomment the Ajax code to handle form data submission asynchronously to a server-side script (e.g., PHP) if needed.
With this implementation, you can dynamically add and remove input fields using jQuery and Ajax (if desired). You can further customize the form and Ajax submission based on your specific requirements.