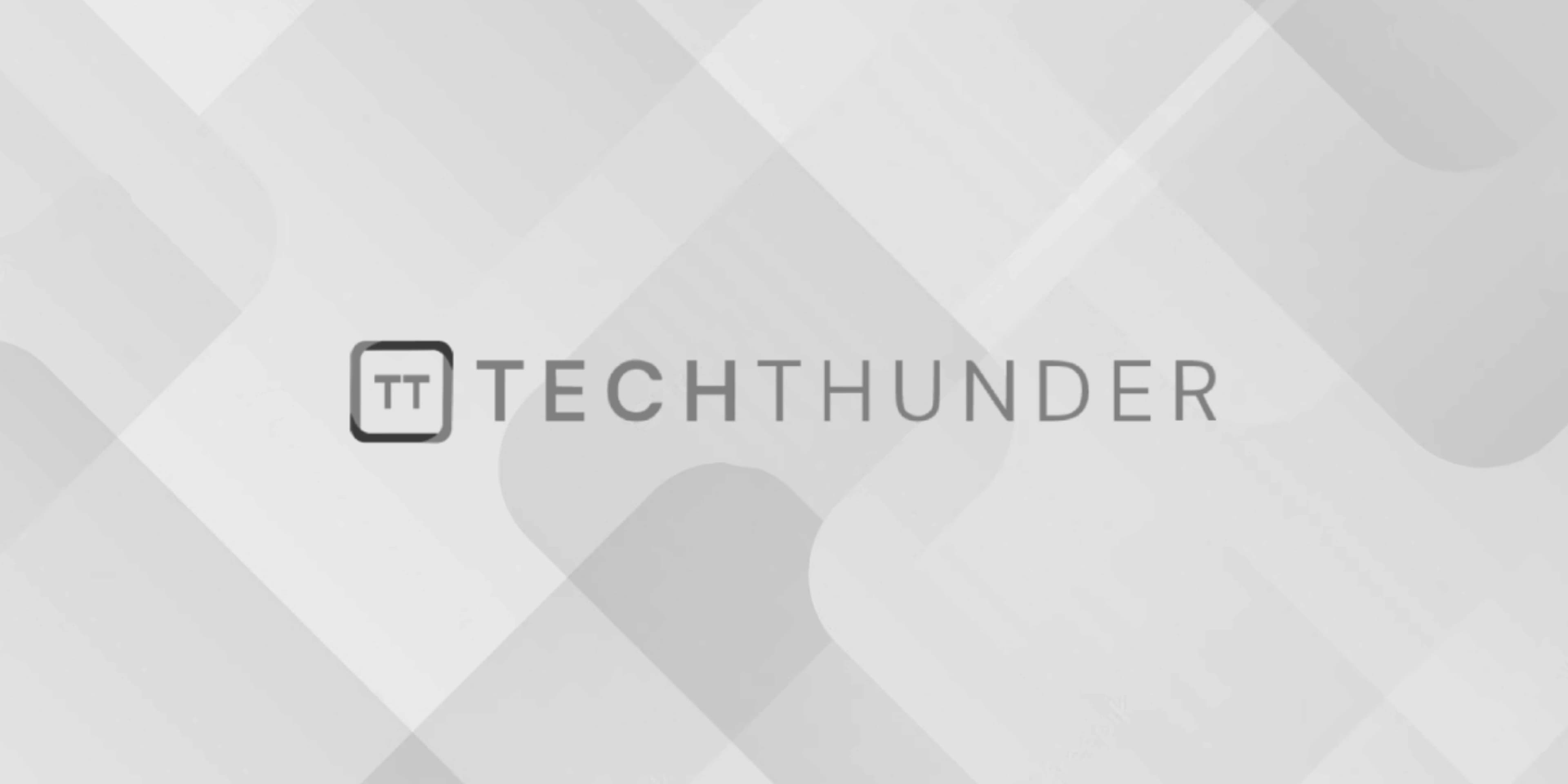
jQuery Barcode
To generate barcodes using jQuery, you can use a barcode generation library that is compatible with jQuery. One popular library for generating barcodes is “JsBarcode,” which is a pure JavaScript library that can be easily used with jQuery.
Here’s a step-by-step guide to using JsBarcode to generate barcodes with jQuery:
Step 1: Set up your HTML structure.
<!DOCTYPE html>
<html>
<head>
<title>jQuery Barcode Generator</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/@hpcc-js/[email protected]/dist/index.js"></script>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/JsBarcode.all.min.js"></script>
</head>
<body>
<div>
<input type="text" id="barcodeInput" placeholder="Enter barcode data">
<button id="generateButton">Generate Barcode</button>
</div>
<div id="barcodeOutput">
<!-- The generated barcode will be displayed here -->
</div>
<script src="script.js"></script>
</body>
</html>
Step 2: JavaScript (script.js)
$(document).ready(function() {
$('#generateButton').click(function() {
var barcodeData = $('#barcodeInput').val();
// Generate the barcode
JsBarcode('#barcodeOutput', barcodeData, {
format: 'CODE128', // Specify the barcode format (e.g., CODE128, EAN13, etc.)
displayValue: true, // Display the data below the barcode
width: 2, // Specify the barcode width (default is 2)
height: 100 // Specify the barcode height (default is 100)
// Add more options as needed
});
});
});
In this example, the JavaScript code uses jQuery to handle the click event on the “Generate Barcode” button. When the button is clicked, it reads the barcode data from the input field and uses JsBarcode to generate the barcode. The generated barcode is displayed within the #barcodeOutput
div.
The JsBarcode library supports various barcode formats like CODE128, EAN13, CODE39, and more. You can change the format
option to generate a different type of barcode.
Remember to include the necessary JavaScript files (JsBarcode and its dependencies) in the <head>
section of your HTML file, as shown in the example.
With this implementation, you can generate barcodes based on user input using jQuery and JsBarcode. You can further customize the appearance of the barcode, adjust its width and height, and explore additional options provided by the JsBarcode library to meet your specific requirements.