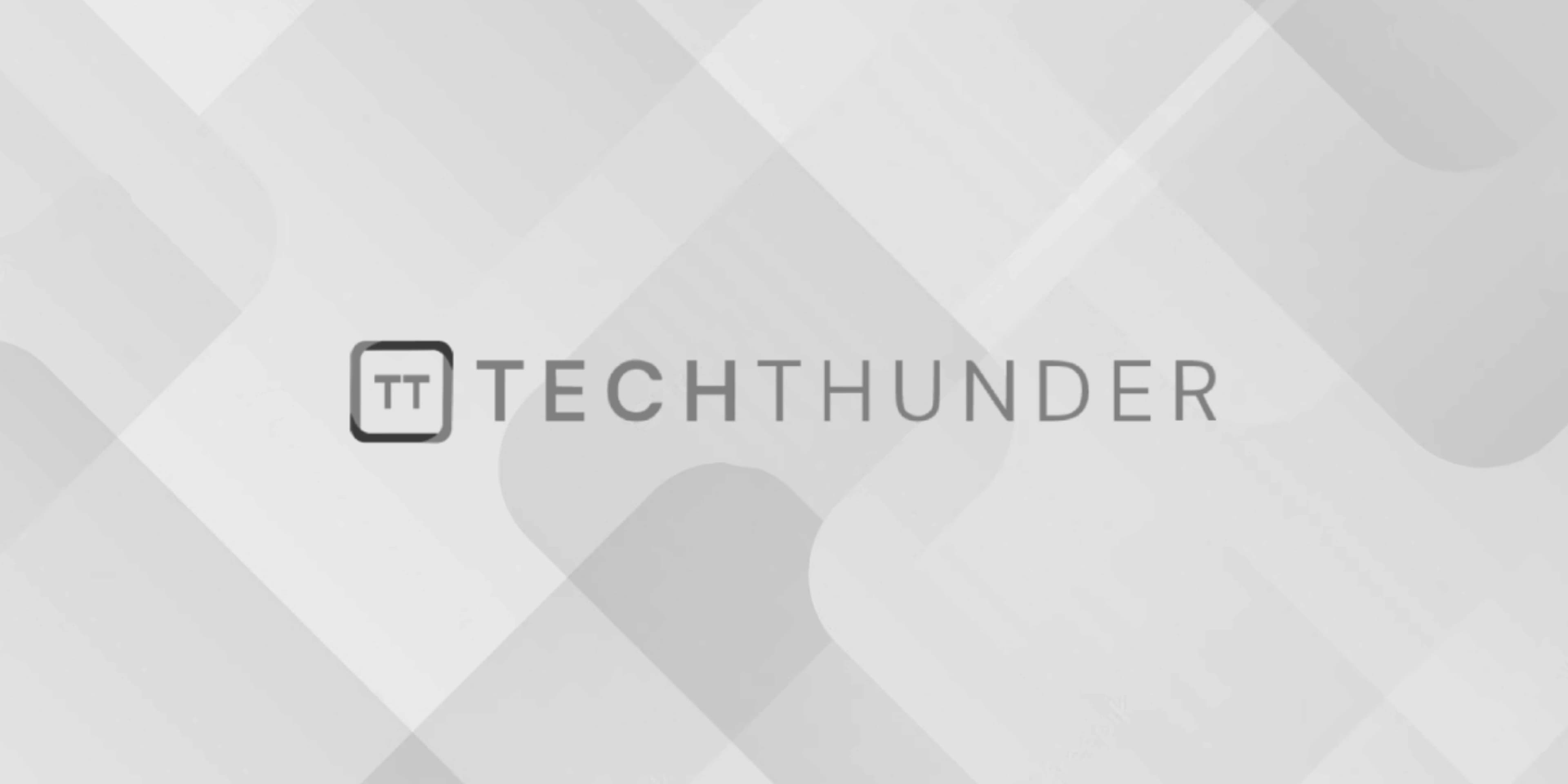
How to change the background image using jQuery
To change the background image of an element using jQuery, you can use the css()
method to modify the CSS background-image
property. Here’s a simple example to demonstrate how to change the background image of a div element:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Change Background Image using jQuery</title>
<style>
.myDiv {
width: 300px;
height: 200px;
background-size: cover;
background-position: center;
}
</style>
</head>
<body>
<div class="myDiv" id="backgroundDiv"></div>
<button id="changeButton">Change Background</button>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
JavaScript (script.js):
$(document).ready(function() {
var backgrounds = [
'url("image1.jpg")',
'url("image2.jpg")',
'url("image3.jpg")'
// Add more image URLs as needed
];
var currentBackground = 0;
var backgroundDiv = $('#backgroundDiv');
// Function to change the background image
function changeBackground() {
backgroundDiv.css('background-image', backgrounds[currentBackground]);
currentBackground = (currentBackground + 1) % backgrounds.length;
}
// Initial background image
changeBackground();
// Button click event to change the background image
$('#changeButton').click(function() {
changeBackground();
});
});
In this example, we have a div
element with the class .myDiv
, and we use jQuery to handle the background image change. We set up an array backgrounds
containing the URLs of the images we want to use as background images. The currentBackground
variable keeps track of the currently displayed image index, and the backgroundDiv
variable represents the div
element where we want to change the background image.
The changeBackground()
function changes the background image of the backgroundDiv
element by modifying the background-image
property using the css()
method. It cycles through the backgrounds
array to show different images each time it’s called.
When the page loads, the initial background image is set using the first image from the backgrounds
array. When the “Change Background” button is clicked, the background image changes to the next one in the array.
You can add as many images as you want to the backgrounds
array to have a larger variety of background images.