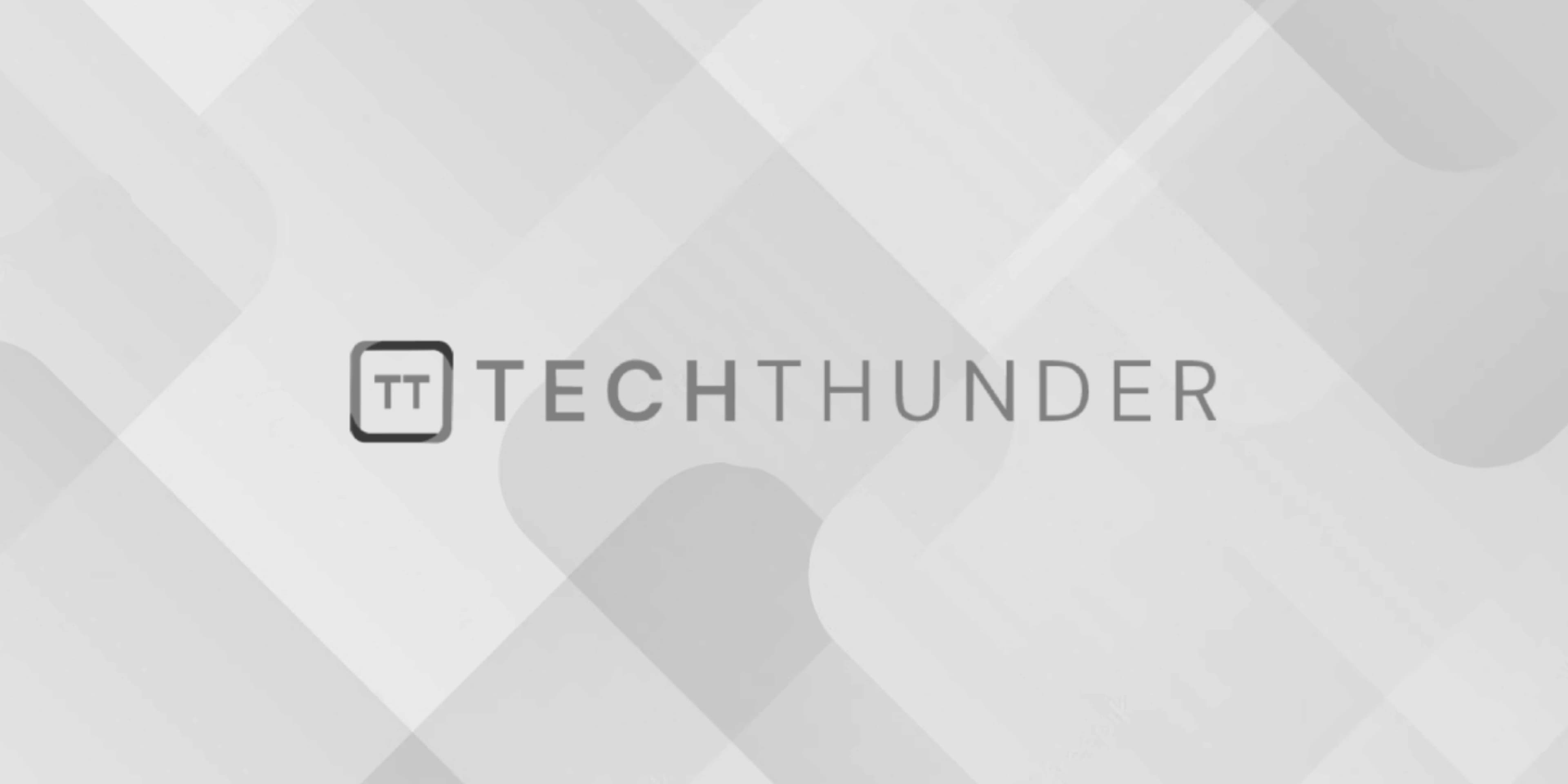
JQuery Calendar
To create a simple calendar using jQuery, you can utilize HTML, CSS, and JavaScript/jQuery. We will use the jQuery UI Datepicker, which is a popular plugin that provides a user-friendly date selection interface.
Here’s a step-by-step guide to creating a basic jQuery calendar:
Step 1: Set up the HTML structure:
<!DOCTYPE html>
<html>
<head>
<title>jQuery Calendar</title>
<link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
</head>
<body>
<label for="datepicker">Select a date:</label>
<input type="text" id="datepicker">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<script src="script.js"></script>
</body>
</html>
Step 2: Initialize the Datepicker in the JavaScript (script.js):
$(document).ready(function() {
// Initialize Datepicker
$("#datepicker").datepicker();
});
In this example, we have included the necessary CSS and JavaScript files for jQuery and jQuery UI Datepicker. We create a text input with the ID datepicker
, and the “Select a date” label associates with it.
When the document is ready, we initialize the Datepicker by calling $("#datepicker").datepicker();
. This will transform the #datepicker
input into a date picker.
Step 3: Styling (Optional):
You can customize the appearance of the calendar by adding custom CSS styles. For instance, you can define your custom theme, change colors, adjust fonts, etc. The appearance is highly customizable to match the overall look and feel of your website.
Your final project structure might look like this:
- index.html
- script.js
- styles.css
In this example, you have a basic jQuery calendar set up using the jQuery UI Datepicker. As you select a date from the calendar, the value will be populated in the associated input field.
Remember to check the official documentation for the jQuery UI Datepicker (https://jqueryui.com/datepicker/) to explore all the available options and customization possibilities.