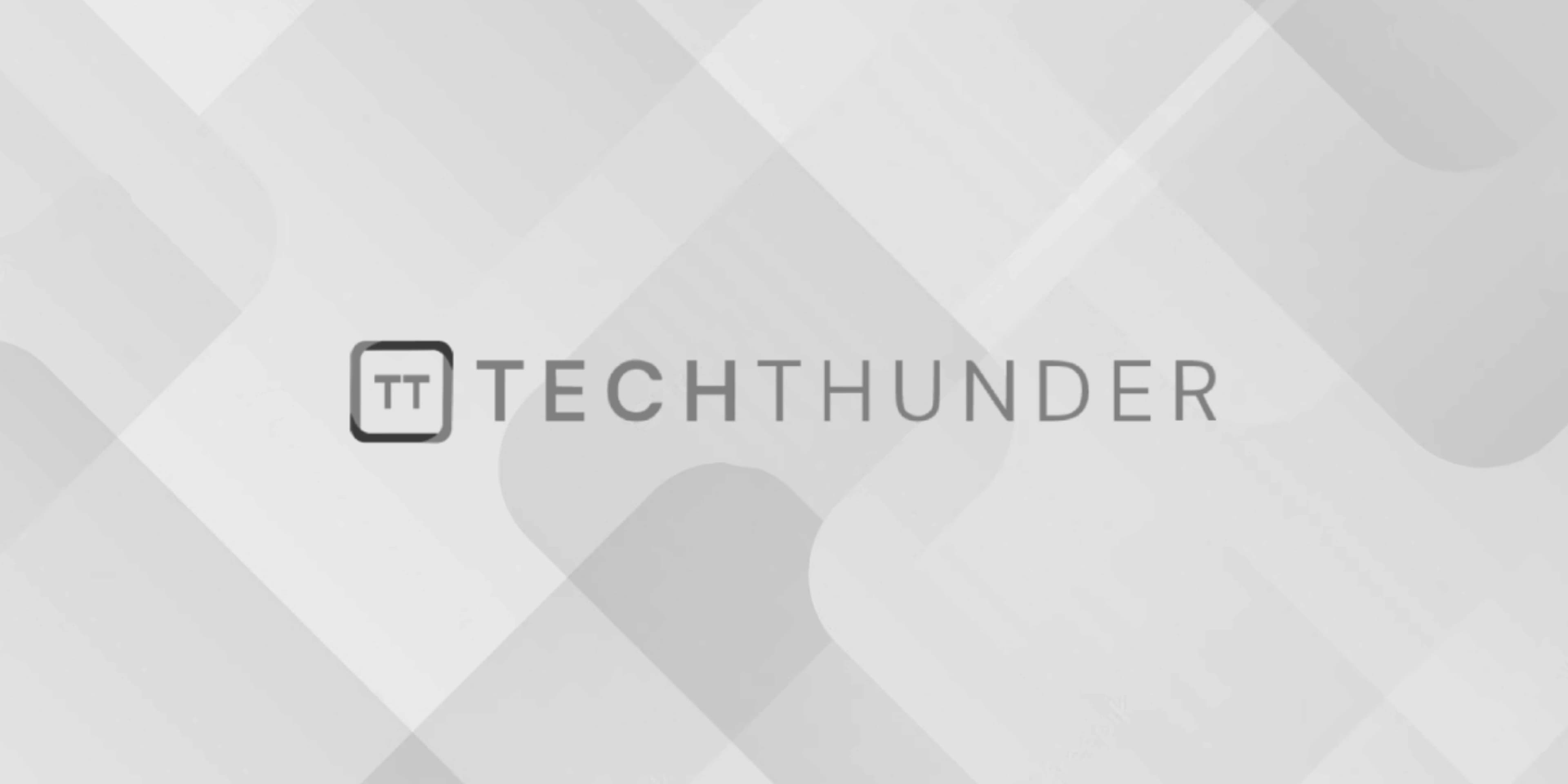
Create To-Do list using JQuery
To create a simple To-Do list using jQuery, you can follow these steps:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>To-Do List</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="todo-container">
<h1>To-Do List</h1>
<input type="text" id="taskInput" placeholder="Add new task...">
<button id="addButton">Add</button>
<ul id="taskList"></ul>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
CSS (styles.css):
body {
font-family: Arial, sans-serif;
text-align: center;
}
.todo-container {
max-width: 400px;
margin: 50px auto;
padding: 20px;
border: 2px solid #ccc;
border-radius: 8px;
}
h1 {
margin-top: 0;
}
input[type="text"] {
width: 70%;
padding: 8px;
border: 1px solid #ccc;
border-radius: 4px;
margin-right: 10px;
}
button {
padding: 8px 16px;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 4px;
cursor: pointer;
}
ul {
list-style: none;
padding: 0;
}
li {
padding: 8px 0;
border-bottom: 1px solid #ccc;
}
li:last-child {
border-bottom: none;
}
JavaScript (script.js):
$(document).ready(function() {
// Add button click event
$('#addButton').on('click', function() {
const taskInput = $('#taskInput').val().trim();
if (taskInput !== '') {
addTask(taskInput);
$('#taskInput').val('');
}
});
// Enter key press event
$('#taskInput').on('keypress', function(event) {
if (event.which === 13) {
const taskInput = $(this).val().trim();
if (taskInput !== '') {
addTask(taskInput);
$(this).val('');
}
}
});
// Task completion event
$(document).on('click', '.task', function() {
$(this).toggleClass('completed');
});
// Task deletion event
$(document).on('click', '.delete', function() {
$(this).parent().remove();
});
function addTask(taskInput) {
const newTask = $('<li class="task"></li>').text(taskInput);
const deleteButton = $('<span class="delete">X</span>');
newTask.append(deleteButton);
$('#taskList').append(newTask);
}
});
In this example, we create a simple To-Do list with an input field to add new tasks and a list to display the tasks. When you type a task in the input field and click the “Add” button or press the “Enter” key, the task will be added to the list. You can mark a task as completed by clicking on it, and you can delete a task by clicking the “X” button next to it.
The JavaScript/jQuery code handles adding tasks, marking them as completed, and deleting tasks from the list. The CSS styles the To-Do list to make it visually appealing.
With this basic structure, you can expand the To-Do list with additional features like saving tasks to local storage, editing tasks, or setting due dates.