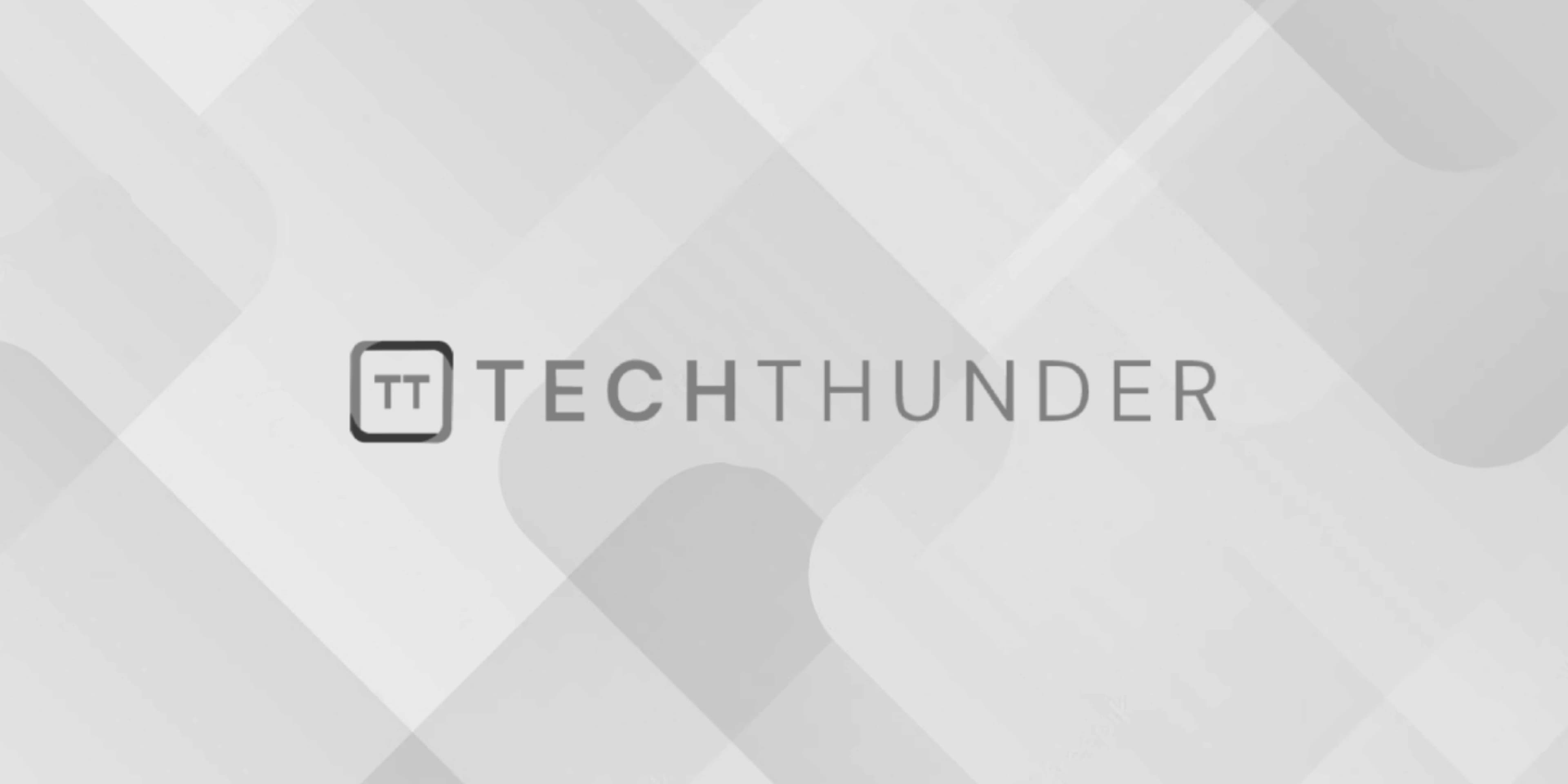
JQuery Pagination
To implement pagination using jQuery, you can create a function that displays a limited number of items per page and provides navigation controls to switch between pages. Here’s a step-by-step guide to create a basic jQuery pagination:
Step 1: Set up your HTML structure.
<!DOCTYPE html>
<html>
<head>
<title>jQuery Pagination</title>
<style>
.item {
display: none;
}
.active {
display: block;
}
.pagination {
margin-top: 10px;
}
.pagination a {
margin: 0 5px;
cursor: pointer;
}
.pagination .active-page {
font-weight: bold;
}
</style>
</head>
<body>
<div class="items">
<div class="item">Item 1</div>
<div class="item">Item 2</div>
<div class="item">Item 3</div>
<div class="item">Item 4</div>
<!-- Add more items as needed -->
</div>
<div class="pagination">
<!-- Pagination links will be dynamically added here -->
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
Step 2: JavaScript (script.js)
$(document).ready(function() {
var itemsPerPage = 2; // Set the number of items to display per page
var $items = $(".items .item");
var numItems = $items.length;
var numPages = Math.ceil(numItems / itemsPerPage);
// Display the first page
showPage(1);
// Create pagination links
var paginationHTML = '';
for (var i = 1; i <= numPages; i++) {
paginationHTML += '<a href="#" data-page="' + i + '">' + i + '</a>';
}
$(".pagination").html(paginationHTML);
// Handle pagination link clicks
$(".pagination a").click(function(e) {
e.preventDefault();
var page = $(this).data("page");
showPage(page);
});
// Function to show a specific page
function showPage(page) {
var startIndex = (page - 1) * itemsPerPage;
var endIndex = Math.min(startIndex + itemsPerPage, numItems);
$items.hide().slice(startIndex, endIndex).addClass("active");
$(".pagination a").removeClass("active-page");
$(".pagination a[data-page='" + page + "']").addClass("active-page");
}
});
In this example, the JavaScript code sets up the pagination functionality. It calculates the total number of pages based on the number of items and the items per page. The function showPage(page)
displays the specified page by showing the relevant items and updating the active pagination link. The pagination links are generated dynamically based on the number of pages.
With this implementation, you have a basic jQuery pagination setup. You can further customize the styles and the number of items per page as per your requirements. Additionally, you can load the items from an external data source using Ajax and update the pagination accordingly.