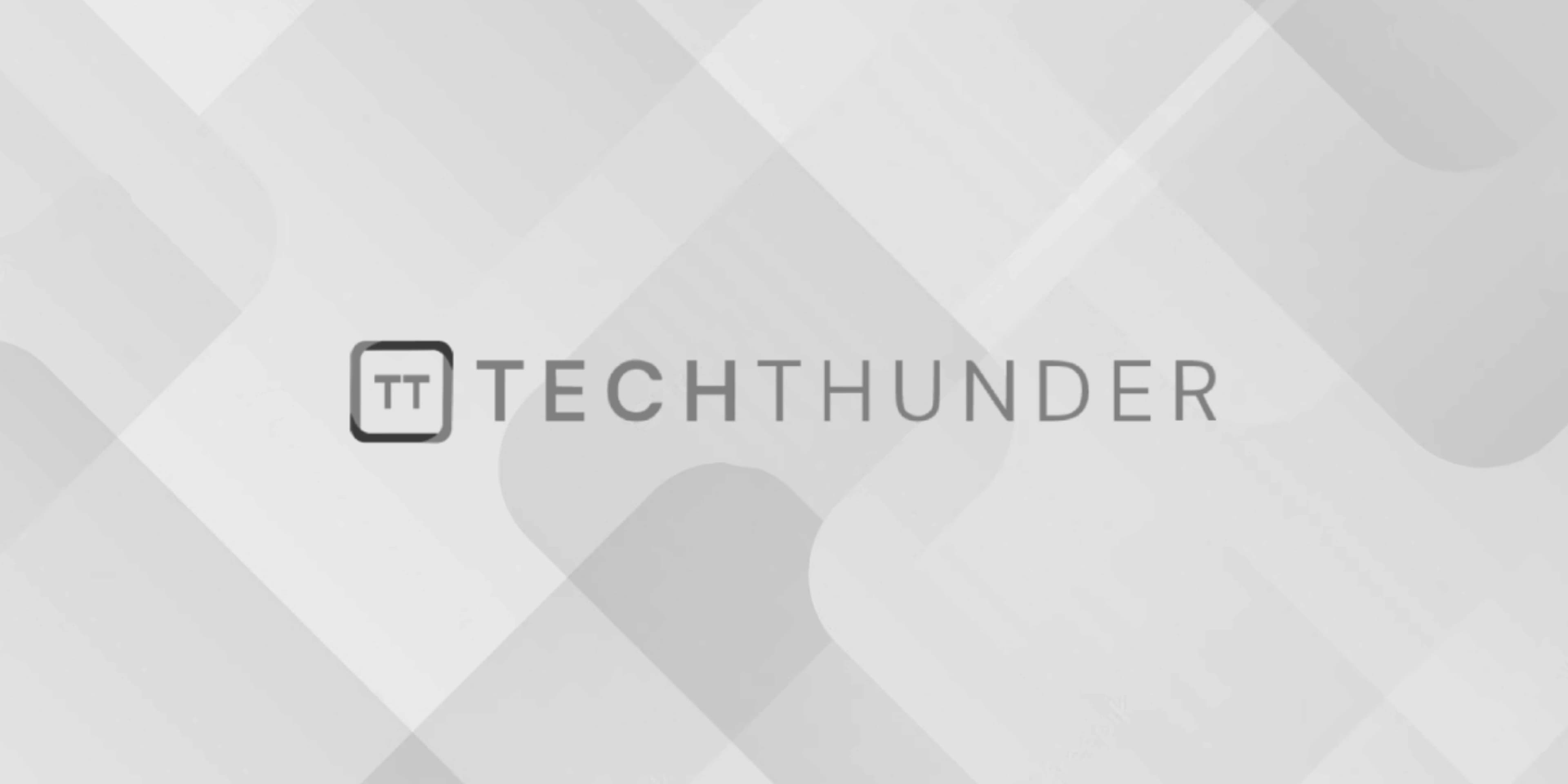
jQuery end() method
The .end()
method in jQuery is used to revert the most recent filtering operation and return the set of matched elements to its previous state. It effectively “closes” the most recent chain of method calls and restores the previously selected elements. This is particularly useful when you want to apply multiple filtering or traversal operations on a set of elements but need to revert to a previous state.
Here’s a simple example to illustrate how the .end()
method works:
<!DOCTYPE html>
<html>
<head>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<script>
$(document).ready(function() {
$("li")
.css("color", "blue")
.end() // Reverts to the previous state
.css("font-weight", "bold");
});
</script>
</body>
</html>
In this example:
- We select all the
<li>
elements and change their text color to blue using.css("color", "blue")
. - We then use the
.end()
method, which reverts the selection to the previous state (before the color change). - After calling
.end()
, we apply another operation to the same set of elements, setting their font weight to “bold” using.css("font-weight", "bold")
.
As a result, the elements will have their font weight set to “bold,” but their text color will remain the default color since the .end()
method reverted the chain of method calls to its previous state.
This is just a basic example, and the .end()
method can be more useful in complex scenarios when you have multiple filtering or traversal operations and need to step back to a previous state in the chain of operations.