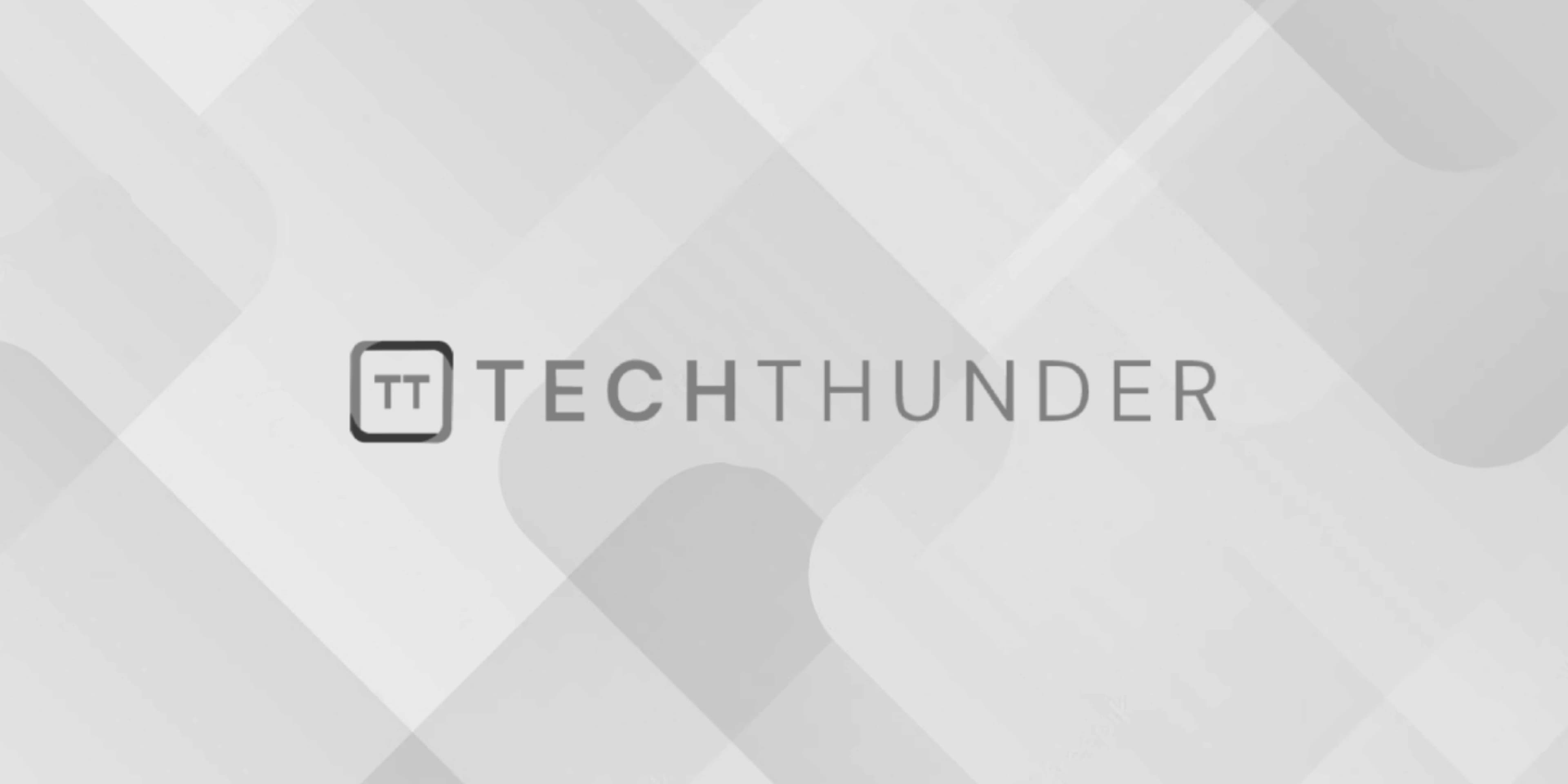
jQuery index() method
The jQuery index()
method is used to get the index of an element within its parent’s collection of child elements. It is often used to find the position of an element relative to its siblings.
Here’s the basic syntax of the index()
method:
$(selector).index()
Parameters:
selector
: A selector expression used to select the element whose index you want to retrieve. If not provided, the method returns the index of the first selected element in the collection.
Return Value:
The index()
method returns an integer value representing the index of the selected element within its parent’s collection of child elements. The index is zero-based, meaning the first element has an index of 0, the second element has an index of 1, and so on.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery index() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li class="selected">Item 3 (Selected)</li>
<li>Item 4</li>
<li>Item 5</li>
</ul>
<script>
$(document).ready(function() {
// Get the index of the selected item within its parent (zero-based index)
var selectedIndex = $("li.selected").index();
// Log the index of the selected item
console.log("Index of the selected item: " + selectedIndex);
});
</script>
</body>
</html>
In this example, we have an unordered list (<ul>
) containing several list items (<li>
). One of the list items has the class “selected” to indicate that it is the selected item. We use the index()
method to get the index of the selected item within its parent (<ul>
). Since the selected item is the third child, its index will be 2 (zero-based index).
When you run the code and check the console, you will see the following output:
Index of the selected item: 2
The index()
method is useful when you need to find the position of an element within a collection of elements. It is often used in combination with other jQuery methods to perform various DOM manipulation and traversal tasks.