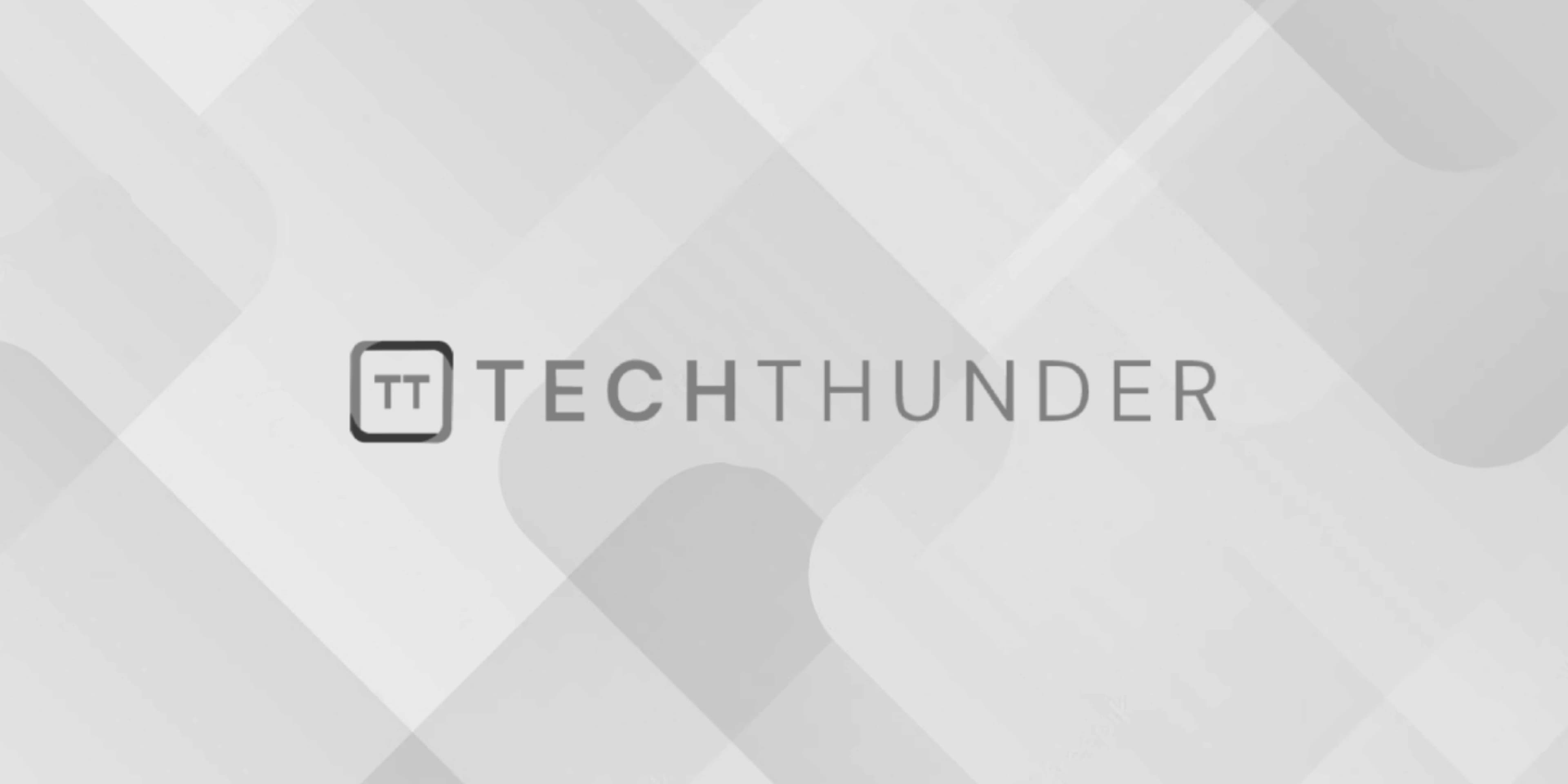
jQuery :file selector
The :file
selector in jQuery is used to select input elements with the type="file"
. It allows you to target file input elements in your HTML forms. This selector is helpful when you want to perform actions specifically on file input elements, such as handling file uploads.
Here’s the basic syntax of the :file
selector:
$(":file")
Return Value:
The :file
selector returns a jQuery object containing all file input elements found in the document.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery :file Selector Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<form>
<label for="fileInput">Select a file:</label>
<input type="file" id="fileInput">
<button id="uploadButton">Upload</button>
</form>
<script>
$(document).ready(function() {
// Add a click event handler to the upload button
$("#uploadButton").click(function() {
// Get the selected file name from the file input
var fileName = $("#fileInput").val();
alert("Selected file: " + fileName);
});
});
</script>
</body>
</html>
In this example, we have a simple HTML form with a file input element and an upload button. When the upload button is clicked, the click
event handler function is executed. Inside the function, we use the :file
selector to select the file input element and get the value of the selected file using the val()
method. We then display an alert with the selected file name.
Keep in mind that the :file
selector is just one of many useful selectors provided by jQuery. It allows you to perform actions on file input elements easily. Additionally, jQuery selectors can be combined with other selectors to target specific elements more precisely in your web page.