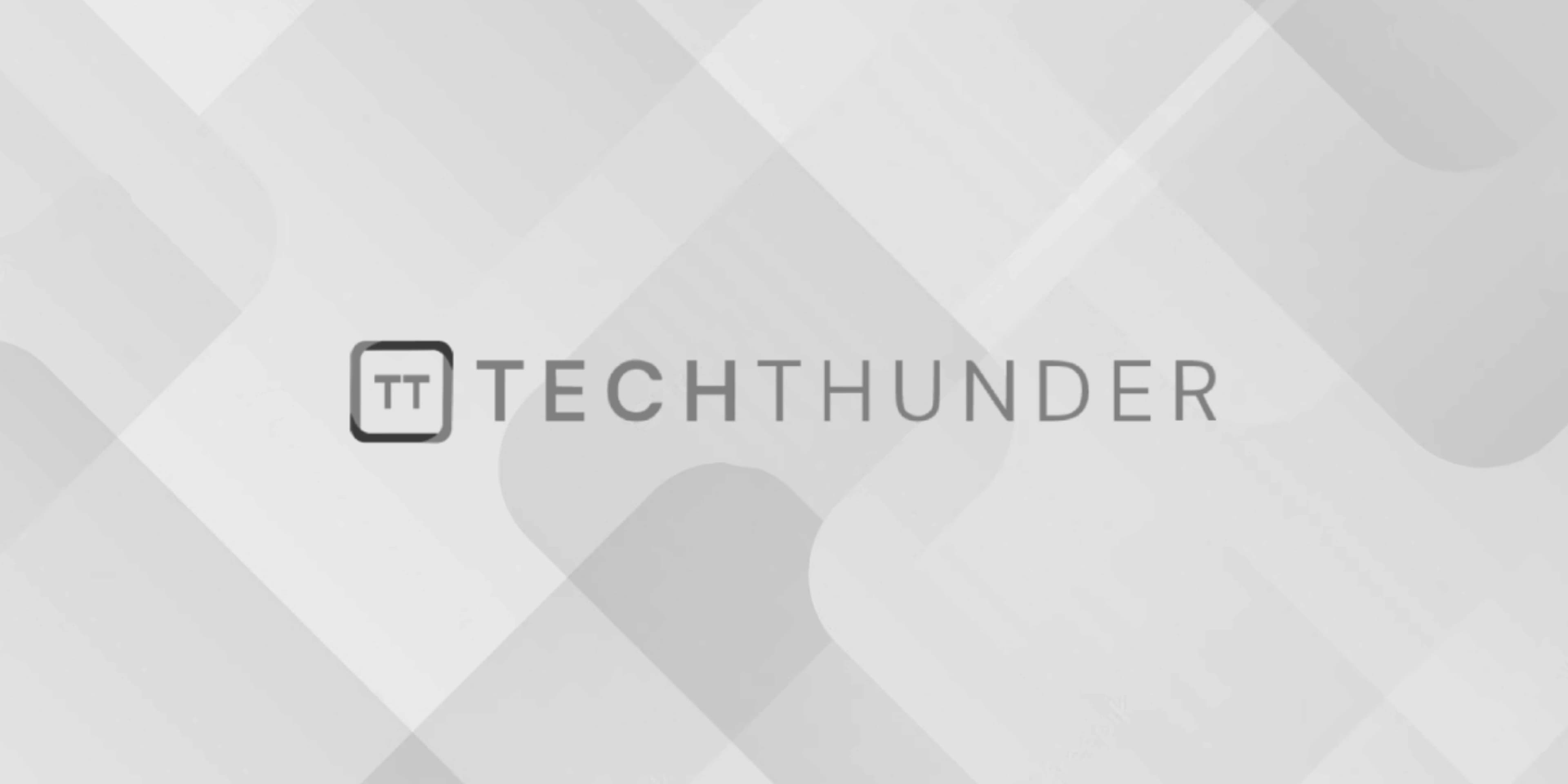
2D Array in C
A two-dimensional (2D) array in C is essentially an array of arrays. It’s a data structure that stores elements in a table or grid format with rows and columns. Each element in a 2D array is identified by two indices: a row index and a column index. To declare, initialize, and access elements in a 2D array in C, you need to follow specific syntax and conventions.
Here’s how to work with 2D arrays in C:
Declaration:
To declare a 2D array, you specify the data type of its elements, the array name, and the dimensions (number of rows and columns) enclosed in square brackets [][]
.
data_type array_name[rows][columns];
For example:
int matrix[3][3]; // Declares a 3x3 integer matrix
Initialization:
You can initialize a 2D array when declaring it, or you can initialize individual elements later in your code.
int matrix[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
Accessing Elements:
To access elements in a 2D array, you use two indices: one for the row and one for the column.
int value = matrix[row_index][column_index];
Remember that C uses zero-based indexing, so the first row and first column have index 0.
Iterating Through a 2D Array:
You can use nested loops (a loop within another loop) to iterate through the elements of a 2D array. This is commonly done using for
loops.
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) {
// Access and process matrix[i][j]
}
}
Dynamic Allocation:
If you need to create a 2D array with dimensions determined at runtime, you can use dynamic memory allocation with pointers. This involves allocating memory for both rows and columns separately.
Here’s an example of dynamically allocating a 2D array:
int rows = 3;
int columns = 3;
int **matrix = (int **)malloc(rows * sizeof(int *));
for (int i = 0; i < rows; i++) {
matrix[i] = (int *)malloc(columns * sizeof(int));
}
// Initialize and use the dynamically allocated matrix
// Don't forget to free the memory when you're done
for (int i = 0; i < rows; i++) {
free(matrix[i]);
}
free(matrix);
Make sure to free the memory when you’re done with the dynamically allocated 2D array to prevent memory leaks.
2D arrays are commonly used in C for tasks such as representing matrices, grids, tables, and more. They provide a structured way to organize and manipulate data in rows and columns.