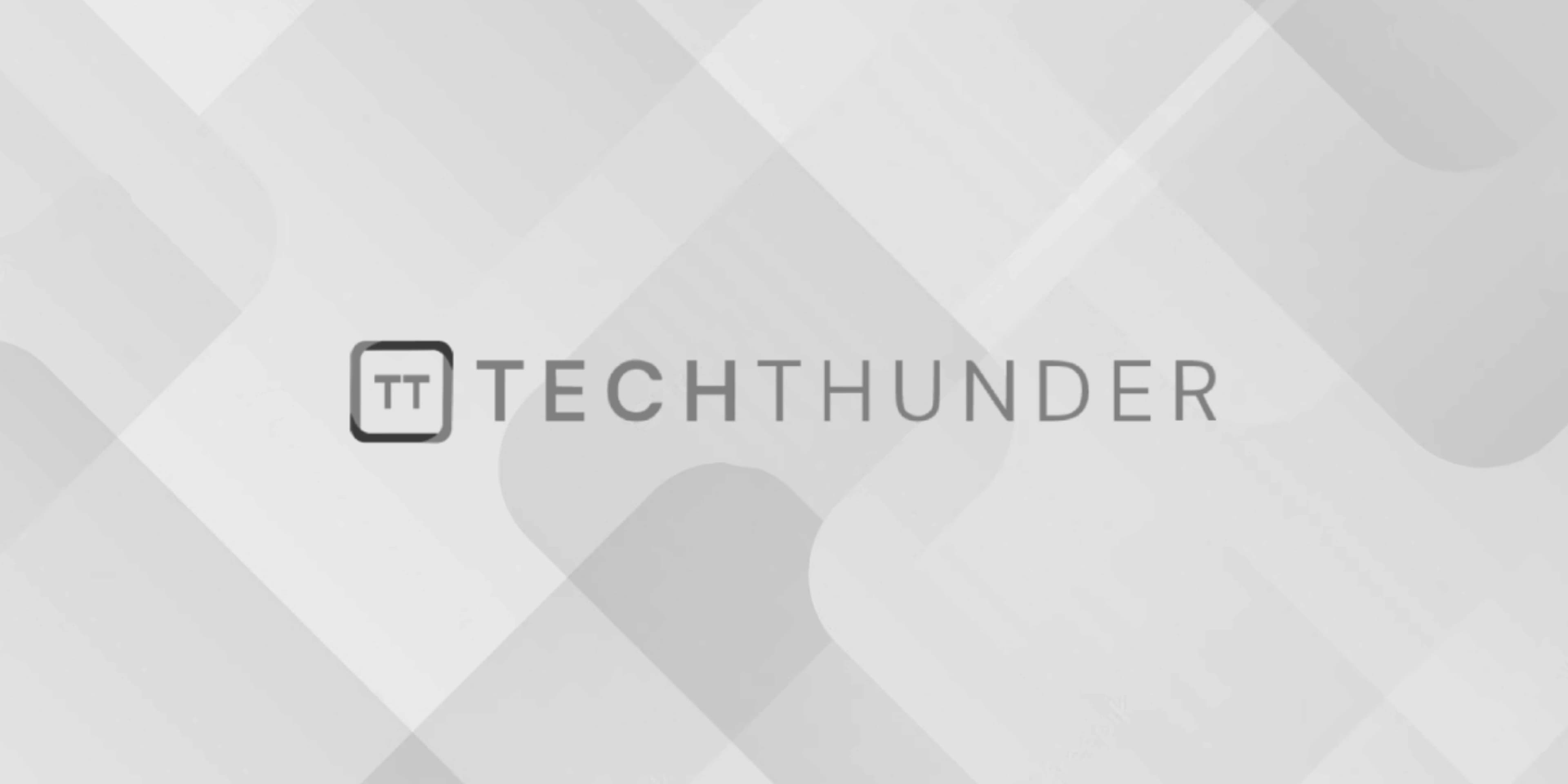
Malloc in C
The malloc()
function is used to dynamically allocate memory on the heap (also known as the free store) during program execution. This memory allocation is done at runtime and is not determined at compile-time, allowing you to work with data structures of variable size. The malloc()
function is part of the C Standard Library and is declared in the <stdlib.h>
header.
Here is the syntax of the malloc()
function:
void *malloc(size_t size);
size
: The number of bytes of memory to allocate. This determines the size of the memory block you want to allocate.
The malloc()
function returns a pointer to the first byte of the allocated memory block, or it returns a null pointer (NULL
) if the allocation fails (e.g., due to insufficient memory).
Here’s an example of how to use malloc()
to allocate memory for an array of integers:
#include <stdio.h>
#include <stdlib.h>
int main() {
int *arr;
int size = 5;
// Allocate memory for an array of integers
arr = (int *)malloc(size * sizeof(int));
if (arr == NULL) {
printf("Memory allocation failed.\n");
return 1; // Exit with an error code
}
// Initialize the array
for (int i = 0; i < size; i++) {
arr[i] = i * 2;
}
// Access and print the elements of the array
for (int i = 0; i < size; i++) {
printf("arr[%d] = %d\n", i, arr[i]);
}
// Deallocate the allocated memory
free(arr);
return 0;
}
In this example:
- We declare a pointer
arr
to store the address of the dynamically allocated memory. - We use
malloc(size * sizeof(int))
to allocate memory for an array ofsize
integers. Thesizeof(int)
is used to ensure that we allocate enough memory to storesize
integers. - We check if
malloc()
returns a null pointer (NULL
) to handle cases where memory allocation fails (e.g., due to insufficient memory). - We initialize the array by setting its elements to some values.
- We access and print the elements of the array.
- Finally, we use the
free()
function to deallocate the dynamically allocated memory when it’s no longer needed. Failure to deallocate memory can lead to memory leaks.
It’s important to remember to free dynamically allocated memory when you’re done using it to prevent memory leaks and ensure efficient memory management.