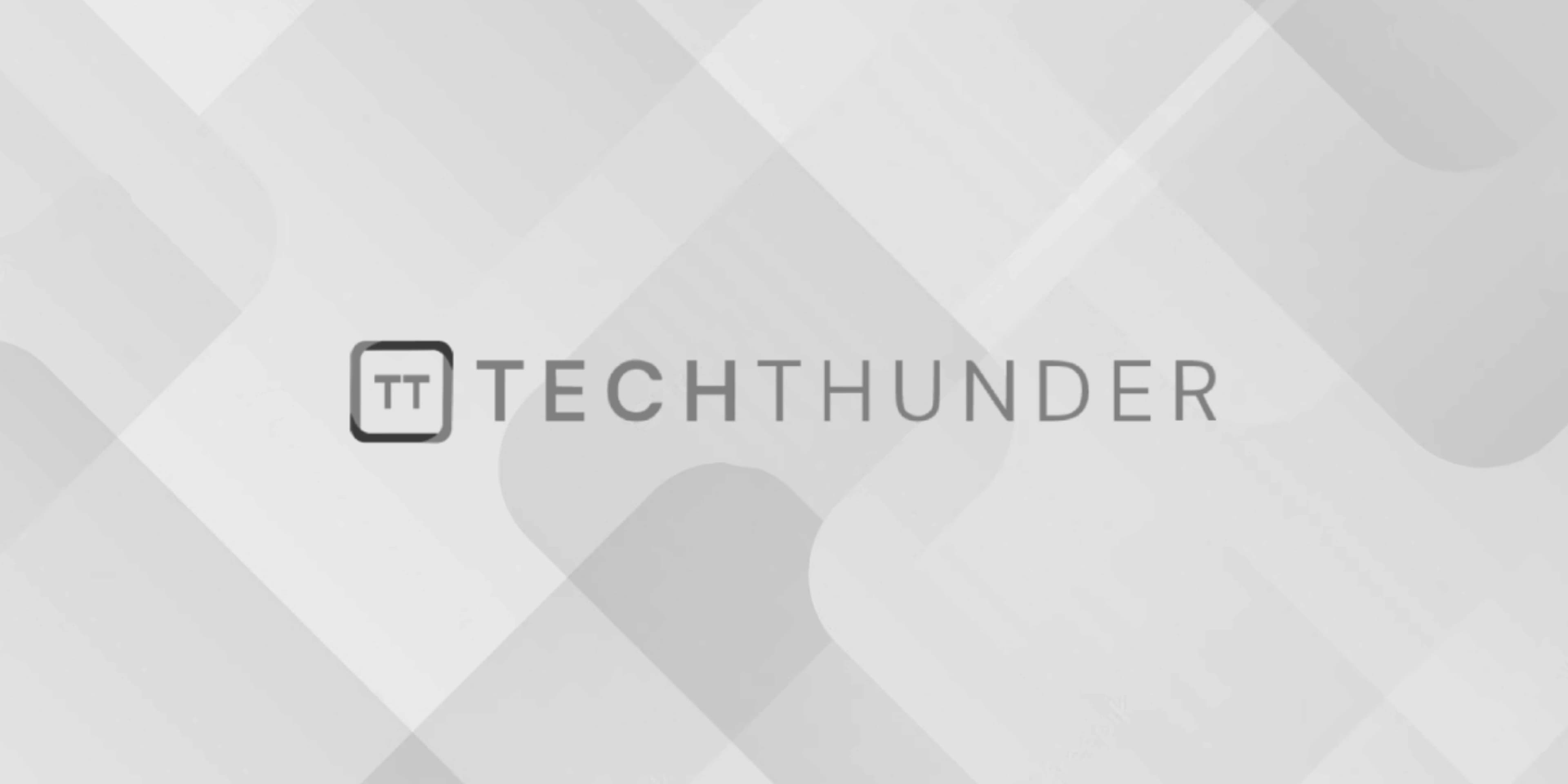
370 views
Hospital Management System in C
Creating a complete hospital management system in C is a complex task that typically involves multiple modules, database management, user interfaces, and more. Here, I’ll provide you with a simplified example of a hospital management system in C that focuses on managing patient records using file operations. This example uses basic data structures and is for educational purposes.
C
#include <stdio.h>
#include <stdlib.h>
// Structure to represent patient information
struct Patient {
int id;
char name[50];
int age;
};
// Function to add a new patient record to the file
void addPatientRecord(FILE *file) {
struct Patient patient;
printf("Enter patient ID: ");
scanf("%d", &patient.id);
printf("Enter patient name: ");
scanf("%s", patient.name);
printf("Enter patient age: ");
scanf("%d", &patient.age);
// Write the patient record to the file
fwrite(&patient, sizeof(struct Patient), 1, file);
printf("Patient record added successfully.\n");
}
// Function to display all patient records from the file
void displayPatientRecords(FILE *file) {
struct Patient patient;
// Read and display each patient record from the file
rewind(file); // Move the file pointer to the beginning
printf("Patient Records:\n");
while (fread(&patient, sizeof(struct Patient), 1, file) == 1) {
printf("ID: %d, Name: %s, Age: %d\n", patient.id, patient.name, patient.age);
}
}
int main() {
FILE *file;
file = fopen("patient_records.dat", "ab+");
if (file == NULL) {
perror("Error opening the file");
return 1;
}
int choice;
do {
printf("\nHospital Management System\n");
printf("1. Add Patient Record\n");
printf("2. Display Patient Records\n");
printf("3. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
addPatientRecord(file);
break;
case 2:
displayPatientRecords(file);
break;
case 3:
printf("Exiting the program.\n");
break;
default:
printf("Invalid choice. Please try again.\n");
}
} while (choice != 3);
fclose(file);
return 0;
}
In this simplified hospital management system:
- Patient records are stored in a binary file named “patient_records.dat.”
- You can add patient records by providing the ID, name, and age of the patient.
- You can display all patient records stored in the file.
- The program menu allows you to choose between these two options or exit the program.
Please note that this is a very basic example, and a real hospital management system would involve much more complexity, including data validation, multiple data structures, database management, user authentication, and more.