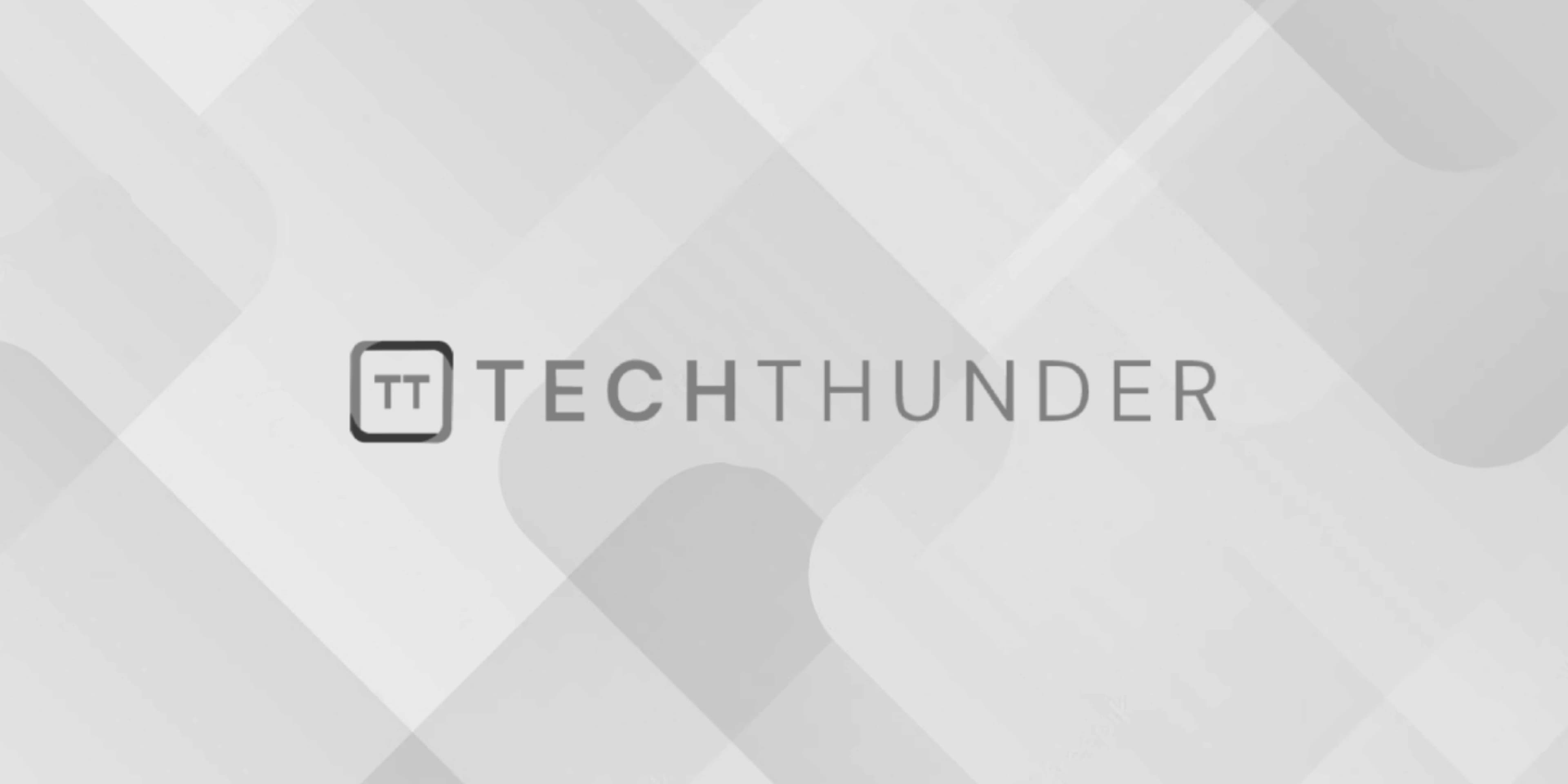
typedef vs define in C
The typedef
and #define
are both used in C to create aliases or synonyms for data types, but they serve different purposes and have different mechanisms.
typedef:
typedef
is used to define a new data type or create a synonym for an existing data type. It improves code readability and allows you to create more descriptive type names. It is often used with user-defined data structures like structures and unions to simplify their use.
typedef int myInt; // Creating a synonym for int
myInt x = 42; // Now you can use myInt as an alias for int
In this example, myInt
is defined as an alias for the int
data type, so you can use myInt
to declare variables of type int
.
#define:
#define
is a preprocessor directive used to create macros or symbolic constants. It is a text replacement mechanism, where the preprocessor replaces occurrences of the macro with the specified value before the code is compiled. #define
does not create new data types but can be used for creating constants or for simple text substitution.
#define PI 3.14159265359
double circleArea = PI * radius * radius; // Using the PI constant
In this example, PI
is defined as a macro representing the value 3.14159265359. It is a text substitution mechanism that replaces PI
with its value in the code.
Key Differences:
- Type Safety:
typedef
creates type-safe aliases because it defines new data types based on existing types.#define
does not provide type safety as it performs textual substitution without type checking. - Scoping:
typedef
is scoped and follows the rules of scope just like regular type names.#define
macros are global and not scoped. - Debugging:
typedef
allows for better debugging because the compiler recognizes the new type names, making it easier to understand error messages. Debugging with#define
macros can be more challenging because they are purely text substitutions.
In summary, use typedef
when you want to create new type names or type synonyms for improved code readability and type safety. Use #define
for creating constants or simple text substitutions, but be cautious about potential issues related to type safety and scoping.