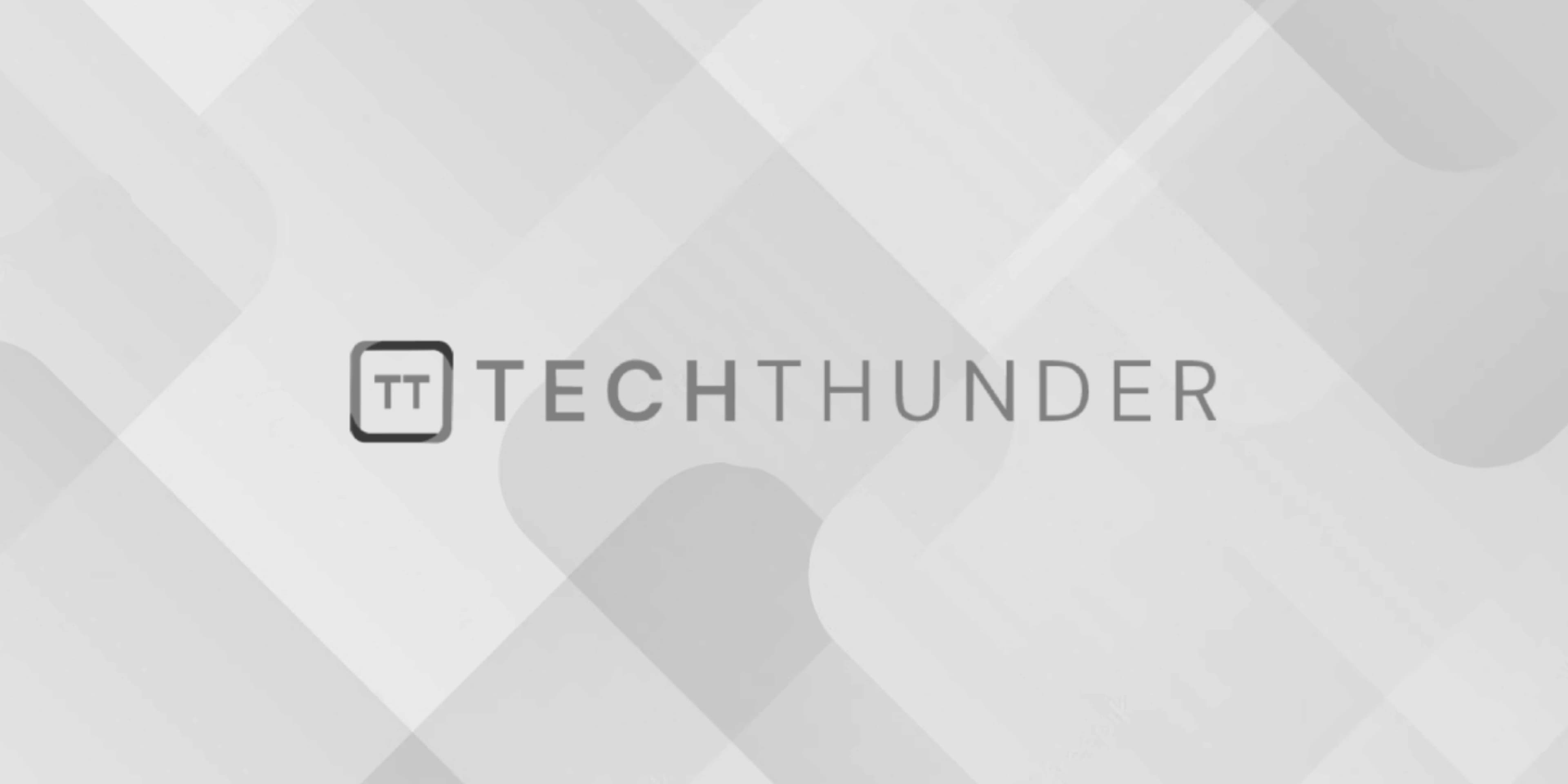
C ftell()
The ftell()
function in C is used to determine the current file position indicator for a given file stream. It is typically used with files that have been opened using the fopen()
function.
Here is the syntax of ftell()
:
long ftell(FILE *stream);
stream
: A pointer to a FILE
object representing the file for which you want to get the current position.
The ftell()
function returns the current file position indicator as a long integer representing the byte offset from the beginning of the file. If an error occurs, it returns -1L.
Here’s an example of how to use ftell()
:
#include <stdio.h>
int main() {
FILE *file;
file = fopen("example.txt", "r");
if (file == NULL) {
perror("Error opening file");
return 1;
}
// Use ftell to get the current position in the file
long position = ftell(file);
if (position == -1L) {
perror("Error getting file position");
} else {
printf("Current position in the file: %ld bytes\n", position);
}
fclose(file);
return 0;
}
In this example, we open a file called “example.txt” in read mode, use ftell()
to get the current file position, and then print it. Remember to include error handling to deal with cases where the file cannot be opened or the file position cannot be determined.