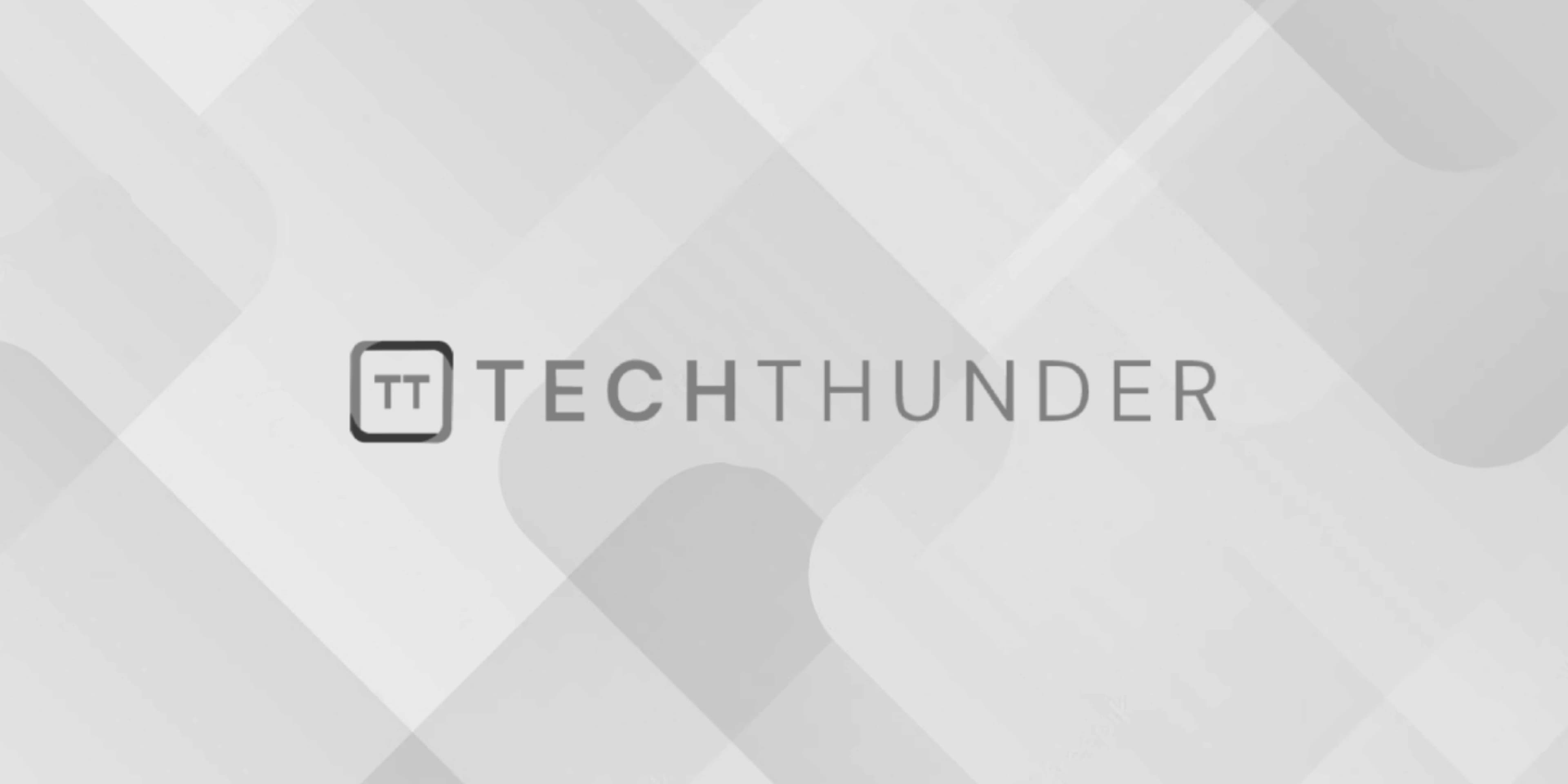
205 views
LCM of two numbers in C
To calculate the least common multiple (LCM) of two numbers in C, you can use the following formula:
C
LCM(a, b) = |a * b| / GCD(a, b)
Where LCM(a, b)
is the LCM of numbers a
and b
, and GCD(a, b)
is their greatest common divisor.
You can calculate the LCM of two numbers in C using a function that calculates the GCD first and then applies the formula. Here’s a C program to find the LCM of two numbers:
C
#include <stdio.h>
// Function to calculate the GCD (Greatest Common Divisor) of two numbers
int gcd(int a, int b) {
while (b != 0) {
int temp = b;
b = a % b;
a = temp;
}
return a;
}
// Function to calculate the LCM (Least Common Multiple) of two numbers
int lcm(int a, int b) {
return (a * b) / gcd(a, b);
}
int main() {
int num1, num2;
printf("Enter two numbers: ");
scanf("%d %d", &num1, &num2);
int result = lcm(num1, num2);
printf("LCM of %d and %d is %d\n", num1, num2, result);
return 0;
}
In this program:
- We have two functions:
gcd
(to calculate the greatest common divisor) andlcm
(to calculate the least common multiple). - The
gcd
function calculates the GCD using the Euclidean algorithm, which we discussed earlier. - The
lcm
function uses the GCD to calculate the LCM using the formulaLCM(a, b) = |a * b| / GCD(a, b)
. - In the
main
function, we input two numbers from the user and then call thelcm
function to calculate the LCM.
Compile and run the program, and it will calculate and display the LCM of the two numbers you provide as input.