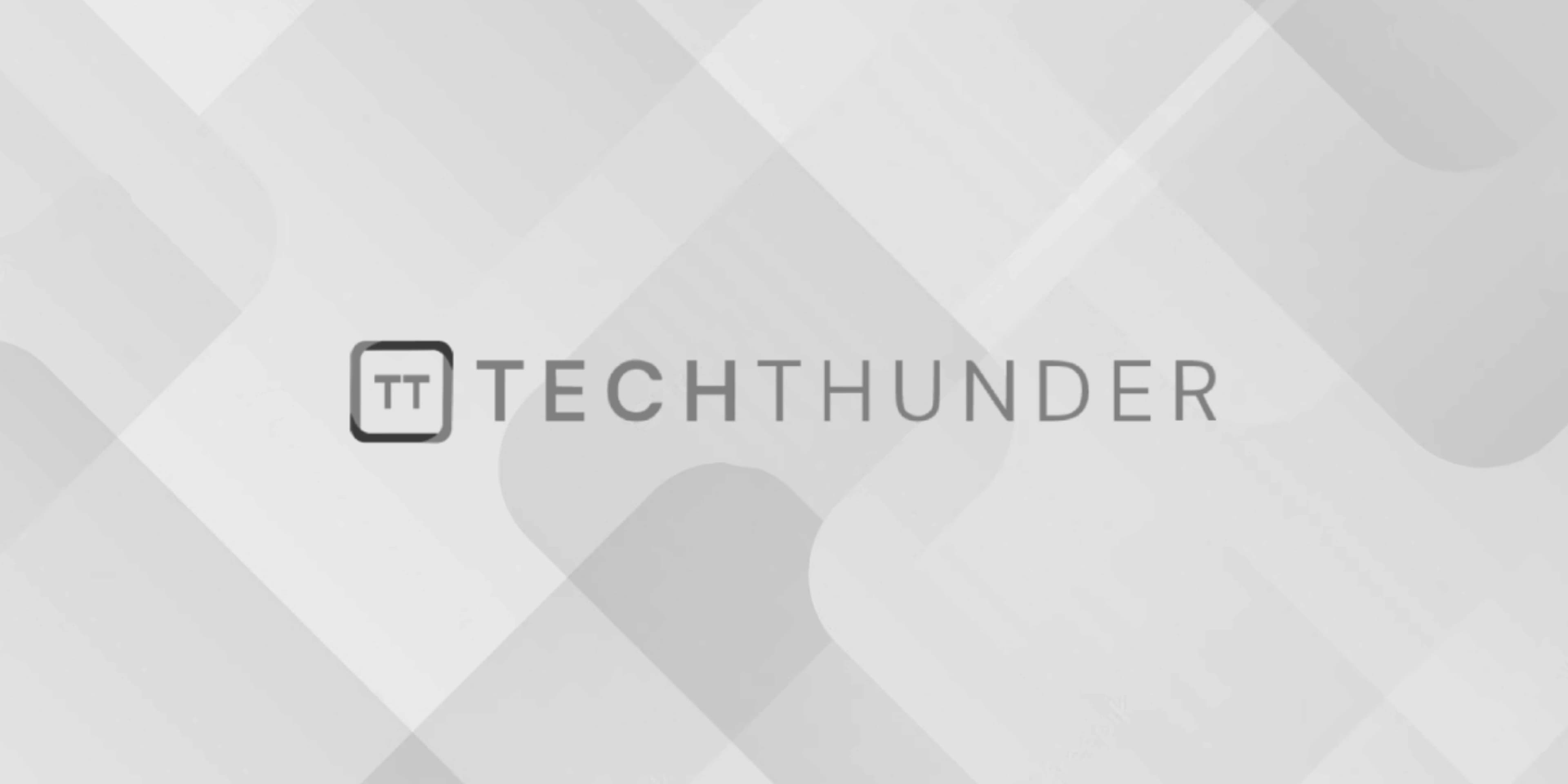
210 views
Compilation Process in C
The compilation process in C involves translating human-readable C source code into machine-executable code that a computer can understand and execute. This process typically consists of several stages, which are:
- Preprocessing:
- In the first stage, the C preprocessor is invoked.
- The preprocessor processes the source code before actual compilation.
- It handles preprocessor directives (lines starting with
#
) and performs tasks like including header files, macro expansion, and conditional compilation. - The output of this stage is often referred to as the “preprocessed code.”
- Compilation:
- In this stage, the preprocessed code is passed to the C compiler (e.g., GCC).
- The compiler generates assembly code or an intermediate representation of the program.
- The generated code is not yet in machine language but is closer to it than the original C source code.
- Syntax and semantic errors are checked at this stage, and if any errors are found, the compiler generates error messages.
- Assembly (Optional):
- Some compilers generate assembly code as an intermediate step, which can be useful for debugging or analyzing the generated code.
- This assembly code can be examined to understand how the C code is translated into lower-level instructions.
- Linking:
- The linking stage takes place if the program consists of multiple source files or if external libraries are used.
- The linker (e.g., GNU
ld
) combines the compiled code and resolves external references to create a single executable program. - External references include function calls to library functions or other functions defined in separate source files.
- Output:
- The final output of the compilation process is an executable binary file (e.g., an executable program or shared library) with machine code instructions.
- This binary file can be run on the target platform.
Here’s a simplified overview of the steps:
- C Source Code
- Write your C source code in a text editor.
- Preprocessing
- Preprocess the code with the C preprocessor (e.g.,
gcc -E
). - Handle
#include
directives, macro expansions, and conditionals.
- Compilation
- Compile the preprocessed code with the C compiler (e.g.,
gcc -c
). - Generate intermediate code and perform syntax and semantic checks.
- Assembly (Optional)
- Generate assembly code (e.g.,
gcc -S
) if desired.
- Linking
- Link object files and libraries to create an executable program (e.g.,
gcc
without-c
). - Resolve external references and create the final executable.
- Executable Program
- The compiled and linked program is now ready to be executed.
It’s important to note that different compilers and platforms may have variations in the compilation process, but the general stages mentioned above remain consistent. Understanding the compilation process is essential for troubleshooting errors and optimizing your C programs.