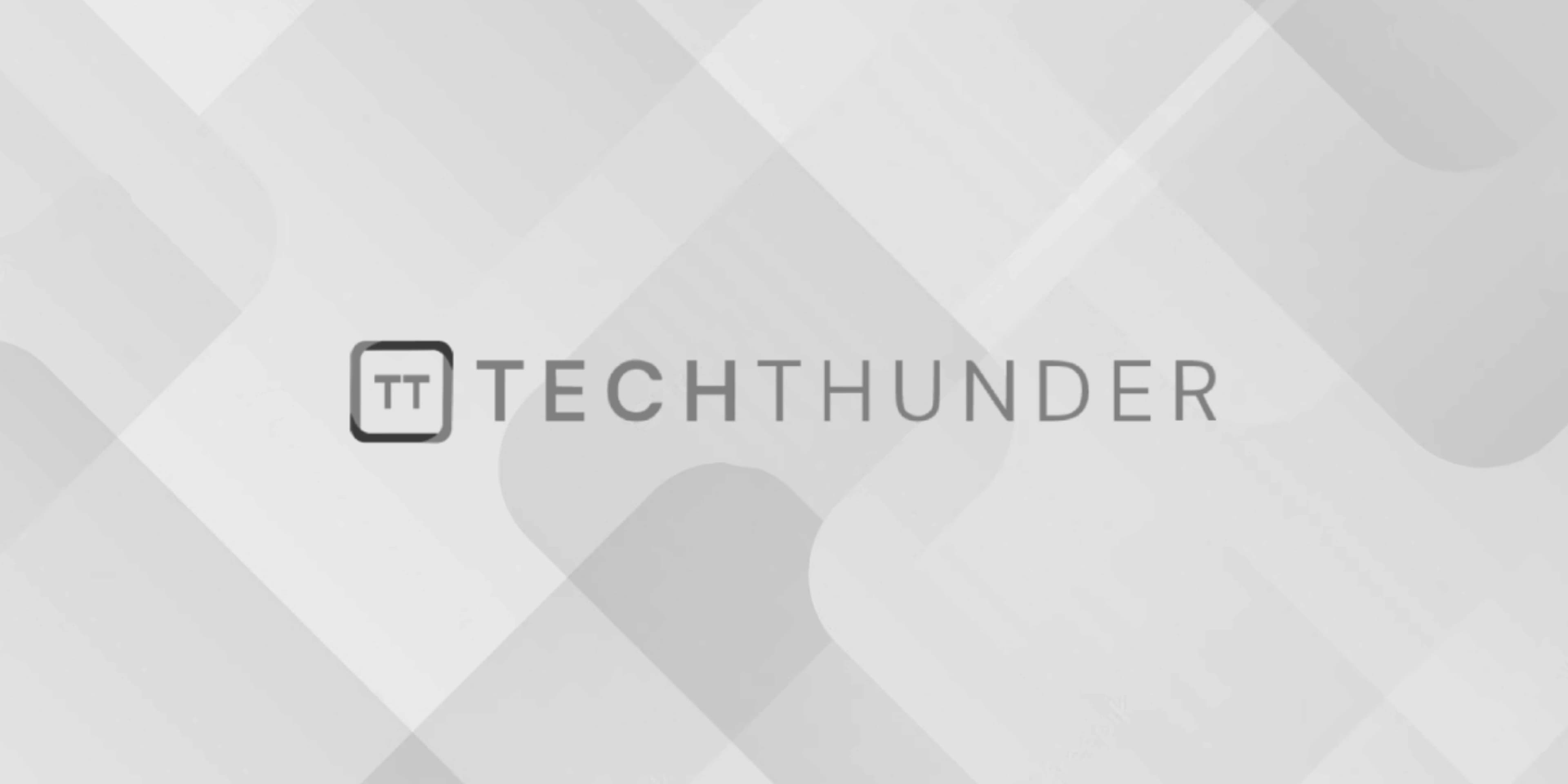
124 views
Atoi() function in C
The atoi()
function is used to convert a string (character array) representing an integer into an actual integer value. The name atoi
stands for “ASCII to Integer.” It’s part of the C Standard Library and is declared in the stdlib.h
header file. Here’s the basic usage of the atoi()
function:
C
#include <stdio.h>
#include <stdlib.h>
int main() {
const char *str = "12345"; // A string representing an integer
int number = atoi(str); // Convert the string to an integer
printf("The integer value is: %d\n", number);
return 0;
}
In this example, we have a string "12345"
, and we use atoi()
to convert it into an integer value, which is then stored in the variable number
. The result is that number
now contains the integer 12345
.
It’s important to note a few things about atoi()
:
- Error Handling:
atoi()
does not provide error checking. If the input string is not a valid integer, it will return0
. Therefore, it’s essential to validate the input string to ensure it represents a valid integer before usingatoi()
. - Leading Whitespace:
atoi()
will skip leading whitespace characters in the input string until it encounters a digit or a plus/minus sign. For example," 123"
will be converted to123
. - Trailing Characters: If the input string contains characters after the integer value (e.g.,
"123abc"
),atoi()
will stop parsing at the first non-numeric character, so it will return123
. - Overflow and Underflow:
atoi()
does not perform any checks for integer overflow or underflow. If the input string represents a value that is too large or too small to fit into anint
, the behavior is undefined. To handle potential overflow or underflow, you might consider usingstrtol()
or similar functions, which provide more control and error handling.
Here’s an example demonstrating error checking with atoi()
:
C
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
bool isInteger(const char *str) {
// Attempt to convert the string to an integer
int number = atoi(str);
// If the conversion resulted in 0 and the first character is not '0',
// it's not a valid integer.
if (number == 0 && str[0] != '0') {
return false;
}
return true;
}
int main() {
const char *str1 = "12345"; // Valid integer
const char *str2 = "12abc"; // Not a valid integer
if (isInteger(str1)) {
printf("%s is a valid integer.\n", str1);
} else {
printf("%s is not a valid integer.\n", str1);
}
if (isInteger(str2)) {
printf("%s is a valid integer.\n", str2);
} else {
printf("%s is not a valid integer.\n", str2);
}
return 0;
}
In this updated example, we define an isInteger
function that checks whether a given string can be converted to a valid integer using atoi()
.