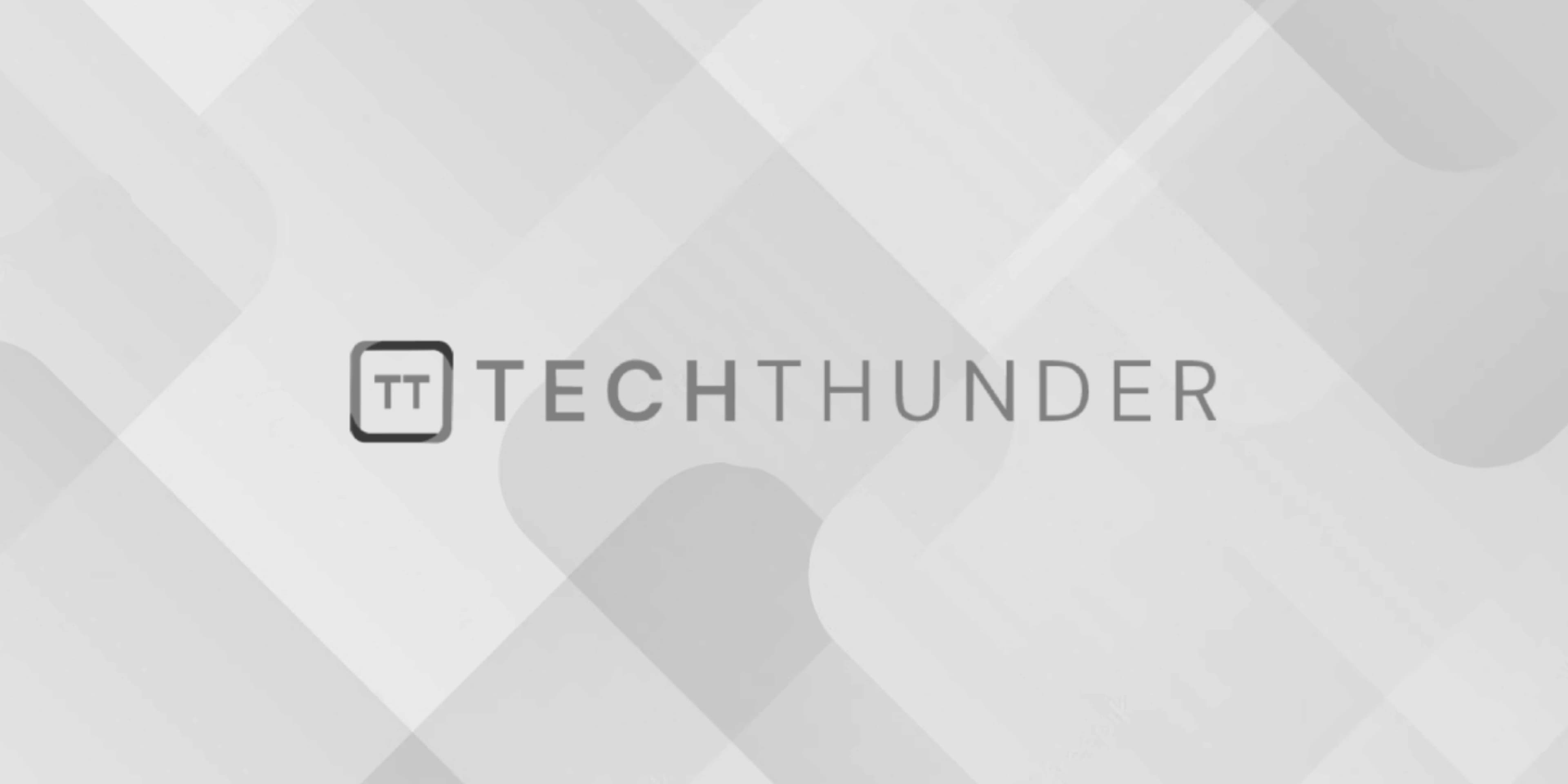
C Array of Structures
You can create an array of structures to store multiple instances of a structured data type in a contiguous block of memory. This is useful when you need to work with collections of related data that share a common structure. To create an array of structures, you define a structure type and then declare an array of that type.
Here’s how you can create an array of structures in C:
#include <stdio.h>
// Define the structure
struct Student {
char name[50];
int roll_number;
float marks;
};
int main() {
// Declare an array of structures
struct Student students[3];
// Initialize the array elements
strcpy(students[0].name, "Alice");
students[0].roll_number = 101;
students[0].marks = 95.5;
strcpy(students[1].name, "Bob");
students[1].roll_number = 102;
students[1].marks = 88.0;
strcpy(students[2].name, "Charlie");
students[2].roll_number = 103;
students[2].marks = 91.2;
// Access and print the array elements
for (int i = 0; i < 3; i++) {
printf("Student %d:\n", i + 1);
printf("Name: %s\n", students[i].name);
printf("Roll Number: %d\n", students[i].roll_number);
printf("Marks: %.2f\n", students[i].marks);
printf("\n");
}
return 0;
}
In this example, we define a struct Student
to represent information about students. We then declare an array of struct Student
named students
with a size of 3. We initialize the elements of the array with data for three different students using array indexing.
You can access and manipulate the individual elements of the array just like you would with a regular structure variable. In the example, we use a loop to print the information of each student in the array.
Arrays of structures are commonly used to store and process data that naturally fits into a structured format, such as employee records, product information, or any other dataset with multiple attributes for each item.