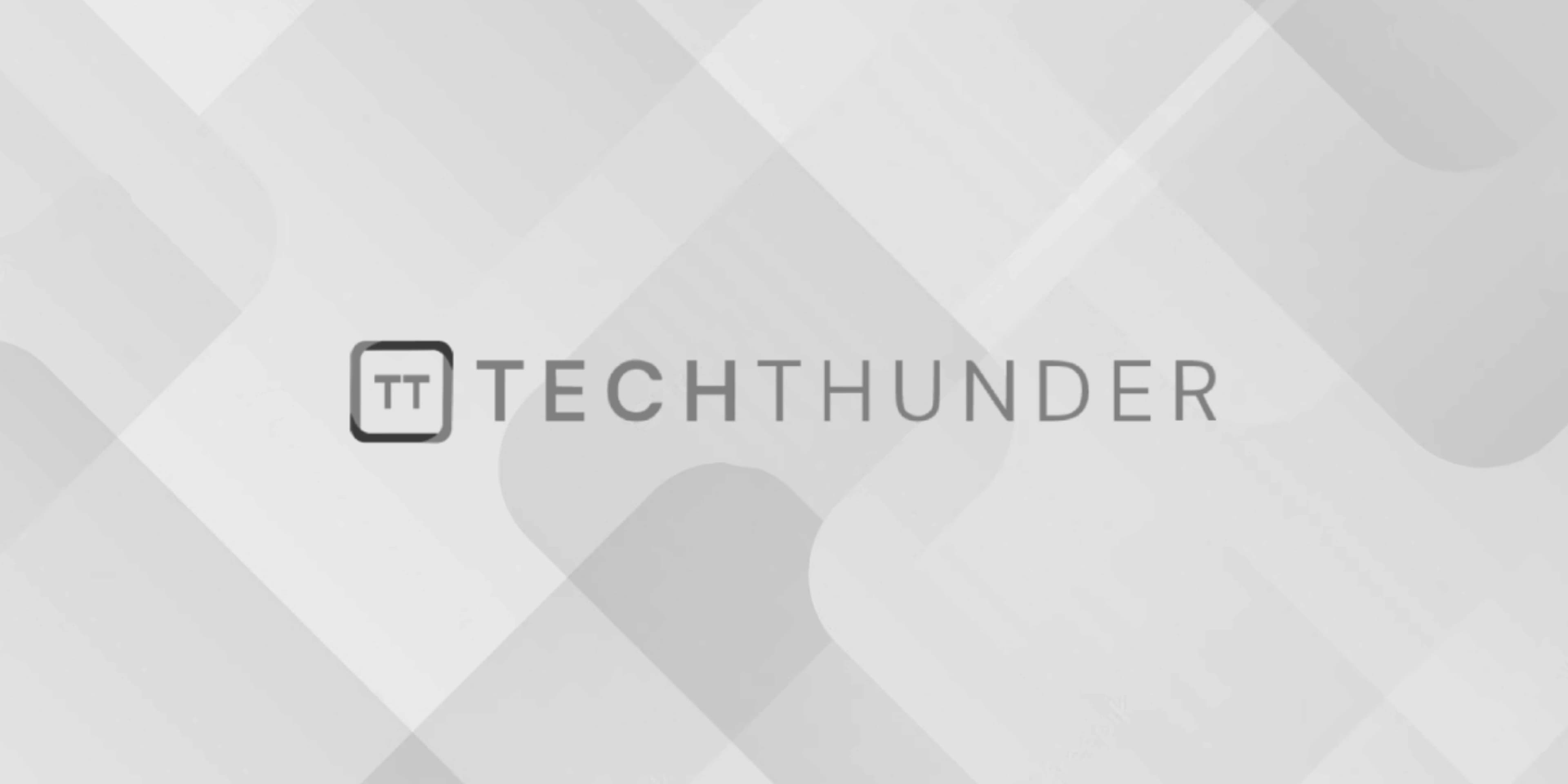
Abort() Function in C
The abort()
function is used to terminate the program abnormally and initiate the abnormal program termination process. It is part of the C Standard Library and is typically used in cases where a critical error or unrecoverable condition is encountered. The abort()
function is declared in the stdlib.h
header.
Here’s the prototype of the abort()
function:
#include <stdlib.h>
void abort(void);
abort()
takes no arguments.
When the abort()
function is called:
- It generates a termination signal, often referred to as SIGABRT (Abort), which is a signal sent to the program.
- The program is terminated immediately.
- An optional message may be printed to the standard error stream (stderr) before termination, depending on the platform and implementation.
Here’s a simple example of how to use the abort()
function:
#include <stdio.h>
#include <stdlib.h>
int main() {
int divisor = 0;
printf("Enter a number to divide 10 by: ");
scanf("%d", &divisor);
if (divisor == 0) {
fprintf(stderr, "Error: Division by zero is not allowed.\n");
abort(); // Terminate the program
}
int result = 10 / divisor;
printf("Result: %d\n", result);
return 0;
}
In this example, if the user enters 0
as the divisor, the program checks for this condition and, if true, calls abort()
. This will cause the program to terminate abruptly with an error message.
abort()
is typically used in situations where the program encounters unrecoverable errors, and you want to exit the program cleanly while providing an indication of the error. It is commonly used in conjunction with error handling mechanisms to gracefully handle exceptional cases.