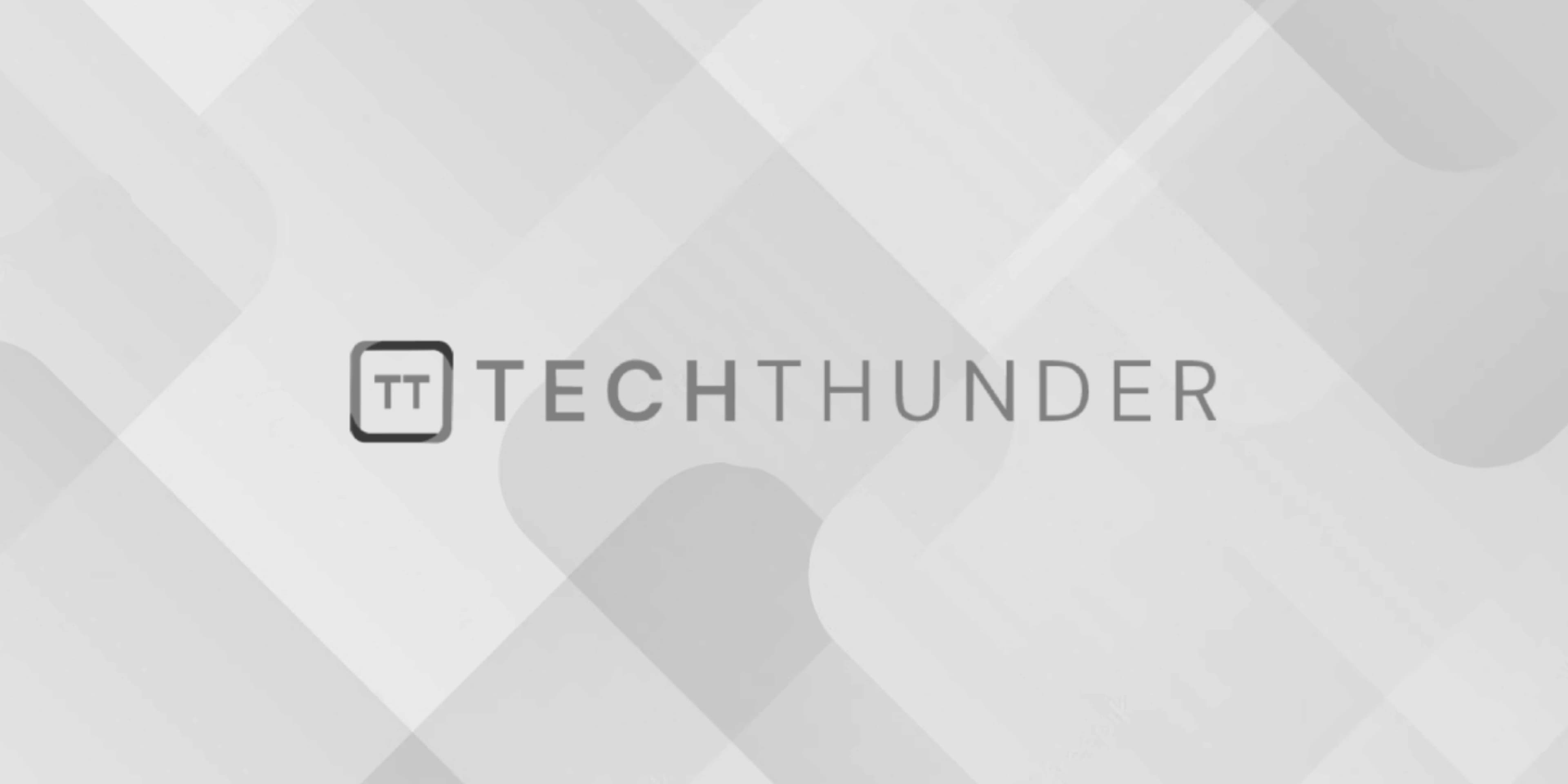
157 views
Unary Operator in C
The unary operators are operators that work with a single operand, or in other words, they operate on a single value or variable. C supports several unary operators, each with its own specific functionality. Here are some common unary operators in C:
- Unary Plus (
+
): It simply returns the operand’s value with a positive sign. This operator is rarely used, as it doesn’t change the value of the operand. Example:
C
int x = 5;
int result = +x; // result will be 5
- Unary Minus (
-
): It negates the value of the operand. If the operand is positive, it becomes negative, and if it’s negative, it becomes positive. Example:
C
int x = 5;
int result = -x; // result will be -5
- Increment (
++
): It increases the value of the operand by 1. It can be used as a prefix (++x
) or a postfix (x++
) operator. Example:
C
int x = 5;
int result = ++x; // result will be 6, and x becomes 6
- Decrement (
--
): It decreases the value of the operand by 1. Like the increment operator, it can be used as a prefix (--x
) or a postfix (x--
) operator. Example:
C
int x = 5;
int result = x--; // result will be 5, but x becomes 4
- Logical NOT (
!
): It reverses the logical value of the operand. If the operand is true (non-zero), it becomes false (0), and if it’s false (0), it becomes true (non-zero). Example:
C
int isTrue = 1;
int isFalse = !isTrue; // isFalse will be 0
- Bitwise NOT (
~
): It performs bitwise negation on each bit of the operand, changing 0s to 1s and vice versa. Example:
C
unsigned int x = 5;
// Binary: 0000 0000 0000 0000 0000 0000 0000 0101
unsigned int result = ~x;
// Binary: 1111 1111 1111 1111 1111 1111 1111 1010, result will be a large positive integer
- Address-of (
&
): It returns the memory address of the operand (variable). It is often used with pointers to store the address of a variable. Example:
C
int x = 5;
int *ptr = &x; // ptr now contains the address of x
- Indirection (Dereference) (
*
): It is used with pointers to access the value stored at the memory address pointed to by the operand. Example:
C
int x = 5;
int *ptr = &x;
int value = *ptr;
// value will be 5 (the value stored at the memory address pointed to by ptr)
Unary operators are essential in C and are used for a variety of tasks, including arithmetic operations, logical operations, and memory manipulation.